使用 framer-motion 和 React 设计一个动画切换按钮
在本文中,我们将学习如何使用
- 成帧运动
- 反应.js
先决条件:
- 应该安装 NodeJS
- 了解 JavaScript (ES6)。
- 箭头函数(ES6)
- 三元运算符
- document.body.style.backgroundColor
- 熟悉 HTML/CSS。
- ReactJS 的基础知识。
- 反应使用状态
- 反应使用效果
创建 React 应用程序并安装模块:
第 1 步:现在,您将使用 create-react-app 启动一个新项目,因此打开您的终端并输入:
npx create-react-app toggle-switch
第 2 步:创建项目文件夹(即切换开关)后,使用以下命令移动到该文件夹。
cd toggle-switch
第 3 步:添加项目期间需要的 npm 包:
npm install framer-motion
要么
npm i framer-motion
第 4 步:现在使用您喜欢的代码编辑器打开您新创建的项目,我正在使用 Visual Studio Code,并建议您使用相同的代码。
打开 src 文件夹并删除以下文件:
- 徽标.svg
- serviceWorker.js
- setupTests.js
- 索引.css
- App.test.js(如果有)
项目结构:您的文件夹结构树应如下所示。
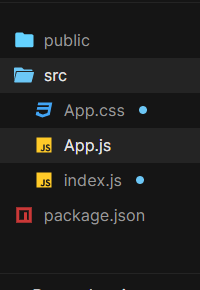
文件夹结构
我强烈建议您编写此代码,而不仅仅是复制粘贴和使用 CSS,根据您的喜好和需要对其进行一些调整。
方法:
- 我们将使用const & arrow函数来编写一个实用组件“ Switch” ,该组件将用于创建拨动开关。
- 在Switch中,我们将编写代码,根据通过解构作为 prop 传递的isOn的状态更改类名和图像源。
- 切换开关及其动画将使用 framer-motion 的motion.div组件和它的animate属性来实现。
- 在应用程序中,我们将使用useState钩子来管理“isOn”的状态,通过单击切换按钮来更改该状态,该按钮又用于更改类名、背景颜色和图像源。
- React useEffect hook 用于在“isOn”状态发生变化时创建副作用,以相应地更改整个页面的背景颜色。
例子:
App.js
import React from "react";
import { useState, useEffect } from "react";
import { motion } from "framer-motion";
import "./App.css";
const Switch = ({ isOn, ...rest }) => {
// initialize the customClassName according to the
// state of the "isOn" using ternary operator
const customClassName =
`toggleSwitch ${isOn ? "on" : "off"}`;
// initialize the src according to the
// state of the "isOn" using ternary operator
const src = isOn
?
"Toggle 1st Image link"
:
"Toggle 2nd Image link";
return (
);
};
const App = () => {
// useState hook is used to manage the state of
// "isOn" that is used to change the className,
// background-color and img src accordingly
const [isOn, setIsOn] = useState(false);
useEffect(() => {
// background-color changes every time "isOn"
// changes using JavaScript DOM methods
document.body.style.backgroundColor =
isOn ? "#1c1c1c" : "#ffffff";
}, [isOn]);
return
setIsOn(!isOn)} />;
};
export default App;
App.css
body {
margin: 0;
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
/* for smooth transition*/
transition: 0.5s ease-in-out;
}
* {
box-sizing: border-box;
}
.toggleSwitch {
width: 170px;
height: 100px;
border-radius: 100px;
padding: 10px;
display: flex;
cursor: pointer;
z-index: 2;
}
/* CSS for switch when "on"*/
.toggleSwitch.on {
background-color: #1aad66;
justify-content: flex-end;
/* for smooth transition*/
transition: 0.5s ease-in-out;
}
/*CSS for switch when "off"*/
.toggleSwitch.off {
background-color: #dddddd;
justify-content: flex-start;
}
.toggleSwitch div {
width: 80px;
height: 80px;
border-radius: 100%;
}
img {
width: 80px;
height: 80px;
border-radius: 100%;
}
index.js
import React from "react";
import ReactDOM from "react-dom";
import App from "./App";
const rootElement = document.getElementById("root");
ReactDOM.render(
,
rootElement
);
应用程序.css
body {
margin: 0;
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
/* for smooth transition*/
transition: 0.5s ease-in-out;
}
* {
box-sizing: border-box;
}
.toggleSwitch {
width: 170px;
height: 100px;
border-radius: 100px;
padding: 10px;
display: flex;
cursor: pointer;
z-index: 2;
}
/* CSS for switch when "on"*/
.toggleSwitch.on {
background-color: #1aad66;
justify-content: flex-end;
/* for smooth transition*/
transition: 0.5s ease-in-out;
}
/*CSS for switch when "off"*/
.toggleSwitch.off {
background-color: #dddddd;
justify-content: flex-start;
}
.toggleSwitch div {
width: 80px;
height: 80px;
border-radius: 100%;
}
img {
width: 80px;
height: 80px;
border-radius: 100%;
}
index.js
import React from "react";
import ReactDOM from "react-dom";
import App from "./App";
const rootElement = document.getElementById("root");
ReactDOM.render(
,
rootElement
);
运行应用程序的步骤:使用以下命令从项目的根目录运行应用程序。
npm start
输出:现在打开浏览器并转到http://localhost:3000/ ,您将看到以下输出。