Node.js NPM shortid 模块
NPM(Node Package Manager) 是 Node.js 包的包管理器。有一个名为“shortid”的 NPM 包用于创建短的非顺序 url 友好的唯一 ID。默认情况下,它使用 7-14 个 url 友好字符:AZ、az、0-9、_-。它支持集群(自动)、自定义种子、自定义字母。它可以生成任意数量的 id 而不会重复。
安装命令:
npm install shortid
将包导入本地文件的语法-
const shortid = require('shortid')
创建唯一 ID 的语法
const newId = shortid.generate()
在 shortid 模块上定义了一些方法来创建唯一 id 和自定义 id。一些方法如下所示。
shortid.generate() -用于创建唯一 ID。
例子:
users.insert({
_id: shortid.generate(),
name: '...',
email: '...'
});
shortid.isValid(id) -用于检查 id 是否有效。
例子:
shortid.isValid('41GHDbE');
// true
shortid.isValid('i have spaces');
// false
短的。 字符(字符) -用于自定义 id。
例子:
shortid.characters('ⒶⒷⒸⒹⒺⒻⒼⒽⒾⒿⓀⓁⓂⓃⓄⓅⓆⓇⓈⓉ'
+ 'ⓊⓋⓌⓍⓎⓏⓐⓑⓒⓓⓔⓕⓖⓗⓘⓙⓚⓛⓜⓝⓞⓟⓠⓡⓢⓣⓤⓥ'
+ 'ⓦⓧⓨⓩ①②③④⑤⑥⑦⑧⑨⑩⑪⑫');
示例 1:此示例说明如何生成和使用 shortid 包来创建唯一 ID。
filename-index.js :此文件包含创建短 id 并将其与用户信息附加并保存到数据库的所有逻辑。
const express = require('express')
const bodyParser = require('body-parser')
const shortid = require('shortid')
const formTemplet = require('./form')
const repo = require('./repository')
const app = express()
const port = process.env.PORT || 3000
// The body-parser middleware to parse form data
app.use(bodyParser.urlencoded({extended : true}))
// Get route to display HTML form
app.get('/', (req, res) => {
res.send(formTemplet({}))
})
// Post route to handle form submission logic and
app.post('/', (req, res) => {
// Fetching user inputs
const {name, email} = req.body
// Creating new unique id
const userId = shortid.generate()
// Saving record to the database
// with attaching userid to each record
repo.create({
userId,
name,
email
})
res.send('Information submitted!')
})
// Server setup
app.listen(port, () => {
console.log(`Server start on port ${port}`)
})
文件名——repository.js:该文件包含创建数据库并与之交互的所有逻辑。
// Importing node.js file system module
const fs = require('fs')
class Repository {
constructor(filename) {
// Filename where data are going to store
if(!filename) {
throw new Error(
'Filename is required to create a datastore!')
}
this.filename = filename
try {
fs.accessSync(this.filename)
} catch(err) {
// If file not exist it is created
// with empty array
fs.writeFileSync(this.filename, '[]')
}
}
// Get all existing records
async getAll(){
return JSON.parse(
await fs.promises.readFile(this.filename, {
encoding : 'utf8'
})
)
}
// Create new record
async create(attrs){
// Fetch all existing records
const records = await this.getAll()
// All the existing records with new
// record push back to database
records.push(attrs)
await fs.promises.writeFile(
this.filename,
JSON.stringify(records, null, 2)
)
return attrs
}
}
// The 'datastore.json' file created
// at runtime and all the information
// provided via signup form store in
// this file in JSON format.
