React Native 中的 Redux 存储
在本文中,我们将了解 Redux Store。它 是保存应用程序状态的对象。 store 是 Redux 的组成部分之一。 Redux 是 JavaScript 应用程序中使用的状态管理库。它用于管理应用程序的数据和状态。
Redux 的使用:借助 redux,可以轻松管理状态和数据。随着我们应用程序的复杂性增加。一开始很难理解,但它确实有助于构建复杂的应用程序。一开始,感觉工作量很大,但确实很有帮助。
存储:存储是保存应用程序整个状态的对象。使用 store,我们可以保存和访问应用程序的当前状态。它有一个允许更新应用程序状态的调度方法。它还可以注册和注销侦听器回调。 Redux 应用程序中只能有一个 store。
在我们的应用程序中创建商店。我们使用 redux 库的 createStore API。此方法用于创建 redux 应用程序的存储。
句法:
const store = createStore(reducer);
正如我们在语法中看到的,createStore 方法中有一个 reducer 参数。现在,什么是减速器? Reducers 是纯函数,它获取当前状态和动作并返回新状态并告诉存储如何去做。
我们还可以使用以下语法创建商店:
createStore(reducer, [preloadedState], [enhancer])
- preloadedState:此参数是可选的。它可以用于应用程序的初始数据。
- 增强器:这也是一个可选参数。它可以改变商店的行为方式。基本上,它通过中间件、时间旅行、持久性等第三方能力增强了商店。
Store的重要方法:
getState():此方法用于获取商店的当前状态。
句法:
store.getState()
dispatch(action):该方法用于改变应用程序的状态。它将动作对象作为参数传递。基本上,在 dispatch action 之后,store 会运行它的 reducer函数(一个纯函数,它获取当前 state 和 action 并返回新的 state,并告诉 store 如何去做)。并将新值保存在其中。然后存储调用侦听器回调。
dispatch 方法将 action 作为参数。现在,什么是行动?动作是包含信息的 JavaScript 对象。操作是商店的唯一信息来源。
句法:
store.dispatch({ type: 'Action' })
订阅:此方法用于保存侦听器回调数组。这些监听器将在 action 被调度后被 redux Store 调用。它返回一个函数来删除监听器。
句法:
store.subscribe(()=>{ console.log(store.getState());})
现在我们知道了 store 和它的方法。下面是一个如何创建 react-redux 应用程序的示例。
创建 React-Redux 应用程序的步骤:
第 1 步:最初,使用下面提到的命令创建一个 React 应用程序:
npx create-react-app MyApp
第 2 步:创建项目文件夹(即 MyApp)后,使用以下命令移动到该文件夹。
cd MyApp
第 3 步:创建 ReactJS 应用程序后,安装 redux 和 react-redux
npm install redux react-redux
项目结构:它将如下所示:
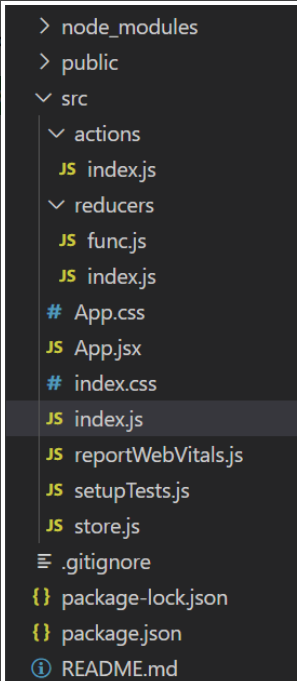
女朋友
示例:在此示例中,我们创建了两个按钮,一个将值递增 2,另一个将值递减 2,但如果值为 0,则它不会递减,我们只能递增它。使用 Redux,我们可以管理状态。
App.js
import React from "react";
import "./index.css";
import { useSelector, useDispatch } from "react-redux";
import { incNum, decNum } from "./actions/index";
function App() {
const mystate = useSelector((state) => state.change);
const dispatch = useDispatch();
return (
<>
Increment/Decrement the number by 2, using Redux.
{mystate}
>
);
}
export default App;
index.js
export const incNum = () => {
return { type: "INCREMENT" }
}
export const decNum = () => {
return { type: "DECREMENT" }
}
src/reducers/func.js
const initialState = 0;
const change = (state = initialState, action) => {
switch (action.type) {
case "INCREMENT": return state + 2;
case "DECREMENT":
if (state == 0) {
return state;
}
else {
return state - 2;
}
default: return state;
}
}
export default change;
src/reducer/index.js
import change from './func'
import { combineReducers } from 'redux';
const rootReducer = combineReducers({
change
});
export default rootReducer;
store.js
import { createStore } from 'redux';
import rootReducer from './reducers/index';
const store = createStore(rootReducer,
window.__REDUX_DEVTOOLS_EXTENSION__ &&
window.__REDUX_DEVTOOLS_EXTENSION__());
export default store;
src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App.jsx'
import store from './store';
import { Provider } from 'react-redux';
ReactDOM.render(
, document.getElementById("root")
);
index.js
export const incNum = () => {
return { type: "INCREMENT" }
}
export const decNum = () => {
return { type: "DECREMENT" }
}
src/reducers/func.js
const initialState = 0;
const change = (state = initialState, action) => {
switch (action.type) {
case "INCREMENT": return state + 2;
case "DECREMENT":
if (state == 0) {
return state;
}
else {
return state - 2;
}
default: return state;
}
}
export default change;
src/reducer/index.js
import change from './func'
import { combineReducers } from 'redux';
const rootReducer = combineReducers({
change
});
export default rootReducer;
store.js
import { createStore } from 'redux';
import rootReducer from './reducers/index';
const store = createStore(rootReducer,
window.__REDUX_DEVTOOLS_EXTENSION__ &&
window.__REDUX_DEVTOOLS_EXTENSION__());
export default store;
src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App.jsx'
import store from './store';
import { Provider } from 'react-redux';
ReactDOM.render(
, document.getElementById("root")
);
运行应用程序的步骤:使用以下命令从项目的根目录运行应用程序。
npm start
输出: