将函数应用于 R 数据帧中的每个值
在 R 编程语言中,要将函数应用于数据帧中的每个整数类型值,我们可以使用dplyr包中的lapply函数。如果值的数据类型是字符串,那么我们可以将paste()与lapply一起使用。让我们借助一个例子来理解这个问题。
使用中的数据集: A B C D 1. 1 8 21 4 2. 9 2 0 6 3. 6 3 14 3 4. 5 6 5 7 5. 9 4 3 1 6. 6 3 2 3
将 value*7+1 应用于数据帧的每个值后
预期结果: A B C D 1. 8 57 148 29 2. 64 15 1 43 3. 43 22 99 22 4. 36 43 36 50 5. 64 29 22 8 6. 43 22 15 22
方法 1:使用 lapply函数:
lapply是apply 系列的一个函数。通过使用 lapply,我们可以避免 for 循环,因为 for 循环比 lapply 慢。 lapply 比普通循环运行得更快,因为它不会干扰您工作的环境。它以列表形式返回输出。 lapply 中的“l”表示列表。
句法:
lapply(X, FUN, …)
这里,X 可以是向量列表或数据框。而有趣的需要,你要应用到数据帧作为自变量的函数。
方法:
- 创建一个虚拟数据集。
- 创建要应用于数据框中每个值的自定义函数。
- 在 lapply 的帮助下,将此自定义函数应用于数据框中的每个值。
- 显示结果
例子
R
# Apply function to every value in dataframe
# Creating dataset
m <- c(1,9,6,5,9,6)
n <- c(8,2,3,6,4,3)
o <- c(21,0,14,5,3,2)
p <- c(4,6,3,7,1,3)
# creating dataframe
df <- data.frame(A=m,B=n,C=o,D=p)
# creating function
# that will multiply
# each value by 7 and then add 1
magic_fun <- function(x){
return (x*7+1)}
# applying the custom function to every value and converting
# it to dataframe, as lapply returns result in list
# we have to convert it to data frame
data.frame(lapply(df,magic_fun))
R
# Apply function to every value in dataframe
# Creating dataset
m <- c("Vikas","Varun","Deepak")
n <- c("Komal","Suneha","Priya")
# creating dataframe
df <- data.frame(A=m,B=n)
# Applying custom function to every element in dataframe
df[]<-data.frame(lapply(df,function(x) paste("Hello,",x,sep="")))
# display dataset
df
R
# Apply function to every value in dataframe
# install and load the purrr
install.packages("purrr")
library("purrr")
# Creating a vector
x <- c(1,2,3,4,5,7,8,9)
# creating list
y <- list(2,4,9,6,3,7,1,5,4)
# creating dataframe
df <- data.frame(A=c(1,2,3,4,5),B=c(6,7,8,9,10))
# creating custom function
custom_f <- function(x){
