给定整数current_row和current_col ,表示车在8 × 8棋盘上的当前位置,以及另外两个整数destination_row和destination_col ,表示车要到达的位置。任务是检查 Rook 是否有可能从其当前位置通过一次移动到达给定的目的地。如果发现是真的,打印“POSSIBLE” 。否则,打印“NOT POSSIBLE” 。
例子:
Input: current_row=8, current_col=8, destination_row=8, destination_col=4
Output: POSSIBLE
Explanation:
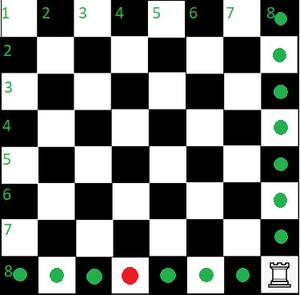
Possible
Input: current_row=3, current_col=2, destination_row=2, destination_col=4
Output: NOT POSSIBLE
Explanation:
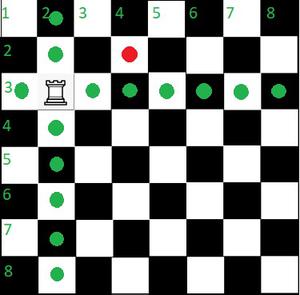
Not Possible
方法:可以使用以下观察来解决给定的问题:
On a chessboard, a rook can move as many squares as possible, horizontally as well as vertically, in a single move. Therefore, it can move to any position present in the same row or the column as in its initial position.
因此,问题简化为简单地检查以下两个条件:
- destination_row 和 current_row 是否相等。
- 否则,检查 destination_col 是否等于 current_col。
- 如果满足以上两个条件中的任何一个,则打印“ POSSIBLE ”。否则,打印“NOT POSSIBLE” 。
下面是上述方法的实现:
C++14
// C++ program to implement
// for the above approach
#include
using namespace std;
// Function to check if it is
// possible to reach destination
// in a single move by a rook
string check(int current_row, int current_col,
int destination_row, int destination_col)
{
if(current_row == destination_row)
return "POSSIBLE";
else if(current_col == destination_col)
return "POSSIBLE";
else
return "NOT POSSIBLE";
}
// Driver Code
int main()
{
// Given arrays
int current_row = 8;
int current_col = 8;
int destination_row = 8;
int destination_col = 4;
string output = check(current_row, current_col,
destination_row, destination_col);
cout << output;
return 0;
}
// This code is contributed by mohit kumar 29.
Java
// Java program for the above approach
import java.util.*;
import java.lang.*;
class GFG{
// Function to check if it is
// possible to reach destination
// in a single move by a rook
static String check(int current_row, int current_col,
int destination_row, int destination_col)
{
if(current_row == destination_row)
return "POSSIBLE";
else if(current_col == destination_col)
return "POSSIBLE";
else
return "NOT POSSIBLE";
}
// Driver code
public static void main(String[] args)
{
// Given arrays
int current_row = 8;
int current_col = 8;
int destination_row = 8;
int destination_col = 4;
String output = check(current_row, current_col,
destination_row, destination_col);
System.out.println(output);
}
}
// This code is contributed by code_hunt.
Python3
# Python program to implement
# for the above approach
# Function to check if it is
# possible to reach destination
# in a single move by a rook
def check(current_row, current_col,
destination_row, destination_col):
if(current_row == destination_row):
return("POSSIBLE")
elif(current_col == destination_col):
return("POSSIBLE")
else:
return("NOT POSSIBLE")
# Driver Code
current_row = 8
current_col = 8
destination_row = 8
destination_col = 4
output = check(current_row, current_col,
destination_row, destination_col)
print(output)
C#
// C# program to implement
// the above approach
using System;
class GFG
{
// Function to check if it is
// possible to reach destination
// in a single move by a rook
static string check(int current_row, int current_col,
int destination_row, int destination_col)
{
if(current_row == destination_row)
return "POSSIBLE";
else if(current_col == destination_col)
return "POSSIBLE";
else
return "NOT POSSIBLE";
}
// Driver Code
public static void Main()
{
// Given arrays
int current_row = 8;
int current_col = 8;
int destination_row = 8;
int destination_col = 4;
string output = check(current_row, current_col,
destination_row, destination_col);
Console.WriteLine(output);
}
}
// This code is contributed by susmitakundugoaldanga.
Javascript
POSSIBLE
时间复杂度: O(1)
空间复杂度:O(1)
如果您希望与专家一起参加现场课程,请参阅DSA 现场工作专业课程和学生竞争性编程现场课程。