使用 Xlwings 在Python中处理 Excel 文件
Xlwings是一个Python库,可以轻松地从 Excel 调用Python ,反之亦然。它使用Python轻松创建 Excel 的读写。还可以修改它作为 Excel 的Python服务器,在Python和 Excel 之间同步交换数据。 Xlwings使使用Python自动化 Excel 变得容易,可用于生成自动报告、创建 Excel 嵌入函数、操作 Excel 或 CSV 数据库等。
安装:
虚拟环境用于将项目环境(库、环境变量等)等与其他项目以及同一台机器的全局环境分开。此步骤是可选的,因为并非总是需要为项目创建虚拟环境。为此,我们将使用Python包virtualenv 。
virtualenv env
.\env\scripts\activate
虚拟环境已准备就绪。
pip install xlwings
要开始使用Xlwings ,几乎每次都需要完成一些基本步骤。这包括打开 Excel 文件,查看可用的工作表,然后选择一个工作表。
data.xlsx 文件的第 1 页。
使用的Excel:点击这里
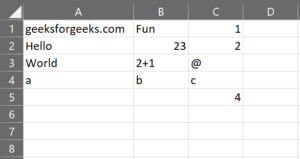
https://drive.google.com/file/d/1ZoT_y-SccAslpD6HWTgCn9N-iiKqw_pA/view?usp=sharing
下面是一些描述如何使用 Xlwings 库执行各种操作的示例:
示例 1:
Python3
# Python program to
# access Excel files
# Import required library
import xlwings as xw
# Opening an excel file
wb = xw.Book('data.xlsx')
# Viewing available
# sheets in it
wks = xw.sheets
print("Available sheets :\n", wks)
# Selecting a sheet
ws = wks[0]
# Selecting a value
# from the selected sheet
val = ws.range("A1").value
print("A value in sheet1 :", val)
Python3
# Python3 code to select
# data from excel
import xlwings as xw
# Specifying a sheet
ws = xw.Book("data.xlsx").sheets['Sheet1']
# Selecting data from
# a single cell
v1 = ws.range("A2").value
v2 = ws.range("A3").value
print("Result :", v1, v2)
# Selecting entire
# rows and columns
r = ws.range("4:4").value
print("Row :", r)
c = ws.range("C:C").value
print("Column :", c)
# Selecting a 2D
# range of data
table = ws.range("A1:C4").value
print("Table :", table)
# Automatic table
# detection from
# a cell
automatic = ws.range("A1").expand().value
print("Automatic Table :", automatic)
Python3
# Python3 code to write
# data to Excel
import xlwings as xw
# Selecting a sheet in Excel
ws = xw.Book('data.xlsx').sheets("Sheet2")
# Writing one value to
# one cell
ws.range("A1").value = "geeks"
# Writing multiple values
# to a cell for automatic
# data placement
ws.range("B1").value = ["for", "geeks"]
# Writing 2D data to a cell
# to automatically put data
# into correct cells
ws.range("A2").value = [[1, 2, 3], ['a', 'b', 'c']]
# Writing multiple data to
# multiple cells
ws.range("A4:C4").value = ["This", "is", "awesome"]
# Outputting entire table
print("Table :\n", ws.range("A1").expand().value)
输出:
通过指定单元格、行、列或区域,可以从 Excel 工作表中选择值。不建议选择整行或整列,因为 Excel 中的整行或整列很长,因此会导致一长串尾随 None。选择一系列二维数据将产生一个数据列表列表。
示例 2:
蟒蛇3
# Python3 code to select
# data from excel
import xlwings as xw
# Specifying a sheet
ws = xw.Book("data.xlsx").sheets['Sheet1']
# Selecting data from
# a single cell
v1 = ws.range("A2").value
v2 = ws.range("A3").value
print("Result :", v1, v2)
# Selecting entire
# rows and columns
r = ws.range("4:4").value
print("Row :", r)
c = ws.range("C:C").value
print("Column :", c)
# Selecting a 2D
# range of data
table = ws.range("A1:C4").value
print("Table :", table)
# Automatic table
# detection from
# a cell
automatic = ws.range("A1").expand().value
print("Automatic Table :", automatic)
输出:
Xlwings可用于在 Excel 文件中插入数据,类似于从 Excel 文件中读取数据。数据可以作为列表或单个输入提供给某个单元格或单元格的选择。
示例 3:
蟒蛇3
# Python3 code to write
# data to Excel
import xlwings as xw
# Selecting a sheet in Excel
ws = xw.Book('data.xlsx').sheets("Sheet2")
# Writing one value to
# one cell
ws.range("A1").value = "geeks"
# Writing multiple values
# to a cell for automatic
# data placement
ws.range("B1").value = ["for", "geeks"]
# Writing 2D data to a cell
# to automatically put data
# into correct cells
ws.range("A2").value = [[1, 2, 3], ['a', 'b', 'c']]
# Writing multiple data to
# multiple cells
ws.range("A4:C4").value = ["This", "is", "awesome"]
# Outputting entire table
print("Table :\n", ws.range("A1").expand().value)
输出:
Excel截图: