使用 Tesseract 从图像中读取文本
Pytesseract或Python-tesseract是用于Python的光学字符识别 (OCR) 工具。它将读取和识别图像、车牌等中的文本。在这里,我们将使用 tesseract 包从给定图像中读取文本。
这里主要涉及3个简单的步骤,如下所示:-
- 加载从计算机保存的图像或使用浏览器下载它,然后加载相同的图像。 (任何带文字的图像)。
- 二值化图像(将图像转换为二进制)。
- 然后我们将通过 OCR 系统传递图像。
执行:
以下Python代码表示文本的本地化并正确猜测图像中写入的文本。
Python3
# We import the necessary packages
#import the needed packages
import cv2
import os,argparse
import pytesseract
from PIL import Image
#We then Construct an Argument Parser
ap=argparse.ArgumentParser()
ap.add_argument("-i","--image",
required=True,
help="Path to the image folder")
ap.add_argument("-p","--pre_processor",
default="thresh",
help="the preprocessor usage")
args=vars(ap.parse_args())
#We then read the image with text
images=cv2.imread(args["image"])
#convert to grayscale image
gray=cv2.cvtColor(images, cv2.COLOR_BGR2GRAY)
#checking whether thresh or blur
if args["pre_processor"]=="thresh":
cv2.threshold(gray, 0,255,cv2.THRESH_BINARY| cv2.THRESH_OTSU)[1]
if args["pre_processor"]=="blur":
cv2.medianBlur(gray, 3)
#memory usage with image i.e. adding image to memory
filename = "{}.jpg".format(os.getpid())
cv2.imwrite(filename, gray)
text = pytesseract.image_to_string(Image.open(filename))
os.remove(filename)
print(text)
# show the output images
cv2.imshow("Image Input", images)
cv2.imshow("Output In Grayscale", gray)
cv2.waitKey(0)
现在,按照以下步骤成功地从图像中读取文本:
- 将要从中读取文本的代码和图像保存在同一文件中。
- 打开命令提示符。转到保存代码文件和图像的位置。
- 执行以下命令以查看输出。
示例 1:
执行以下命令以查看输出。
python tesseract.py --image Images/title.png
我们显示了原始图像。
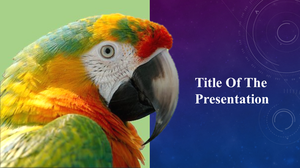
标题
我们显示了灰度图像。 (p.png)
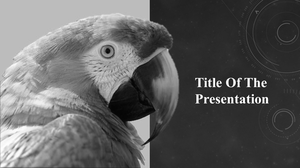
p
输出:
示例 2:
执行以下命令以查看输出。
python tesseract.py --image Images/OCR.png
我们显示了原始图像。
光学字符识别
我们显示了灰度图像。 (p.png)
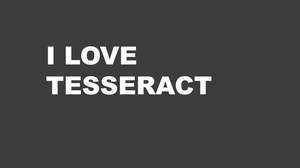
p
输出: