使用Java在 PPT 中的幻灯片上格式化文本
要使用Java在 PowerPoint 演示文稿中设置幻灯片上的文本格式,请使用名为 Apache POI 的Java库。 Apache POI 是 Apache Software Foundation 运行的一个项目,之前是 Jakarta Project 的一个子项目,提供纯Java库,用于读写 Microsoft Office 格式的文件,例如 Word、PowerPoint 和 Excel。使用 Apache 指南为 Windows/Linux 系统安装 Apache POI 库。
方法:
- 使用 Apache POI包中的XMLSlideShow创建一个空的 Presentation Object。
- 创建 SlideMaster 对象并使用XSLFSlideMaster获取第一张幻灯片。
- 使用XSLFSlideLayout对象设置幻灯片的布局。
- 使用布局创建幻灯片。
- 使用XSLFTextShape对象获取 Slide 的第二个标题,并使用XSLFTextParagraph对象向其中添加 Paragraph。
- 使用XSLFTextRun对象向段落添加行并添加格式属性。
执行:
Java
// Formatting text on a slide in a PPT using java
import java.io.*;
// importing Apache POI environment packages
import org.apache.poi.xslf.usermodel.*;
public class FormatTextPPT {
public static void main(String args[])
throws IOException
{
// creating an empty presentation
XMLSlideShow ppt = new XMLSlideShow();
// creating the slide master object
XSLFSlideMaster slideMaster
= ppt.getSlideMasters().get(0);
// select a layout from specified slideLayout list
XSLFSlideLayout slidelayout = slideMaster.getLayout(
SlideLayout.TITLE_AND_CONTENT);
// creating a slide with title and content layout
XSLFSlide slide = ppt.createSlide(slidelayout);
// selection of title place holder
XSLFTextShape title = slide.getPlaceholder(1);
// clear the existing text in the slide
title.clearText();
// adding new paragraph
XSLFTextParagraph paragraph
= title.addNewTextParagraph();
// formatting line 1
XSLFTextRun line1 = paragraph.addNewTextRun();
line1.setText("Formatted Bold");
// making the text bold
line1.setBold(true);
// moving to the next line
paragraph.addLineBreak();
// formatting line 2
XSLFTextRun line2 = paragraph.addNewTextRun();
line2.setText("Formatted with Color");
// setting color to the text
line2.setFontColor(java.awt.Color.RED);
// setting font size to the text
line2.setFontSize(24.0);
// moving to the next line
paragraph.addLineBreak();
// formatting line 3
XSLFTextRun line3 = paragraph.addNewTextRun();
line3.setText("Formatted with Underline");
// underlining the text
line3.setUnderlined(true);
// setting color to the text
line3.setFontColor(java.awt.Color.GRAY);
// moving to the next line
paragraph.addLineBreak();
// formatting line 4
XSLFTextRun line4 = paragraph.addNewTextRun();
line4.setText("Text Formatted with Strike");
line4.setFontSize(12.0);
// making the text italic
line4.setItalic(true);
// setting color to the text
line4.setFontColor(java.awt.Color.BLUE);
// strike through the text
line4.setStrikethrough(true);
// setting font size to the text
line4.setFontSize(24.0);
// moving to the next line
paragraph.addLineBreak();
// getting path of current working directory
// to create the pdf file in the same directory of
// the running java program
String path = System.getProperty("user.dir");
path += "/FormattedText.pptx";
// creating a file object with the path specified
File file = new File(path);
FileOutputStream out = new FileOutputStream(file);
// saving the changes to a file
ppt.write(out);
out.close();
ppt.close();
System.out.println(
"PPT with Formatted Text created successfully!");
}
}
程序执行后:
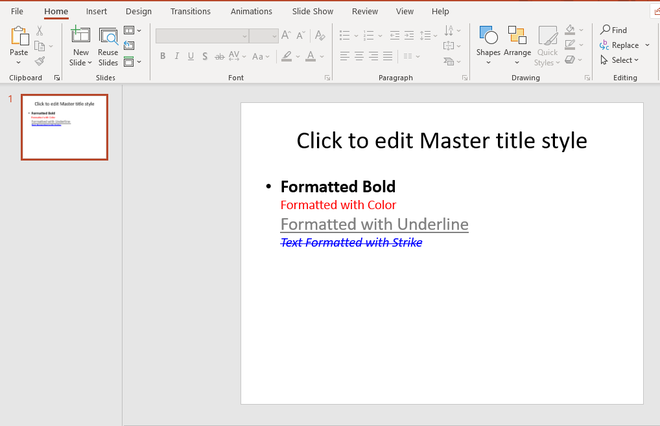
输出:FormattedText.ppt