📅  最后修改于: 2021-01-02 12:58:26             🧑  作者: Mango
Google Web Toolkit提供了多种创建自定义窗口小部件的方法。最简单的方法是通过组合现有的基本小部件并向其中添加一些交互逻辑来创建复合小部件。
要创建定制窗口小部件,它具有三个用于创建定制窗口小部件的通用概念,如下所示:
要创建复合窗口小部件,应扩展Composite Class 。组合是可以包含另一个组件的专用小部件。它的行为就像是它包含的小部件一样。在创建复合窗口小部件时,我们可以将现有窗口小部件的组合并为一个本身就是可重用窗口小部件的复合窗口。
窗口小部件以Java语言编写,位于com.google.gwt.user.client.ui软件包下。源代码在gwt-user.jar中。在此方法中创建基本窗口小部件是一种复杂的方法。许多基本小部件都是以这种方式编写的,例如Button和TextBox。
在实现直接从Widget基类派生的自定义Widget时,我们使用JavaScript编写了一些Widget方法。由于难以调试,最终实现了该方法。
// colorpicker.java
import com.google.gwt.event.dom.client.*;
import com.google.gwt.user.client.*;
import com.google.gwt.user.client.ui.*;
public class GwtColorPicker extends Composite
implements ClickHandler {
// The currently selected color name to give client-side
// feedback to the user.
protected Label currentcolor = new Label();
public GwtColorPicker() {
// Create a 4x4 grid of buttons with names for 16 colors
final Grid grid = new Grid(4, 4);
final String[] colors = new String[] { "aqua", "black",
"blue", "fuchsia", "gray", "green", "lime",
"maroon", "navy", "olive", "purple", "red",
"silver", "teal", "white", "yellow" };
int colornum = 0;
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++, colornum++) {
// Create a button for each color
Button button = new Button(colors[colornum]);
button.addClickHandler(this);
// Put the button in the Grid layout
grid.setWidget(i, j, button);
// Set the button background colors.
DOM.setStyleAttribute(button.getElement(),
"background",
colors[colornum]);
// For dark colors, the button label must be
// in white.
if ("black navy maroon blue purple"
.indexOf(colors[colornum]) != -1) {
DOM.setStyleAttribute(button.getElement(),
"color", "white");
}
}
}
// Create a panel with the color grid and currently
// selected color indicator.
final HorizontalPanel panel = new HorizontalPanel();
panel.add(grid);
panel.add(currentcolor);
// Set the class of the color selection feedback box
// to allow CSS styling. We need to obtain the DOM
// element for the current color label. This assumes
// that the element of the HorizontalPanel is
// the parent of the label element. Notice that the
// element has no parent before the widget has been
// added to the horizontal panel.
final Element panelcell =
DOM.getParent(currentcolor.getElement());
DOM.setElementProperty(panelcell, "className",
"colorpicker-currentcolorbox");
// Set initial color. This will be overridden with the
// value read from server.
setColor("white");
// Composite GWT widgets must call initWidget().
initWidget(panel);
}
/** Handles click on a color button. */
@Override
public void onClick(ClickEvent event) {
// Use the button label as the color name to set
setColor(((Button) event.getSource()).getText());
}
/** Sets the currently selected color. */
public void setColor(String newcolor) {
// Give client-side feedback by changing the color
// name in the label.
currentcolor.setText(newcolor);
// Obtain the DOM elements. This assumes that the
// element of the HorizontalPanel is the parent of the
// caption element.
final Element caption = currentcolor.getElement();
final Element cell = DOM.getParent(caption);
// Give feedback by changing the background color
DOM.setStyleAttribute(cell, "background", newcolor);
DOM.setStyleAttribute(caption, "background", newcolor);
if ("black navy maroon blue purple"
.indexOf(newcolor) != -1)
DOM.setStyleAttribute(caption, "color", "white");
else
DOM.setStyleAttribute(caption, "color", "black");
}
}
// colorpicker.css
/* Set style for the color picker table.
* This assumes that the Grid layout is rendered
* as a HTML . */
table.example-colorpicker {
border-collapse: collapse;
border: 0px;
}
/* Set color picker button style.
* This does not make assumptions about the HTML
* element tree as it only uses the class attributes
* of the elements. */
.example-colorpicker .gwt-Button {
height: 60px;
width: 60px;
border: none;
padding: 0px;
}
/* Set style for the right-hand box that shows the
* currently selected color. While this may work for
* other implementations of the HorizontalPanel as well,
* it somewhat assumes that the layout is rendered
* as a table where cells are elements. */
.colorpicker-currentcolorbox {
width: 240px;
text-align: center;
/* Must be !important to override GWT styling: */
vertical-align: middle !important;
}
输出:
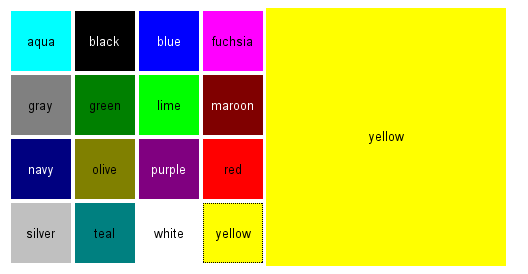