给定两个整数D和A,分别代表从原点到直线的垂直距离和垂线与x轴正方向的夹角,任务是找到直线的方程。
例子:
Input: D = 10, A = 30 degrees
Output: 0.87x +0.50y = 10
Input: D = 12, A = 45 degrees
Output: 0.71x +0.71y = 12
方法:根据以下观察可以解决给定的问题:
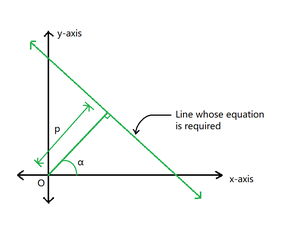
图1
- 设垂直距离为(p) ,垂直线与正 x 轴之间的角度为(α) 度。
- 考虑在所需线上具有坐标(x, y)的点P。
- 从P绘制一条垂线,在L处与 x 轴相交。
- 从L ,在OQ 上在M处画一条垂线。
- 现在,从P画一条垂线,在N处与ML相交。
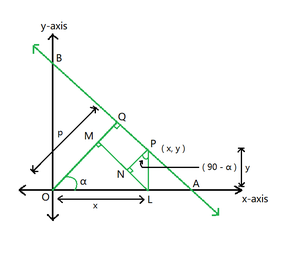
图2
Now consider right triangle OLM — (1)
Now consider right triangle PNL
— (2)
Now
Using equations (1) and (2) which is the equation of the required line
下面是上述方法的实现:
C++
// C++ program for the approach
#include
using namespace std;
// Function to find equation of a line whose
// distance from origin and angle made by the
// perpendicular from origin with x-axis is given
void findLine(int distance, float degree)
{
// Convert angle from degree to radian
float x = degree * 3.14159 / 180;
// Handle the special case
if (degree > 90) {
cout << "Not Possible";
return;
}
// Calculate the sin and cos of angle
float result_1 = sin(x);
float result_2 = cos(x);
// Print the equation of the line
cout << fixed << setprecision(2)
<< result_2 << "x +"
<< result_1 << "y = " << distance;
}
// Driver Code
int main()
{
// Given Input
int D = 10;
float A = 30;
// Function Call
findLine(D, A);
return 0;
}
Java
// Java program for the approach
class GFG{
// Function to find equation of a line whose
// distance from origin and angle made by the
// perpendicular from origin with x-axis is given
static void findLine(int distance, float degree)
{
// Convert angle from degree to radian
float x = (float) (degree * 3.14159 / 180);
// Handle the special case
if (degree > 90) {
System.out.print("Not Possible");
return;
}
// Calculate the sin and cos of angle
float result_1 = (float) Math.sin(x);
float result_2 = (float) Math.cos(x);
// Print the equation of the line
System.out.print(String.format("%.2f",result_2)+ "x +"
+ String.format("%.2f",result_1)+ "y = " + distance);
}
// Driver Code
public static void main(String[] args)
{
// Given Input
int D = 10;
float A = 30;
// Function Call
findLine(D, A);
}
}
// This code is contributed by shikhasingrajput
Python3
# Python3 program for the approach
import math
# Function to find equation of a line whose
# distance from origin and angle made by the
# perpendicular from origin with x-axis is given
def findLine(distance, degree):
# Convert angle from degree to radian
x = degree * 3.14159 / 180
# Handle the special case
if (degree > 90):
print("Not Possible")
return
# Calculate the sin and cos of angle
result_1 = math.sin(x)
result_2 = math.cos(x)
# Print the equation of the line
print('%.2f' % result_2,
"x +", '%.2f' % result_1,
"y = ", distance, sep = "")
# Driver code
# Given Input
D = 10
A = 30
# Function Call
findLine(D, A)
# This code is contributed by mukesh07
C#
// C# program for the approach
using System;
class GFG
{
// Function to find equation of a line whose
// distance from origin and angle made by the
// perpendicular from origin with x-axis is given
static void findLine(int distance, float degree)
{
// Convert angle from degree to radian
float x = (float)(degree * 3.14159 / 180);
// Handle the special case
if (degree > 90) {
Console.WriteLine("Not Possible");
return;
}
// Calculate the sin and cos of angle
float result_1 = (float)(Math.Sin(x));
float result_2 = (float)(Math.Cos(x));
// Print the equation of the line
Console.WriteLine(result_2.ToString("0.00") + "x +"
+ result_1.ToString("0.00") + "y = " + distance);
}
static void Main ()
{
// Given Input
int D = 10;
float A = 30;
// Function Call
findLine(D, A);
}
}
// This code is contributed by suresh07.
Javascript
输出:
0.87x +0.50y = 10
时间复杂度: O(1)
辅助空间: O(1)
如果您希望与专家一起参加现场课程,请参阅DSA 现场工作专业课程和学生竞争性编程现场课程。