给定数字N,任务是打印宽度为n的小屋。
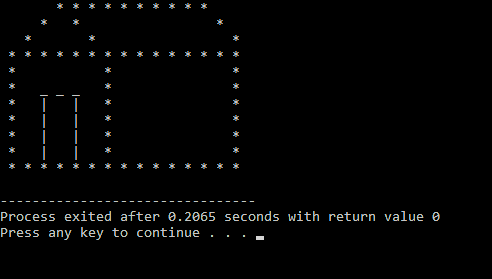
输出
下面是实现上述问题的代码:
程序:
C++
// C++ Program to draw a hut pattern
#include
using namespace std;
// Program to print the Hut
int hut_pattern(int n)
{
int i, j, t;
if (n % 2 == 0) {
n++;
}
for (i = 0; i <= n - n / 3; i++) {
for (j = 0; j < n; j++) {
t = 2 * n / 5;
if (t % 2 != 0) {
t--;
}
if (i == n / 5
|| i == n - n / 3
|| (j == n - 1 && i >= n / 5)
|| (j >= n / 5 && j < n - n / 5 && i == 0)
|| (j == 0 && i >= n / 5)
|| (j == t && i > n / 5)
|| (i <= n / 5 && (i + j == n / 5 || j - i == n / 5))
|| (j - i == n - n / 5)) {
cout << "*";
}
else if (i == n / 5 + n / 7
&& (j >= n / 7 && j <= t - n / 7)) {
cout << "_";
}
else if (i >= n / 5 + n / 7
&& (j == n / 7 || j == t - n / 7)) {
cout << "|";
}
else {
cout << " ";
}
}
cout << "\n";
}
}
// Driver method
int main()
{
// Get the width of the Hut in n
int n = 15;
// Print the Hut
hut_pattern(n);
}
Java
// Java Program to draw a hut pattern
class GFG{
// Program to print the Hut
static void hut_pattern(int n)
{
int i, j, t;
if (n % 2 == 0) {
n++;
}
for (i = 0; i <= n - n / 3; i++) {
for (j = 0; j < n; j++) {
t = 2 * n / 5;
if (t % 2 != 0) {
t--;
}
if (i == n / 5
|| i == n - n / 3
|| (j == n - 1 && i >= n / 5)
|| (j >= n / 5 && j < n - n / 5 && i == 0)
|| (j == 0 && i >= n / 5)
|| (j == t && i > n / 5)
|| (i <= n / 5 && (i + j == n / 5 || j - i == n / 5))
|| (j - i == n - n / 5)) {
System.out.print("*");
}
else if (i == n / 5 + n / 7
&& (j >= n / 7 && j <= t - n / 7)) {
System.out.print("_");
}
else if (i >= n / 5 + n / 7
&& (j == n / 7 || j == t - n / 7)) {
System.out.print("|");
}
else {
System.out.print(" ");
}
}
System.out.print("\n");
}
}
// Driver method
public static void main (String[] args) {
// Get the width of the Hut in n
int n = 15;
// Print the Hut
hut_pattern(n);
}
}
Python3
# Python 3 Program to
# draw a hut pattern
# Program to print the Hut
def hut_pattern(n):
if n % 2 == 0:
n = n+1
for i in range(0, n - n //3 + 1, 1):
for j in range(0, n, 1):
t = 2 * n / 5
if t % 2 != 0:
t = t- 1
if ((i == n / 5) or (i == n - n / 3)
or(j == n - 1 and i >= n / 5) or
(j >= n / 5 and j < n - n / 5 and i == 0)or
(j == 0 and i >= n / 5)or (j == t and i > n / 5) or
(i <= n / 5 and (i + j == n / 5 or j - i == n / 5))or
(j - i == n - n / 5)):
print("*",end = " ")
elif ((i == n // 5 + n // 7) and
(j >= n //7 and j <= t - n // 7)):
print("_",end = " ")
elif ((i >= n // 5 + n // 7) and
(j == n // 7 or j == t - n // 7)):
print("|",end = " ")
else:
print(" ",end = " ")
print("\n");
# Driver method
if __name__ == '__main__':
# Get the width of
# the Hut in n
n = 15
# Print the Hut
hut_pattern(n)
# This code is contributed by
# Surendra_Gangwar
C#
// C# Program to draw a hut pattern
using System;
class GFG
{
// Program to print the Hut
public static void hut_pattern(int n)
{
int i, j, t;
if (n % 2 == 0)
{
n++;
}
for (i = 0; i <= n - n / 3; i++)
{
for (j = 0; j < n; j++)
{
t = 2 * n / 5;
if (t % 2 != 0)
{
t--;
}
if (i == n / 5 || i == n - n / 3 ||
(j == n - 1 && i >= n / 5) ||
(j >= n / 5 && j < n - n / 5 && i == 0) ||
(j == 0 && i >= n / 5) ||
(j == t && i > n / 5) ||
(i <= n / 5 && (i + j == n / 5 ||
j - i == n / 5)) ||
(j - i == n - n / 5))
{
Console.Write("*");
}
else if (i == n / 5 + n / 7 &&
(j >= n / 7 && j <= t - n / 7))
{
Console.Write("_");
}
else if (i >= n / 5 + n / 7 &&
(j == n / 7 || j == t - n / 7))
{
Console.Write("|");
}
else
{
Console.Write(" ");
}
}
Console.Write("\n");
}
}
// Driver Code
static void Main()
{
// Get the width of the Hut in n
int n = 20;
// Print the Hut
hut_pattern(n);
}
}
// This code is contributed by DrRoot_
输出:
**********
* * *
* * *
***************
* * *
* ___ * *
* | | * *
* | | * *
* | | * *
* | | * *
***************
想要从精选的最佳视频中学习和练习问题,请查看《基础知识到高级C的C基础课程》。