Alcuin序列是
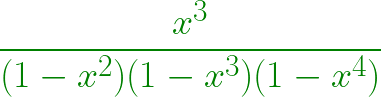
该系列具有重要意义,因为
- Alcuin序列a(n)是具有整数边的三角形的数量,并且三角形的周长是n。
- Alcuin序列a(n)是具有不同整数边的三角形的数量,并且三角形的周长为n + 6。
Alcuin序列如下:
0, 0, 1, 0, 1, 1, 2, 1, 3, 2
例子:
Input: n = 10
Output: 0, 0, 1, 0, 1,
1, 2, 1, 3, 2
Input: n = 15
Output:0, 0, 1, 0, 1,
1, 2, 1, 3, 2,
4, 3, 5, 4, 7,
方法:
- 使用以下公式找到Alcuin序列的第n个项
a(n)=圆(n ^ 2/12)–地板(n / 4)*地板((n + 2)/ 4) - 找出第i个术语并显示它。
下面是上述方法的实现:
C++
#include
using namespace std;
// find the nth term of
// Alcuin's sequence
int Alcuin(int n)
{
double _n = n, ans;
ans = round((_n * _n) / 12)
- floor(_n / 4)
* floor((_n + 2) / 4);
// return the ans
return ans;
}
// print first n terms of Alcuin number
void solve(int n)
{
int i = 0;
for (int i = 1; i <= n; i++) {
// display the number
cout << Alcuin(i) << ", ";
}
}
// Driver code
int main()
{
int n = 15;
solve(n);
return 0;
}
Java
// Java program for Alcuin's Sequence
import java.util.*;
class GFG
{
// find the nth term of
// Alcuin's sequence
static int Alcuin(int n)
{
double _n = n, ans;
ans = Math.round((_n * _n) / 12) -
Math.floor(_n / 4) *
Math.floor((_n + 2) / 4);
// return the ans
return (int) ans;
}
// print first n terms of Alcuin number
static void solve(int n)
{
int i = 0;
for (i = 1; i <= n; i++)
{
// display the number
System.out.print(Alcuin(i) + ", ");
}
}
// Driver code
public static void main(String[] args)
{
int n = 15;
solve(n);
}
}
// This code is contributed by Princi Singh
Python3
# Python3 program for Alcuin’s Sequence
from math import ceil, floor
# find the nth term of
# Alcuin's sequence
def Alcuin(n):
_n = n
ans = 0
ans = (round((_n * _n) / 12) -
floor(_n / 4) *
floor((_n + 2) / 4))
# return the ans
return ans
# print first n terms of Alcuin number
def solve(n):
for i in range(1, n + 1):
# display the number
print(Alcuin(i), end = ", ")
# Driver code
n = 15
solve(n)
# This code is contributed by Mohit Kumar
C#
// C# program for Alcuin's Sequence
using System;
class GFG
{
// find the nth term of
// Alcuin's sequence
static int Alcuin(int n)
{
double _n = n, ans;
ans = Math.Round((_n * _n) / 12) -
Math.Floor(_n / 4) *
Math.Floor((_n + 2) / 4);
// return the ans
return (int) ans;
}
// print first n terms of Alcuin number
static void solve(int n)
{
int i = 0;
for (i = 1; i <= n; i++)
{
// display the number
Console.Write(Alcuin(i) + ", ");
}
}
// Driver code
public static void Main(String[] args)
{
int n = 15;
solve(n);
}
}
// This code is contributed by Rajput-Ji
输出:
0, 0, 1, 0, 1, 1, 2, 1, 3, 2, 4, 3, 5, 4, 7,
参考: https : //en.wikipedia.org/wiki/Alcuin%27s_sequence