使用 framer-motion 和 ReactJS 的动画扩展卡
在本文中,我们将学习如何使用 react 和 framer 创建动画扩展卡片。
先决条件:
- 了解 JavaScript (ES6)。
我们将使用的 JavaScript 内置方法是:
- 箭头函数(ES6)
- 三元运算符
- JavaScript 中的对象
- 熟悉 HTML/CSS。
- ReactJS的基础知识
用于构建此应用程序的 React 钩子是:
- 反应使用状态
Framer:我们将在本教程中使用的组件和钩子是:
- https://www.framer.com/api/frame/
- https://www.framer.com/api/scroll/
- https://www.framer.com/api/utilities/#useanimation
创建 React 应用程序并安装模块:
第 1 步:现在,您将使用 create-react-app 启动一个新项目,因此打开您的终端并输入。
$ npx create-react-app animated-card
第 2 步:创建项目文件夹(即动画卡)后,使用以下命令移动到该文件夹。
$ cd animated-card
第 3 步:添加项目期间需要的 npm 包。
$ npm install framer react-icons // For yarn $ yarn add framer react-icons
打开src文件夹并删除以下文件:
- 徽标.svg
- serviceWorker.js
- setupTests.js
- 应用程序.css
- 应用程序.js
- App.test.js(如果有)
创建一个名为Card.js的文件。
项目结构:您的项目结构树应如下所示:
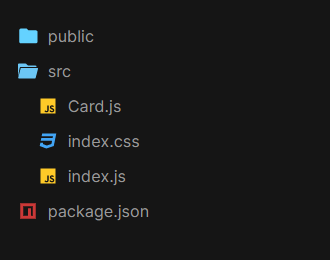
项目结构
例子:
index.js
import React from "react";
import { Frame, Scroll } from "framer";
import Card from "./Card";
import ReactDOM from "react-dom";
import "./index.css";
// main App HOC
export const App = () => {
return (
{/* Card component with props yPos,title,subtitle */}
);
};
const rootElement = document.getElementById("root");
ReactDOM.render( , rootElement);
index.css
body {
margin: 0;
cursor: pointer;
}
Card.js
import React, { useState } from "react";
import { ImCross } from "react-icons/im";
import { Frame, Scroll, useAnimation } from "framer";
// Card component with destructured props :
// yPos, title, subtitle
const Card = ({ yPos, title, subtitle }) => {
// useState hook to manage the state of
// expanding of card
const [state, setState] = useState(false);
// utility function to handle
// onTap on card component
const handleTap = () => {
state ? controls.start({ y: 0 }) : setState(!state);
};
const controls = useAnimation();
// Variants allow you to define animation
// states and organise them by name.
// They allow you to control animations
// throughout a component
// tree by switching a single animate prop.
const variants = {
active: {
width: 320,
height: 800,
borderRadius: 0,
overflow: "visible",
left: 28,
right:0,
y: 0,
transition: { duration: 0.125,
type: "spring",
damping: 10,
mass: 0.6 }
},
inactive: {
width: 280,
height: 280,
borderRadius: 24,
overflow: "hidden",
left: 45,
y: yPos,
transition: { duration: 0.125,
type: "spring",
damping: 10,
mass: 0.6 }
}
};
return (
// basic container for layout, styling,
// animation and events.
{title}
{subtitle}
{state && (
{
setState(false);
}}
>
)}
);
};
export default Card;
索引.css
body {
margin: 0;
cursor: pointer;
}
Card.js
import React, { useState } from "react";
import { ImCross } from "react-icons/im";
import { Frame, Scroll, useAnimation } from "framer";
// Card component with destructured props :
// yPos, title, subtitle
const Card = ({ yPos, title, subtitle }) => {
// useState hook to manage the state of
// expanding of card
const [state, setState] = useState(false);
// utility function to handle
// onTap on card component
const handleTap = () => {
state ? controls.start({ y: 0 }) : setState(!state);
};
const controls = useAnimation();
// Variants allow you to define animation
// states and organise them by name.
// They allow you to control animations
// throughout a component
// tree by switching a single animate prop.
const variants = {
active: {
width: 320,
height: 800,
borderRadius: 0,
overflow: "visible",
left: 28,
right:0,
y: 0,
transition: { duration: 0.125,
type: "spring",
damping: 10,
mass: 0.6 }
},
inactive: {
width: 280,
height: 280,
borderRadius: 24,
overflow: "hidden",
left: 45,
y: yPos,
transition: { duration: 0.125,
type: "spring",
damping: 10,
mass: 0.6 }
}
};
return (
// basic container for layout, styling,
// animation and events.
{title}
{subtitle}
{state && (
{
setState(false);
}}
>
)}
);
};
export default Card;
运行应用程序的步骤:使用以下命令从项目的根目录运行应用程序。
npm start
输出:现在打开浏览器并访问 http://localhost:3000/,您将看到以下输出: