使用Python获取 Google Drive 存储中的文件和文件夹列表
在本文中,我们将看看如何使用Python中的Google Drive API获取存储在 Google Drive 云存储中的文件(或文件夹)列表。它是一个 REST API,允许您从应用程序或程序中利用 Google Drive 存储。
因此,让我们创建一个与 Google Drive API 通信的简单Python脚本。
要求:
- Python (2.6 或更高版本)
- 启用了 Google Drive 的 Google 帐户
- Google API 客户端和 Google OAuth 库
安装:
通过运行以下命令安装所需的库:
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib
现在,按照以下步骤设置您的 Google 帐户以使用 Google Drive API。
- 转到 Google Cloud 控制台并使用您的 Google 帐户登录。
- 创建一个新项目。

创建一个新项目
- 转到API 和服务。

转到 API 和服务
- 为此项目启用Google Drive API 。

启用 API

启用 Google Drive API
- 转到OAuth 同意屏幕并为您的项目配置同意屏幕。
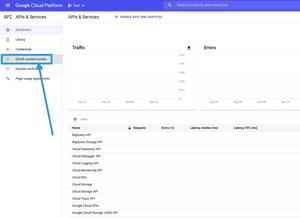
转到 OAuth 同意屏幕

选择外部并单击创建
- 输入您的应用程序的名称。它将显示在同意屏幕上。

输入应用程序名称并选择电子邮件地址
- 现在转到凭据。
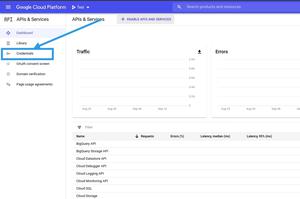
转到凭据
- 单击创建凭据,然后转到OAuth 客户端 ID。

单击创建凭据并选择 OAuth 客户端 ID
- 输入您的应用程序的名称,然后单击Create 。

输入应用程序名称,然后单击创建
- 您的客户 ID 将被创建。将其下载到您的计算机并将其另存为credentials.json
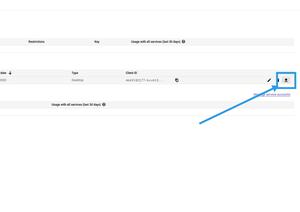
下载json文件
注意:不要与任何人分享您的客户 ID 或客户机密。
现在,我们完成了设置和安装。所以,让我们编写Python脚本:
Python3
# import the required libraries
import pickle
import os.path
from googleapiclient.discovery import build
from google_auth_oauthlib.flow import InstalledAppFlow
from google.auth.transport.requests import Request
# Define the SCOPES. If modifying it,
# delete the token.pickle file.
SCOPES = ['https://www.googleapis.com/auth/drive']
# Create a function getFileList with
# parameter N which is the length of
# the list of files.
def getFileList(N):
# Variable creds will store the user access token.
# If no valid token found, we will create one.
creds = None
# The file token.pickle stores the
# user's access and refresh tokens. It is
# created automatically when the authorization
# flow completes for the first time.
# Check if file token.pickle exists
if os.path.exists('token.pickle'):
# Read the token from the file and
# store it in the variable creds
with open('token.pickle', 'rb') as token:
creds = pickle.load(token)
# If no valid credentials are available,
# request the user to log in.
if not creds or not creds.valid:
# If token is expired, it will be refreshed,
# else, we will request a new one.
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials.json', SCOPES)
creds = flow.run_local_server(port=0)
# Save the access token in token.pickle
# file for future usage
with open('token.pickle', 'wb') as token:
pickle.dump(creds, token)
# Connect to the API service
service = build('drive', 'v3', credentials=creds)
# request a list of first N files or
# folders with name and id from the API.
resource = service.files()
result = resource.list(pageSize=N, fields="files(id, name)").execute()
# return the result dictionary containing
# the information about the files
return result
# Get list of first 5 files or
# folders from our Google Drive Storage
result_dict = getFileList(5)
# Extract the list from the dictionary
file_list = result_dict.get('files')
# Print every file's name
for file in file_list:
print(file['name'])
现在,运行脚本:
python3 script.py
这将尝试在您的默认浏览器中打开一个新窗口。如果失败,请从控制台复制 URL 并在浏览器中手动打开它。
现在,如果您尚未登录,请登录您的 Google 帐户。如果有多个帐户,系统会要求您选择其中一个。然后,单击“允许”按钮。
身份验证完成后,您的浏览器将显示一条消息,提示身份验证流程已完成。您可以关闭此窗口。
身份验证完成后,这将打印 Google Drive 存储中前N个文件(或文件夹)的名称。
注意:文件credentials.json应与Python脚本位于同一目录中。如果不是这样,您必须在程序中指定文件的完整路径。