return(x*2)
}
# applying function to vector
# and return output as vector
map_dbl(x,.f=custom_f)
# applying function to list
# and return output as vector
map(y,.f=custom_f)
# applying function to dataframe
# and return output as dataframe
map_df(df,.f=custom_f)
R
# Apply function to every value in dataframe
#install and load the purrr
#install.packages("purrr")
library("purrr")
# Creating a vector
x <- c("Red","Blue", "Green","Yellow","Orange")
# creating list
y <- list("spring","summer", "fall","winter")
# creating dataframe
df <- data.frame(Working_days=c("Mon","Tues","Wednes"),
off_days=c("Sun","Satur","Thurs") )
# applying function to vector
# and return output as vector
map_chr(x,paste0," color")
# applying function to list
# and return output as vector
map(y,paste0," season")
# applying function to dataframe
# and return output as dataframe
map_df(df,paste0,"day")
输出 :
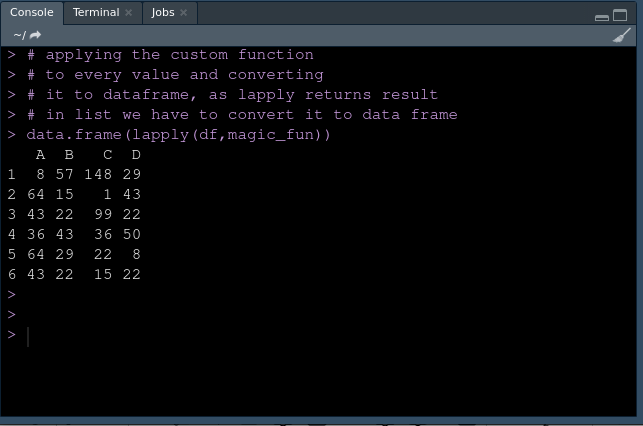
使用 lapply
方法 2:使用粘贴和应用函数:
粘贴()采用的R对象作为参数并将其转换为字符然后将其粘贴背面与另一字符串,ieit将参数转换成和连接它们。
句法:
paste (…, sep = ” “)
我们的研发对象将被转换成字符串去代替“...”,月=”“表示的以分离的条款。
方法:
- 创建一个虚拟数据集。
- 应用将打印“Hello”的自定义函数,然后在数据框值中取值
- 显示结果
例子:
电阻
# Apply function to every value in dataframe
# Creating dataset
m <- c("Vikas","Varun","Deepak")
n <- c("Komal","Suneha","Priya")
# creating dataframe
df <- data.frame(A=m,B=n)
# Applying custom function to every element in dataframe
df[]<-data.frame(lapply(df,function(x) paste("Hello,",x,sep="")))
# display dataset
df
输出:
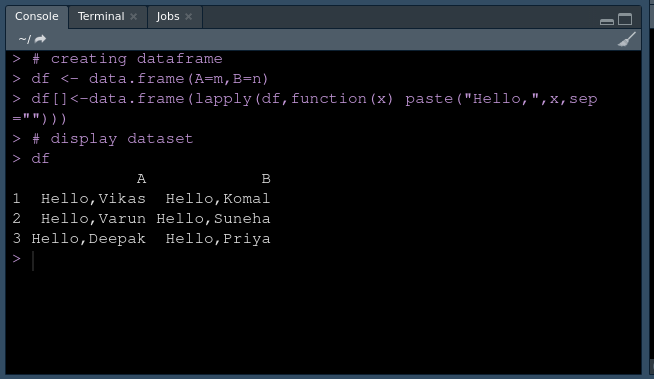
使用粘贴和应用
方法 3:使用 purrr
purrr是一个函数式编程工具包。它带有许多有用的功能,例如地图。 map()函数遍历向量的所有条目并将输出作为列表返回。它允许我们在代码中替换 for 循环并使其更易于阅读。
句法 :
map(.x, .f) returns a list
map_df(.x, .f) returns a data frame
map_dbl(.x, .f) returns a numeric (double) vector
map_chr(.x, .f) returns a character vector
