使用 react、framer-motion 和 styled-components 的动画模式
在本文中,我们将学习如何使用 react、framer-motion 和 styled-components 创建动画模式。
先决条件:
- 了解 JavaScript (ES6)。
- 箭头函数(ES6)
- 三元运算符
- 文档.body.style
- 熟悉 HTML/CSS。
- ReactJS 的基础知识。
- 反应使用状态
Framer-motion:我们将在本教程中使用的组件和属性。
- https://www.framer.com/api/motion/component/
- https://www.framer.com/api/motion/animate-presence/
创建 React 应用程序并安装模块:
第 1 步:现在,您将使用 create-react-app 启动一个新项目,因此打开您的终端并输入。
npx create-react-app toggle-modal
第 2 步:创建项目文件夹后,即切换模式,使用以下命令移动到它。
cd toggle-modal
第 3 步:添加项目期间需要的 npm 包。
npm install framer-motion styled-components
//For yarn
yarn add framer-motion styled-components
第 5 步:现在使用您最喜欢的代码编辑器打开您新创建的项目,我正在使用 Visual Studio Code,并建议您使用相同的。
打开src文件夹并删除以下文件:
- 徽标.svg
- serviceWorker.js
- setupTests.js
- 索引.css
- App.test.js(如果有)
创建一个名为Styles.js 的文件。
项目结构:您的文件夹结构树应如下所示。
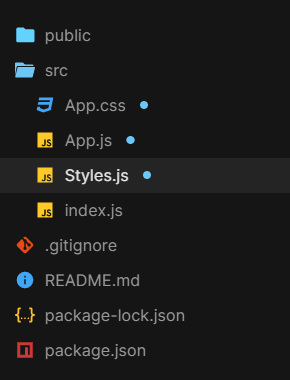
项目结构
方法:
- 我们将创建一个带有 'showModal' 属性的 Modal 组件,仅用于管理其可见性状态并使用 framer-motion AnimatePresence进行动画处理。
- AnimatePresence允许组件在从 React 树中移除时进行动画处理并启用退出动画。
- 为了给模态容器提供弹簧动画,我们将使用刚度 = 300 的 framer-motion弹簧动画。
- modal 的内容是 geeksforgeeks 图像,它也使用 framer-motion motion.div 进行动画处理。
- React useState hook 来管理“showModal”的状态,即负责切换模态容器。
- 'displayModal'实用函数将 'showModal' 值设置为与其上一个值相反的值以切换模式。
- 文档正文的事件侦听器,以便在单击外部或模式时,将“showModal”设置为 false,然后模式消失。
- ToggleBtn也使用 framer-motion motion.button进行动画处理。
例子:
Styles.js
import styled from "styled-components";
import { motion } from "framer-motion";
export const ModalBox = styled(motion.div)`
position: relative;
z-index: 2;
width: 400px;
height: 200px;
display: flex;
justify-content: center;
align-items: center;
background: #fff;
`;
export const ModalContent = styled(motion.div)`
padding: 5px;
`;
export const ModalContainer = styled.div`
height: 100vh;
background: #111;
display: flex;
flex-direction: column;
align-items: center;
`;
export const ToggleBtn = styled(motion.button)`
cursor: pointer;
font-size: 20px;
color: #fff;
padding: 0.5rem 0.8rem;
margin-top: 3rem;
background: #3bb75e;
text-decoration: none;
border: none;
border-radius: 50px;
`;
App.js
import React, { useState } from "react";
import { AnimatePresence } from "framer-motion";
import { ToggleBtn, ModalBox, ModalContent, ModalContainer } from "./Styles";
import "./App.css";
// Modal component with 'showModal' prop only
// to manage its state of visibility and
// animated using framer-motion
const Modal = ({ showModal }) => {
return (
{showModal && (
{/* Modal content is geeksforgeeks image */}
)}
);
};
const App = () => {
// React useState hook to manage the state of 'showModal'
// i.e. responsible to toggle the modal container
const [showModal, setShowModal] = useState(false);
// utility function to set the showModal value
// opposite of its last value
// to toggle modal
const displayModal = () => {
setShowModal(!showModal);
document.getElementById("btn").style.visibility = "hidden";
};
// event listener for document body
// so that on clicking outside the modal,
// 'showModal' is set to false.
document.body.addEventListener("click", () => {
if (showModal) {
setShowModal(false);
}
});
return (
Toggle Modal
{/* passing 'showModal' as a prop to Modal component */}
);
};
export default App;
index.js
import React from "react";
import ReactDOM from "react-dom";
import App from "./App";
ReactDOM.render(
,
document.getElementById("root")
);
App.css
* {
margin: 0;
box-sizing: border-box;
}
img {
padding: 5px;
width: 400px;
overflow: hidden;
}
应用程序.js
import React, { useState } from "react";
import { AnimatePresence } from "framer-motion";
import { ToggleBtn, ModalBox, ModalContent, ModalContainer } from "./Styles";
import "./App.css";
// Modal component with 'showModal' prop only
// to manage its state of visibility and
// animated using framer-motion
const Modal = ({ showModal }) => {
return (
{showModal && (
{/* Modal content is geeksforgeeks image */}
)}
);
};
const App = () => {
// React useState hook to manage the state of 'showModal'
// i.e. responsible to toggle the modal container
const [showModal, setShowModal] = useState(false);
// utility function to set the showModal value
// opposite of its last value
// to toggle modal
const displayModal = () => {
setShowModal(!showModal);
document.getElementById("btn").style.visibility = "hidden";
};
// event listener for document body
// so that on clicking outside the modal,
// 'showModal' is set to false.
document.body.addEventListener("click", () => {
if (showModal) {
setShowModal(false);
}
});
return (
Toggle Modal
{/* passing 'showModal' as a prop to Modal component */}
);
};
export default App;
index.js
import React from "react";
import ReactDOM from "react-dom";
import App from "./App";
ReactDOM.render(
,
document.getElementById("root")
);
应用程序.css
* {
margin: 0;
box-sizing: border-box;
}
img {
padding: 5px;
width: 400px;
overflow: hidden;
}
运行应用程序的步骤:使用以下命令从项目的根目录运行应用程序。
npm start
输出:现在打开浏览器并转到http://localhost:3000/ ,您将看到以下输出。