使用 OpenCV 从图像中裁剪人脸 – Python
Opencv 是一个Python库,主要用于图像处理和计算机视觉。在本文中,我们首先检测人脸,然后从图像中裁剪人脸。人脸检测是图像处理的一个分支,用于检测人脸。
我们将使用预训练的 Haar Cascade 模型从图像中检测人脸。 haar 级联是用于从图像中检测对象的对象检测方法。该算法由大量图像训练。您可以单击此处下载 face haar 级联文件。
让我们分步实施:
步骤1:在这一步中,我们将读取图像并将其转换为灰度。
Python3
import cv2
# Read the input image
img = cv2.imread('mpw.jpeg')
# Convert into grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
Python3
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_alt2.xml')
# Detect faces
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
Python3
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h),
(0, 0, 255), 2)
faces = img[y:y + h, x:x + w]
cv2.imshow("face",faces)
cv2.imwrite('face.jpg', faces)
cv2.imshow('img', img)
cv2.waitKey()
Python3
import cv2
# Read the input image
img = cv2.imread('mpw.jpeg')
# Convert into grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Load the cascade
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_alt2.xml')
# Detect faces
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
# Draw rectangle around the faces and crop the faces
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 0, 255), 2)
faces = img[y:y + h, x:x + w]
cv2.imshow("face",faces)
cv2.imwrite('face.jpg', faces)
# Display the output
cv2.imwrite('detcted.jpg', img)
cv2.imshow('img', img)
cv2.waitKey()
说明:在这段代码中,我们首先使用 import cv2 导入我们的 opencv 库。 cv2.imread() 方法从给定的路径加载图像(例如:mpw.jpeg 或 filepath/mpw.jpeg)加载图像后,我们使用 COLOR_BGR2GRAY 将图像转换为灰度图像。
步骤 2:使用 Haar Cascade 模型从图像中检测人脸。
蟒蛇3
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_alt2.xml')
# Detect faces
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
说明:我们使用 cv2.CascadeClassifier 在 face_cascade 中加载 haarcascade 文件。用于检测人脸的detectMultiScale()函数。它需要3个参数:
- 灰色:输入图像(灰度图像)
- 1.1:比例因子,它指定图像尺寸随着每个比例缩小多少。它改进了检测。
- 4:MinNeighbours,指定每个候选矩形应该保留多少邻居。
第 3 步:在图像中找到人脸。
蟒蛇3
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h),
(0, 0, 255), 2)
faces = img[y:y + h, x:x + w]
cv2.imshow("face",faces)
cv2.imwrite('face.jpg', faces)
cv2.imshow('img', img)
cv2.waitKey()
说明: x,y 是人脸的像素位置,w,h 是人脸的宽度和高度。 cv2.rectangle()函数用于在检测到的物体上绘制矩形,img 是输入图像,(x,y),(x+w, y+h) 是矩形的位置,(0,0,255) 是矩形的颜色此参数作为 BGR 的元组传递,我们将 (0,0,255) 用于红色,2 是矩形的厚度。
下面是完整的实现:
使用的图像 –
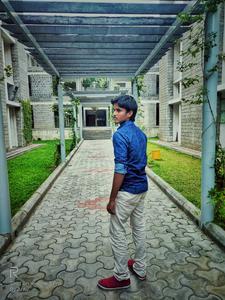
输入图像
蟒蛇3
import cv2
# Read the input image
img = cv2.imread('mpw.jpeg')
# Convert into grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Load the cascade
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_alt2.xml')
# Detect faces
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
# Draw rectangle around the faces and crop the faces
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 0, 255), 2)
faces = img[y:y + h, x:x + w]
cv2.imshow("face",faces)
cv2.imwrite('face.jpg', faces)
# Display the output
cv2.imwrite('detcted.jpg', img)
cv2.imshow('img', img)
cv2.waitKey()
输出:
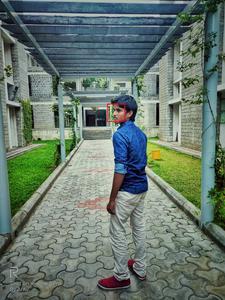
检测到的人脸

裁剪图像