map_lgl(.x, .f) returns a logical vector
这里, .x是输入, .f是您要应用的函数。 map函数的输入可以是列表、向量或数据框。
注意:您需要使用以下命令显式安装purrr包。
install.packages(“purrr”)
方法 :
- 创建一个向量、一个列表和一个数据框
- 创建要应用的自定义函数。
- 使用 map() 在向量、列表和数据框上应用自定义函数。
- 显示结果
使用整数数据类型:
程序:
电阻
# Apply function to every value in dataframe
# install and load the purrr
install.packages("purrr")
library("purrr")
# Creating a vector
x <- c(1,2,3,4,5,7,8,9)
# creating list
y <- list(2,4,9,6,3,7,1,5,4)
# creating dataframe
df <- data.frame(A=c(1,2,3,4,5),B=c(6,7,8,9,10))
# creating custom function
custom_f <- function(x){
return(x*2)
}
# applying function to vector
# and return output as vector
map_dbl(x,.f=custom_f)
# applying function to list
# and return output as vector
map(y,.f=custom_f)
# applying function to dataframe
# and return output as dataframe
map_df(df,.f=custom_f)
输出:
对于向量:
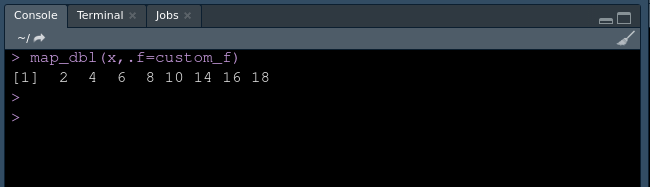
矢量输出
对于列表:
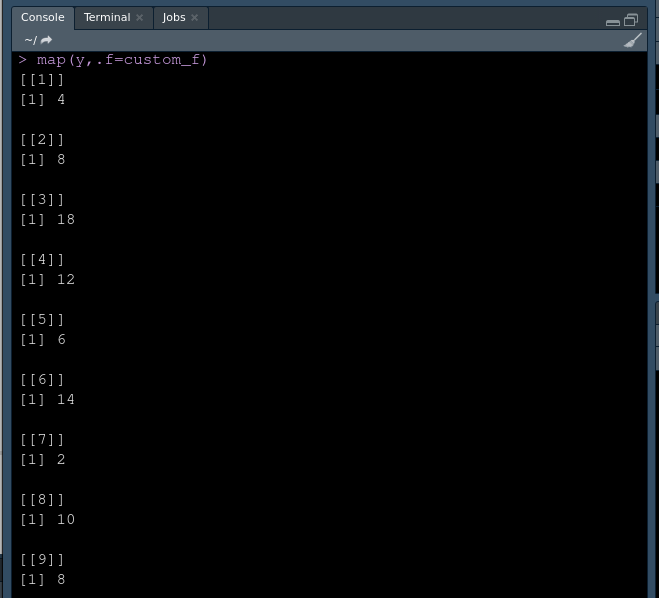
列出输出
对于数据框:
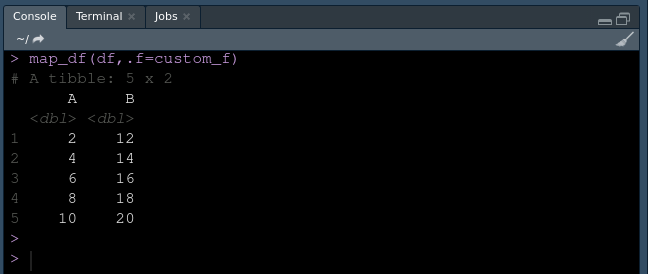
数据帧输出
使用字符数据类型:
程序:
电阻
# Apply function to every value in dataframe
#install and load the purrr
#install.packages("purrr")
library("purrr")
# Creating a vector
x <- c("Red","Blue", "Green","Yellow","Orange")
# creating list
y <- list("spring","summer", "fall","winter")
# creating dataframe
df <- data.frame(Working_days=c("Mon","Tues","Wednes"),
off_days=c("Sun","Satur","Thurs") )
# applying function to vector
# and return output as vector
map_chr(x,paste0," color")
# applying function to list
# and return output as vector
map(y,paste0," season")
# applying function to dataframe
# and return output as dataframe
map_df(df,paste0,"day")
输出:
对于向量:
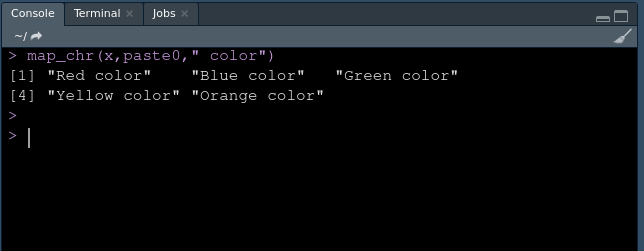
向量作为输出
对于列表:
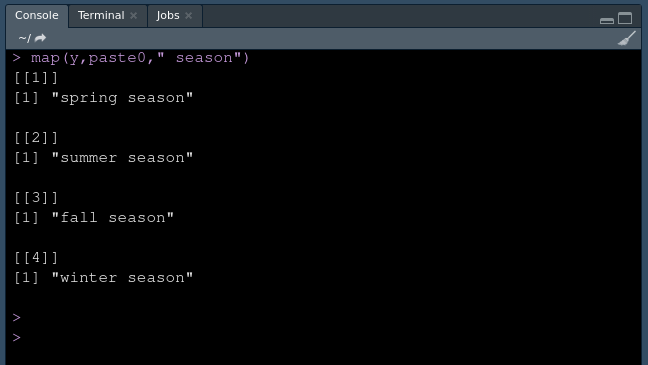
列出作为输出
对于数据框:
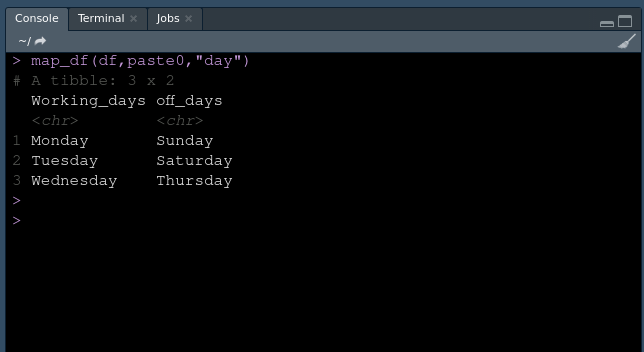
数据帧作为输出