使用水平、垂直和对角线移动最小化到达 Matrix 中的单元格的成本
给定矩阵的两个点P 1 (x 1 , y 1 )和P 2 (x 2 , y 2 ) ,任务是找到从P 1到达P 2的最小成本:
- 任何方向的水平或垂直移动需要 1 个单位
- 任何方向的对角线运动消耗 0 个单位。
例子:
Input: P1 = {7, 4}, P2 = {4, 4}
Output: 1
Explanation: The movements are given below given below:
Movements are (7, 4) -> (6, 5) -> (5, 5) -> (4, 4).
As there is only 1 vertical movement so cost will be 1.
Input: P1 = {1, 2}, P2 = {2, 2}
Output: 1
方法:运动应该使得水平或垂直运动最小。这可以从以下观察中确定:
If the horizontal distance is h = (y2 – y1) and the vertical distance between the points is v = (x2 – x1):
If |h – v| is even, only diagonal movements are enough.
Because horizontal or vertical positions can be maintained with two opposite diagonal movements as shown in the image below:
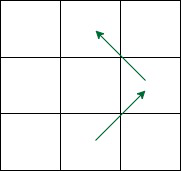
maintaining vertical position
And when horizontal and vertical distances become same, then can directly move to P2 using diagonal movements.
But in case this value is odd then one vertical or horizontal movement is required to make it even.
为此,请执行以下步骤:
- 求从P 1到P 2的垂直距离。假设它是vert 。
- 求从P 1到P 2的水平距离。假设它是hori 。
- 如果|hori – vert| ,总有一条从源到目的地的路,通过对角线移动。甚至。
- 但是如果|hori – vert|是奇数,则需要水平或垂直 1 步之后,仅对角线移动就足够了。
下面是上述方法的实现。
C++
// C++ algorithm for above approach
#include
using namespace std;
// Function to find Minimum Cost
int minCostToExit(int x1, int y1, int x2, int y2)
{
// Finding vertical distance
// from destination
int vert = abs(x2 - x1);
// Finding horizontal distance
// from destination
int hori = abs(y1 - y2);
// Finding the minimum cost
if (abs(hori - vert) % 2 == 0)
return 0;
else
return 1;
}
// Driver code
int main()
{
int x1 = 7;
int y1 = 4;
int x2 = 4, y2 = 4;
int cost = minCostToExit(x1, y1, x2, y2);
cout << cost;
return 0;
}
Java
// Java algorithm for above approach
class GFG {
// Function to find Minimum Cost
static int minCostToExit(int x1, int y1,
int x2, int y2)
{
// Finding vertical distance
// from destination
int vert = Math.abs(x2 - x1);
// Finding horizontal distance
// from destination
int hori = Math.abs(y1 - y2);
// Finding the minimum cost
if (Math.abs(hori - vert) % 2 == 0)
return 0;
else
return 1;
}
// Driver code
public static void main(String args[]) {
int x1 = 7;
int y1 = 4;
int x2 = 4, y2 = 4;
int cost = minCostToExit(x1, y1, x2, y2);
System.out.println(cost);
}
}
// This code is contributed by Saurabh Jaiswal
Python3
# Python algorithm for above approach
import math as Math
# Function to find Minimum Cost
def minCostToExit (x1, y1, x2, y2):
# Finding vertical distance
# from destination
vert = Math.fabs(x2 - x1);
# Finding horizontal distance
# from destination
hori = Math.fabs(y1 - y2);
# Finding the minimum cost
if (Math.fabs(hori - vert) % 2 == 0):
return 0;
else:
return 1;
# Driver code
x1 = 7
y1 = 4
x2 = 4
y2 = 4;
cost = minCostToExit(x1, y1, x2, y2);
print(cost);
# This code is contributed by gfgking
C#
// C# algorithm for above approach
using System;
class GFG {
// Function to find Minimum Cost
static int minCostToExit(int x1, int y1, int x2, int y2)
{
// Finding vertical distance
// from destination
int vert = Math.Abs(x2 - x1);
// Finding horizontal distance
// from destination
int hori = Math.Abs(y1 - y2);
// Finding the minimum cost
if (Math.Abs(hori - vert) % 2 == 0)
return 0;
else
return 1;
}
// Driver code
public static void Main()
{
int x1 = 7;
int y1 = 4;
int x2 = 4, y2 = 4;
int cost = minCostToExit(x1, y1, x2, y2);
Console.Write(cost);
}
}
// This code is contributed by ukasp.
Javascript
1
时间复杂度: O(1)
辅助空间: O(1)