在 2D 网格中获取隐藏单元所需的最小提示数
给定一个大小为M * N的二维数组。任务是找到在网格上选择隐藏单元格的正确位置所需的最小提示数,在每个提示中,将通知隐藏单元格与所选单元格的曼哈顿距离。
注意:两个单元格之间的曼哈顿距离是单元格的行和列之间的绝对差之和。
例子:
Input: M = 1, N = 3
Output: 1
Explanation: For any hidden cell , if X-path distance from (1,1) is known, then hidden cell is (1, 1+X).
Input: M = 1, N = 1
Output: 0
Explanation: Only one possible cell.
方法:有三种可能的情况来找到网格上单元格的位置:
案例 1:当网格为行形式时,即维度 = (1 x N)(1,1) (1,2) (1,3) (1,4) . . . (1,N)
对于上表,至少需要一个提示。在提示中,可以获得与单元格(1, 1)的距离,如果X是隐藏单元格与(1, 1)的距离,则(1, 1+X )将是隐藏单元格。
案例 2:当网格是列形式时,即维度 = (N x 1)(1,1) (2,1) (3,1) .
.
.(4,1)
对于上表,至少需要一个提示。在提示中,可以获得到(1, 1)的距离,如果X是隐藏单元到(1, 1)的距离,则(1+X, 1)将是隐藏单元。
案例3:当网格为矩形时,即尺寸=(M x N)
对于这种类型的表,至少需要两个提示。所需的提示如下所示:
- 一个获取到单元格(1, 1)的路径距离,如果X是隐藏单元格到(1, 1)的路径距离,则任何形式为(1+X 1 , 1+X 2 )的单元格都将是隐藏的X 1 + X 2 = X的单元格。
- 另一个获取到单元格(1, N)的路径距离,如果Y是隐藏单元格到(1, N)的路径距离,则任何形式为(1+Y 1 , NY 2 )的单元格都将是隐藏单元格其中Y 1 + Y 2 = Y 。
对于 X 和 Y 的任意组合,只有一个单元格同时满足这两个距离。使用上述两个方程可以很容易地找到隐藏的单元格。因此,在这种情况下,至少需要两个帮助。
请按照下图更好地了解具有任何 X 和 Y 值的单元格的条件和交叉点。 X 和 Y 的任何值都将只有一个单元格作为交点。
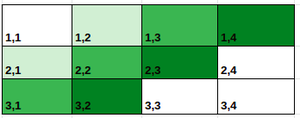
来自 1,1 的等距细胞
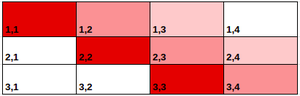
来自 (1,N) 的等距单元,即 1,4
下面是上述方法的实现:
C++
// C++ code to implement above approach
#include
using namespace std;
// Function to find minimum help required
// for finding the hidden cell
int minHints(int M, int N)
{
int res;
// Grid having one cell
if (M == 1 && N == 1)
res = 0;
// Row form or column form grid
else if (M == 1 || N == 1)
res = 1;
// Rectangle form grid
else
res = 2;
return res;
}
int main()
{
// Declaring the dimension of the grid
int M = 1, N = 3;
cout << minHints(M, N);
return 0;
}
Java
// Java code to implement above approach
import java.util.*;
public class GFG
{
// Function to find minimum help required
// for finding the hidden cell
static int minHints(int M, int N)
{
int res;
// Grid having one cell
if (M == 1 && N == 1)
res = 0;
// Row form or column form grid
else if (M == 1 || N == 1)
res = 1;
// Rectangle form grid
else
res = 2;
return res;
}
public static void main(String args[])
{
// Declaring the dimension of the grid
int M = 1, N = 3;
System.out.print(minHints(M, N));
}
}
// This code is contributed by Samim Hossain Mondal.
Python3
# python3 code to implement above approach
# Function to find minimum help required
# for finding the hidden cell
def minHints(M, N):
res = 0
# Grid having one cell
if (M == 1 and N == 1):
res = 0
# Row form or column form grid
elif (M == 1 or N == 1):
res = 1
# Rectangle form grid
else:
res = 2
return res
if __name__ == "__main__":
# Declaring the dimension of the grid
M = 1
N = 3
print(minHints(M, N))
# This code is contributed by rakeshsahni
C#
// C# program for the above approach
using System;
class GFG
{
// Function to find minimum help required
// for finding the hidden cell
static int minHints(int M, int N)
{
int res;
// Grid having one cell
if (M == 1 && N == 1)
res = 0;
// Row form or column form grid
else if (M == 1 || N == 1)
res = 1;
// Rectangle form grid
else
res = 2;
return res;
}
public static void Main()
{
// Declaring the dimension of the grid
int M = 1, N = 3;
Console.Write(minHints(M, N));
}
}
// This code is contributed by Samim Hossain Mondal.
Javascript
1
时间复杂度: O(1)
辅助空间: O(1)