module.exports =
new Repository('datastore.json')
文件名 - form.js:此文件包含呈现表单的所有逻辑。
module.exports = ({errors}) => {
return `
`
}
输出:
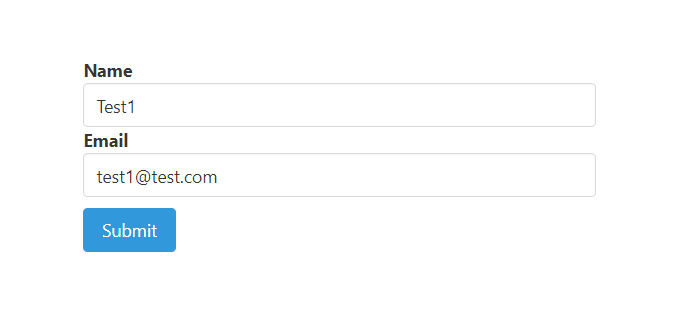
提交信息1
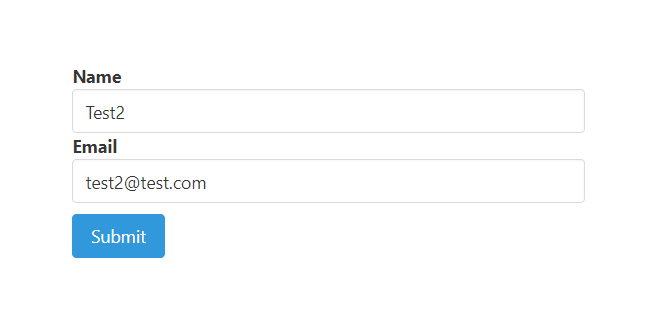
提交信息2
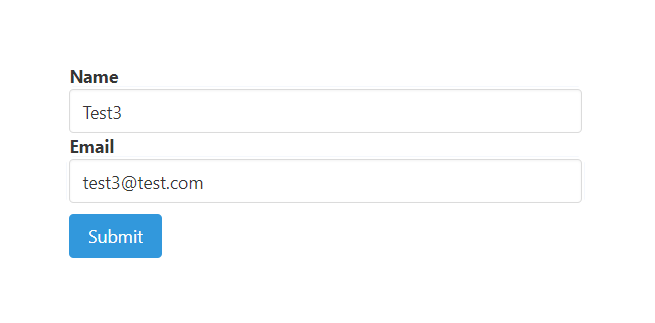
提交信息3
数据库:
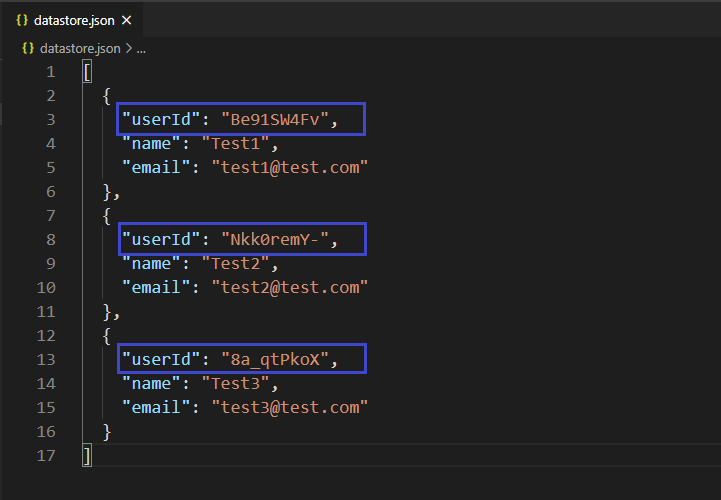
提交信息后的数据库
示例 2:此示例说明了如何自定义和使用 shortid 包来创建唯一 ID。
filename-index.js:此文件包含创建短 id 并将其与用户信息附加并保存到数据库的所有逻辑。
const express = require('express')
const bodyParser = require('body-parser')
const shortid = require('shortid')
const formTemplet = require('./form')
const repo = require('./repository')
const app = express()
const port = process.env.PORT || 3000
// The body-parser middleware to parse form data
app.use(bodyParser.urlencoded({extended : true}))
// Get route to display HTML form
app.get('/', (req, res) => {
res.send(formTemplet({}))
})
// Post route to handle form
// submission logic and
app.post('/', (req, res) => {
// Fetching user inputs
const {name, email} = req.body
// Customising id creation
shortid.characters('ⒶⒷⒸⒹⒺⒻⒼⒽⒾ'
+ 'ⒿⓀⓁⓜⓃⓄⓅⓆⓇⓈⓉⓊⓋⓌⓍⓎⓏ'
+ 'ⓐⓑⓒⓓⓔⓕⓖⓗⓘⓙⓚⓛⓜⓝⓞⓟⓠ'
+ 'ⓡⓢⓣⓤⓥⓦⓧⓨⓩ①②③④⑤⑥⑦⑧⑨⑩⑪⑫')
// Creating new unique id
const userId = shortid.generate()
// Saving record to the database
// with attaching userid to each record
repo.create({
userId,
name,
email
})
res.send('Information submitted!')
})
// Server setup
app.listen(port, () => {
console.log(`Server start on port ${port}`)
})
文件名——repository.js:该文件包含创建数据库并与之交互的所有逻辑。
// Importing node.js file system module
const fs = require('fs')
class Repository {
constructor(filename) {
// Filename where data are going to store
if(!filename) {
throw new Error(
'Filename is required to create a datastore!')
