Swift – 集合操作
Set 是唯一值的集合。可以使用初始化程序或集合字面量语法创建集合。也可以通过将唯一值数组添加到空集来创建集。当我们想要删除重复值时,我们可以使用集合而不是数组。集合是无序的,不维护索引,因此不能通过索引访问。
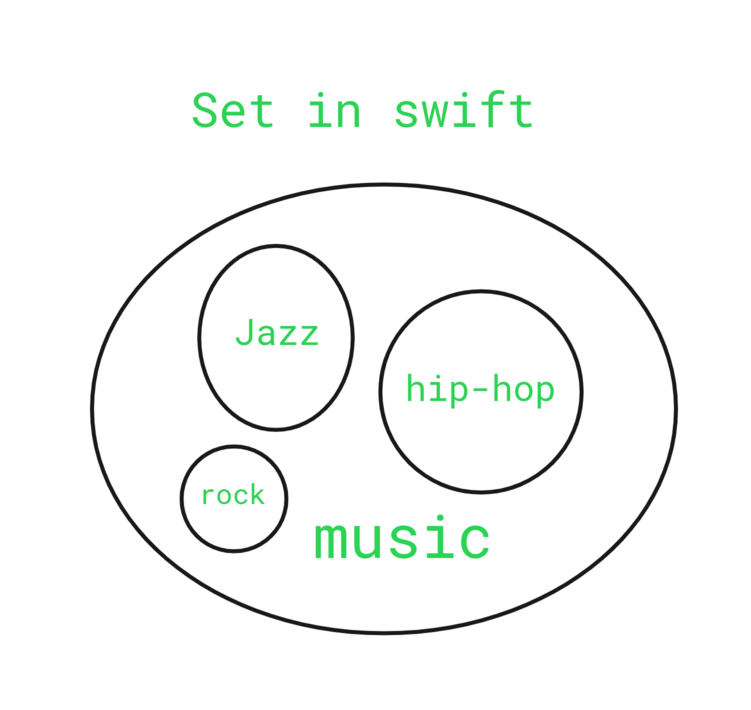
放
在这里,我们使用音乐数组来存储音乐流派。名为music的集合包含了rock、jazz、hip-hop等值。一般来说,我们可以将集合看成是唯一值的集合。在我们的示例中,我们以音乐为例,我们将摇滚、爵士、嘻哈的值存储在音乐中。
在 Swift 中创建集合
我们可以创建一个包含任何类型的可散列值的集合。 Hashable 意味着可以对值进行散列和比较。在 swift 中,字符串、数字和布尔类型是可散列的。要创建一个新集合,只需使用集合字面量语法。
句法:
var set_name: Set
这里,set_name 是集合的名称,type 是集合中值的类型。
例子:
Swift
// Swift program to create a set of strings
import Swift
// Creating a set of string
var setname: Set = [ "a", "b", "c", "d", "e" ]
// Displaying the set
print(setname)
Swift
// Swift program to find the union of two sets
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Displaying the union of setA and setB
// Using union() method
print("Union of setA and setB:", setA.union(setB))
Swift
// Swift program to find the intersection of two sets
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Displaying the intersection of setA and setB
// Using intersection() method
print("Intersection of setA and setB:", setA.intersection(setB))
Swift
// Swift program to find the subtracting of two sets
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Displaying the subtracting of setA and setB
// Using subtracting() method
print("Subtracting of setA and setB:", setA.subtracting(setB))
Swift
// Swift program to find the symmetricDifference of two sets
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Displaying the symmetricDifference of setA and setB
// Using symmetricDifference() method
print("SymmetricDifference of setA and setB:",
setA.symmetricDifference(setB))
Swift
// Swift program to check the given sets are equal or not
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Checking setA is equal to setB
// Using == operator
print("Is setA is equal to setB: ",
setA == setB)
Swift
// Swift program to check the given set is the subset or not
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Checking setB is the subset of setA
// Using isSubset() method
print("Is setB is the subset of setA and setB:",
setB.isSubset(of: setA))
输出:
["a", "b", "c", "d", "e"]
设置操作
在 Swift 中,我们还可以执行各种集合操作,例如将两个集合连接在一起,查找两个集合之间的差异,检查一个集合是否为空,以及使用它们的值比较两个集合。或者我们可以说 Swift 提供了内置的方法来执行集合操作,例如并集、交集、差集和对称差集。下面是各种集合操作的简单示意图。
联盟
联合是两个集合中都存在的所有元素的集合,它不包含重复的元素。例如,我们有两个集合:集合 a 和集合 b。所以这两个集合的并集包含集合 a 和集合 b 中的所有元素。在这里,在上图中,图中突出显示的绿色部分显示这些区域包含在结果集中。集合 a 和集合 b 区域都包含在集合的结果并集中。
Swift 提供了一个内置的 union() 方法来查找两个集合的并集。或者我们可以说这个方法返回一个包含集合 a 和集合 b 的所有元素的新集合。
句法:
setA.union(setB)
这里,setA 是第一个集合的名称,setB 是第二个集合的名称。
例子:
迅速
// Swift program to find the union of two sets
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Displaying the union of setA and setB
// Using union() method
print("Union of setA and setB:", setA.union(setB))
输出:
Set A: [3, 4, 5, 2, 1]
Set B: [3, 4, 6, 5, 7]
Union of setA and setB: [4, 3, 6, 2, 5, 7, 1]
在这里,我们可以看到,它打印出两个集合的并集,即 [4, 3, 6, 2, 5, 7, 1]。这些元素位于两组中的任何一组中。
路口
交集是只包含两个集合中存在的公共元素的集合。例如,我们有两个集合:集合 a 和集合 b。因此,交集包含集合 a 和集合 b 中存在的公共元素。此处图表中突出显示的绿色部分显示了两组共有的区域。所以两个集合的结果交集只有共同的元素。
