找到可以写成 N 的不同幂的第 K 个数
给定 两个正整数N和K 。任务是找到可以写为N的不同非负幂之和的第 K个数。
例子:
Input: N = 3, K = 4
Output: 9
Explanation:
First number that can be written as sum of powers of 3 is [1 = 30]
Second number that can be written as sum of powers of 3 is [3 = 31]
Third number that can be written as sum of powers of 3 is [4 = 30 + 31]
Fourth number that can be written as sum of powers of 3 is [9 = 32]
Therefore answer is 9.
Input: N = 2, K = 12
Output: 12
方法:这个问题可以通过使用十进制到二进制转换的概念来解决。这个想法是找到 K 的二进制表示并开始从最低有效位迭代到最高有效位。如果设置了当前位,则在答案中包含相应的 N 次方,否则跳过该位。 请参阅下图以更好地理解。
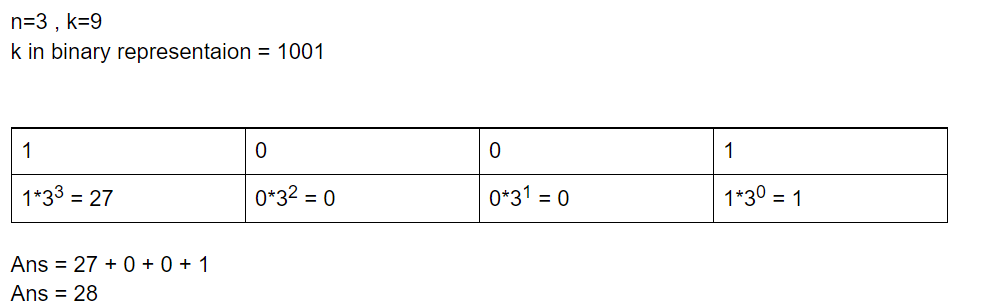
示例:当 N = 3 时,K = 9
下面是上述方法的实现:
C++
// C++ program for the above approach
#include
using namespace std;
// Function to find Kth number that can be
// represented as sum of different
// non-negative powers of N
int FindKthNum(int n, int k)
{
// To store the ans
int ans = 0;
// Value of current power of N
int currPowOfN = 1;
// Iterating through bits of K
// from LSB to MSB
while (k) {
// If the current bit is 1 then include
// corresponding power of n into ans
if (k & 1) {
ans = ans + currPowOfN;
}
currPowOfN = currPowOfN * n;
k = k / 2;
}
// Return the result
return ans;
}
// Driver Code
int main()
{
int N = 3;
int K = 4;
cout << FindKthNum(N, K);
}
Java
// Java program for the above approach
class GFG
{
// Function to find Kth number that can be
// represented as sum of different
// non-negative powers of N
static int FindKthNum(int n, int k)
{
// To store the ans
int ans = 0;
// Value of current power of N
int currPowOfN = 1;
// Iterating through bits of K
// from LSB to MSB
while (k > 0)
{
// If the current bit is 1 then include
// corresponding power of n into ans
if ((k & 1) == 1) {
ans = ans + currPowOfN;
}
currPowOfN = currPowOfN * n;
k = k / 2;
}
// Return the result
return ans;
}
// Driver Code
public static void main(String []args)
{
int N = 3;
int K = 4;
System.out.println(FindKthNum(N, K));
}
}
// This Code is contributed by ihritik
Python3
# Python program for the above approach
# Function to find Kth number that can be
# represented as sum of different
# non-negative powers of N
def FindKthNum(n, k):
# To store the ans
ans = 0
# value of current power of N
currPowOfN = 1
# Iterating through bits of K
# from LSB to MSB
while (k > 0):
# If the current bit is 1 then include
# corresponding power of n into ans
if ((k & 1) == 1) :
ans = ans + currPowOfN
currPowOfN = currPowOfN * n
k = k // 2
# Return the result
return ans
# Driver Code
N = 3
K = 4
print(FindKthNum(N, K));
# This Code is contributed by ihritik
C#
// C# program for the above approach
using System;
class GFG
{
// Function to find Kth number that can be
// represented as sum of different
// non-negative powers of N
static int FindKthNum(int n, int k)
{
// To store the ans
int ans = 0;
// Value of current power of N
int currPowOfN = 1;
// Iterating through bits of K
// from LSB to MSB
while (k > 0)
{
// If the current bit is 1 then include
// corresponding power of n into ans
if ((k & 1) == 1) {
ans = ans + currPowOfN;
}
currPowOfN = currPowOfN * n;
k = k / 2;
}
// Return the result
return ans;
}
// Driver Code
public static void Main()
{
int N = 3;
int K = 4;
Console.WriteLine(FindKthNum(N, K));
}
}
// This Code is contributed by ihritik
Javascript
输出:
9
时间复杂度: O(log 2 K)
空间复杂度: O(1)