}
this.filename = filename
try {
fs.accessSync(this.filename)
} catch(err) {
// If file not exist it is
// created with empty array
fs.writeFileSync(this.filename, '[]')
}
}
// Get all existing records
async getAll(){
return JSON.parse(
await fs.promises.readFile(this.filename, {
encoding : 'utf8'
})
)
}
// Create new record
async create(attrs){
// Fetch all existing records
const records = await this.getAll()
// All the existing records with new
// record push back to database
records.push(attrs)
await fs.promises.writeFile(
this.filename,
JSON.stringify(records, null, 2)
)
return attrs
}
}
// The 'datastore.json' file created
// at runtime and all the information
// provided via signup form store in
// this file in JSON formet.
module.exports =
new Repository('datastore.json')
文件名 - form.js:此文件包含呈现表单的所有逻辑
const getError = (errors, prop) => {
try {
return errors.mapped()[prop].msg
} catch (error) {
return ''
}
}
module.exports = ({errors}) => {
return `
`
}
输出:
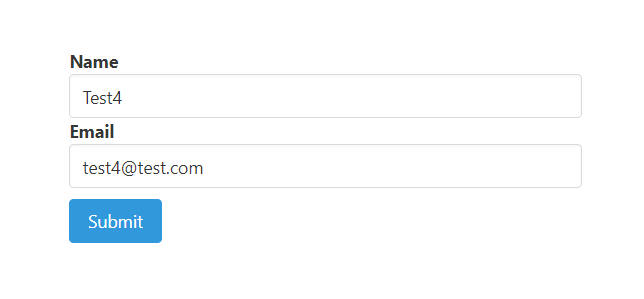
提交信息1
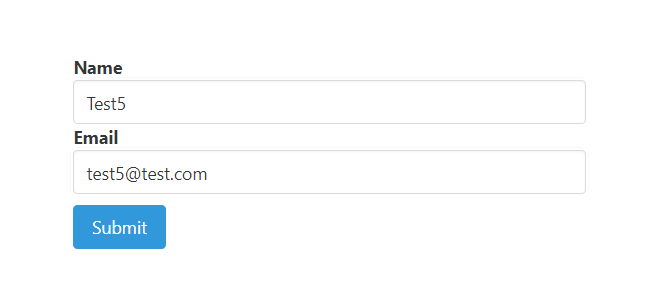
提交资料2
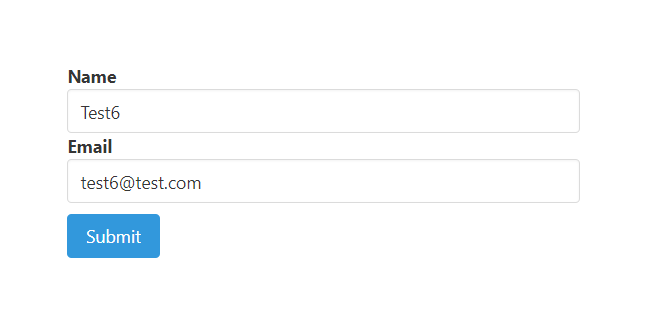
提交资料 3
数据库:
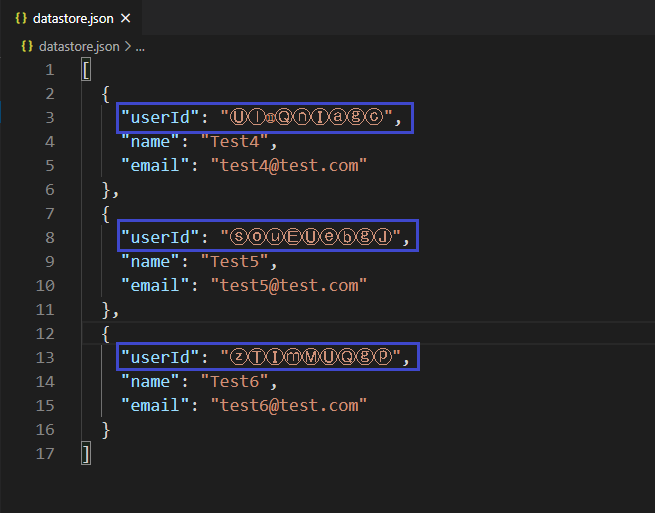
提交信息后的数据库
注意:我们在 form.js 文件中使用了一些 Bulma 类来设计我们的内容。