Swift 提供了一个内置的 intersection() 方法来查找两个集合的交集。或者我们可以说这个方法返回一个包含集合 a 和集合 b 的所有公共元素的新集合。
句法:
setA.intersection(setB)
这里,setA 是第一个集合的名称,setB 是第二个集合的名称。
例子:
迅速
// Swift program to find the intersection of two sets
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Displaying the intersection of setA and setB
// Using intersection() method
print("Intersection of setA and setB:", setA.intersection(setB))
输出:
Set A: [3, 2, 4, 5, 1]
Set B: [7, 3, 4, 5, 6]
Intersection of setA and setB: [4, 3, 5]
在这里,我们可以看到,它打印出两个集合的交集,即 [4, 3, 5]。这些元素仅在两组中。
减法
减法是在第一个集合中但不在第二个集合中的元素集合。例如,我们有两个集合:集合 a 和集合 b。因此,减法包含存在于集合 a 中但不存在于集合 b 中的元素。这里,图中突出显示的绿色部分显示了在两组之间执行减法运算后包含在结果组中的区域。
Swift 提供了一个内置的 Subtracting() 方法来查找两组之间的差异。或者我们可以说这个方法返回一个新的集合,其中包含集合 a 中存在但不存在于集合 b 中的元素。
句法:
setA.subtracting(setB)
这里,setA 是第一个集合的名称,setB 是第二个集合的名称。
例子:
迅速
// Swift program to find the subtracting of two sets
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Displaying the subtracting of setA and setB
// Using subtracting() method
print("Subtracting of setA and setB:", setA.subtracting(setB))
输出:
Set A: [4, 5, 1, 2, 3]
Set B: [3, 4, 5, 7, 6]
Subtracting of setA and setB: [1, 2]
在这里,如我们所见,它打印出两组的差异,即 [1, 2]。这些元素存在于第一组中,但不存在于第二组中。
对称差
对称差是在两个集合中的任何一个中但不在这两个集合中的所有元素的集合。例如,我们有两个集合:集合 a 和集合 b。所以对称差包含两个集合中的任何一个但不在这两个集合中的元素。这里,图中突出显示的绿色部分显示了在对两个集合执行对称差分运算后包含在结果集合中的区域。在此操作中,两个集合中共有的元素将被忽略。
Swift 提供了一个内置的 symmetricDifference() 方法来创建一个新集合,其中任一集合中的值,但不是两者。其中 a 和 b 代表我们正在执行对称差分操作的两个集合。
句法:
setA.symmetricDifference(setB)
这里,setA 是第一个集合的名称,setB 是第二个集合的名称。
例子:
迅速
// Swift program to find the symmetricDifference of two sets
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Displaying the symmetricDifference of setA and setB
// Using symmetricDifference() method
print("SymmetricDifference of setA and setB:",
setA.symmetricDifference(setB))
输出:
Set A: [3, 1, 2, 4, 5]
Set B: [6, 5, 3, 7, 4]
SymmetricDifference of setA and setB: [6, 1, 2, 7]
在这里,我们可以看到,它打印出两组的对称差,即 [6, 1, 2, 7]。那些在两个集合中的任何一个中但不在这两个集合中的元素。
检查两组是否相等
Swift 提供了一个 ==运算符。此运算符用于检查两个集合是否相等。如果两个集合相等,此运算符将返回 true。或者,如果两个集合不相等,它将返回 false。
句法:
setA == setB
这里,setA 是第一个集合的名称,setB 是第二个集合的名称。
例子:
迅速
// Swift program to check the given sets are equal or not
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Checking setA is equal to setB
// Using == operator
print("Is setA is equal to setB: ",
setA == setB)
输出:
Set A: [2, 4, 5, 3, 1]
Set B: [4, 5, 6, 7, 3]
Is setA is equal to setB: false
检查集合的子集
如果第一个集合的所有元素都存在于第二个集合中,则称一个集合是另一个集合的子集,如下图所示。 Swift 提供了一个内置方法 isSubset()。此方法用于检查集合 b 的所有值是否在集合 a 中可用。如果集合是子集,则此方法将返回 true,否则将返回 false。
句法:
setB.isSubset(of: setA)
这里,setB 是我们要检查它是否是 setA 的子集的集合。
例子:
迅速
// Swift program to check the given set is the subset or not
// Creating set A of integers
var setA: Set = [1, 2, 3, 4, 5]
// Displaying set A
print("Set A:", setA)
// Creating set B of integers
var setB: Set = [3, 4, 5, 6, 7]
// Displaying set B
print("Set B:", setB)
// Checking setB is the subset of setA
// Using isSubset() method
print("Is setB is the subset of setA and setB:",
setB.isSubset(of: setA))
输出:
Set A: [3, 2, 1, 4, 5]
Set B: [4, 6, 3, 5, 7]
Is setB is the subset of setA and setB: false