在 Y 节点组中将链表左旋转 X
给定一个单链表和两个整数X和Y ,任务是在Y节点组中将链表左旋转X 。
例子:
Input: 10 -> 20 -> 30 -> 40 -> 50 -> 60 -> 70 -> 80 -> 90 -> 100, X = 2, Y = 4
Output: 30 -> 40 -> 10 -> 20 -> 70 -> 80 -> 50 -> 60 -> 90 -> 100
Explanation: First group of nodes is 10->20->30->40.
After rotating by 2, it becomes 30->40->10->20.
Second group of nodes is 50->60->70->80.
After rotating by 2, it becomes 70->80->50->60.
Third group of nodes is 90->100.
After rotating by 2, it becomes 90->100.
Input: 40 -> 60 -> 70 -> 80 -> 90 -> 100, X = 1, Y = 3
Output: 70 -> 40 -> 60 -> 100 -> 80 -> 90
方法:该问题可以通过使用基于以下观察的反转算法进行旋转来解决:
Let A1 -> B1 -> A2 -> B2 ->…….-> AnBn represents the complete list where, AiBi represents a group of Y nodes, and Ai and Bi represents the separate parts of this group at the node of rotation i.e. Ai has X nodes and Bi has (Y – X) nodes.
For all Ai and Bi such that 1 ≤ i ≤ N
- Reverse A and B of size X and (Y-X) nodes to get ArBr, where Ar and Br are reverse of A and B respectively,
- Reverse ArBr of size Y to get BA.
请按照下面显示的图像更好地理解
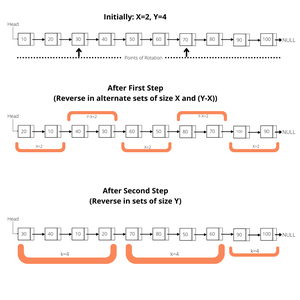
以 4 个节点为一组,将链表旋转 2 个节点
请按照以下步骤解决问题:
- 从头开始遍历链表:
- 选择 Y 节点组:
- 使用反转方法在这些组中旋转以向左旋转 X 个位置。
- 移动到下一组 Y 节点。
- 返回最终的链表。
下面是上述方法的实现:
C#
// C# program to rotate a linked list
using System;
public class LinkedList {
Node head;
// Linked list Node
public class Node {
public int data;
public Node next;
public Node(int d)
{
data = d;
next = null;
}
}
// Function to left rotate a list
// by x nodes in groups of y
Node leftRotate(int x, int y)
{
Node prev = reverse(head, x,
y - x, true);
return reverse(prev, y, y, true);
}
// Function to reverse list in
// alternate groups of size m and n
Node reverse(Node head, int m, int n,
bool isfirstHalf)
{
if (head == null)
return null;
Node current = head;
Node next = null;
Node prev = null;
int count = 0, k = m;
if (!isfirstHalf)
k = n;
// Reverse first k nodes of linked list
while (count < k && current != null) {
next = current.next;
current.next = prev;
prev = current;
current = next;
count++;
}
// Next is now a pointer
// to (k+1)th node
// Recursively call for the list
// starting from current and
// make rest of the list
// as next of first node
if (next != null)
head.next
= reverse(next, m, n,
!isfirstHalf);
// prev is now head of input list
return prev;
}
// Function to push a new node
// on the front of the list.
void push(int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
// Function to print list
void printList()
{
Node temp = head;
while (temp != null) {
Console.Write(temp.data + " ");
temp = temp.next;
}
Console.WriteLine();
}
// Driver code
public static void Main()
{
LinkedList llist = new LinkedList();
// Create a list
// 10->20->30->40->50->60
// ->70->80->90->100
for (int i = 100; i >= 10; i -= 10)
llist.push(i);
Console.WriteLine("Given list");
llist.printList();
llist.head = llist.leftRotate(2, 4);
Console.WriteLine("Rotated Linked List");
llist.printList();
}
}
Python3
# Python program to rotate a linked list
# Linked list Node
class Node :
def __init__(self,d):
self.data = d
self.next = None
class LinkedList :
def __init__(self):
self.head = None
# Function to left rotate a list
# by x nodes in groups of y
def leftRotate(self,x,y):
prev = self.reverse(self.head, x, y - x, True)
return self.reverse(prev, y, y, True)
# Function to reverse list in
# alternate groups of size m and n
def reverse(self,head,m,n,isfirstHalf):
if (head == None):
return None
current = head
next = None
prev = None
count,k = 0,m
if (isfirstHalf == False):
k = n
# Reverse first k nodes of linked list
while (count < k and current != None) :
next = current.next
current.next = prev
prev = current
current = next
count += 1
# Next is now a pointer
# to (k+1)th node
# Recursively call for the list
# starting from current and
# make rest of the list
# as next of first node
if (next != None):
head.next = self.reverse(next, m, n,~isfirstHalf)
# prev is now head of input list
return prev
# Function to push a new node
# on the front of the list.
def push(self,new_data):
new_node = Node(new_data)
new_node.next = self.head
self.head = new_node
# Function to print list
def printList(self):
temp = self.head
while (temp != None) :
print(temp.data,end = " ")
temp = temp.next
print()
# Driver code
llist = LinkedList()
# Create a list
# 10->20->30->40->50->60
# ->70->80->90->100
for i in range(100,9,-10):
llist.push(i)
print("Given list")
llist.printList()
llist.head = llist.leftRotate(2, 4)
print("Rotated Linked List")
llist.printList()
# This code is contributed by shinjanpatra
Javascript
Given list
10 20 30 40 50 60 70 80 90 100
Rotated Linked List
30 40 10 20 70 80 50 60 90 100
时间复杂度: O(N) 其中 N 是链表的长度
辅助空间: O(1)