Julia 是一种本质上是快速和动态的编程语言(最适合执行数值和科学应用程序),它是可选类型的(描述类型和类型声明的丰富语言,用于巩固我们的程序)和通用和开放-来源。 Julia 像其他编程语言一样支持文件处理,具有更高的效率和相关方法,例如读取、写入和关闭文件。它是 C 语言(性能强大)和Python(简单性)的结合。
创建文件
为了在 Julia 中处理文件,首先我们需要使用“ touch”创建一个新文件(用于创建一个新的空文件) 方法,“ pwd”(用于检查系统当前工作目录) 方法和“ cd” (用于更改目录并在我们想要的位置创建文件)方法。
touch("example.txt")
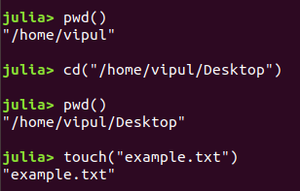
文件已创建
打开现有文件
要在 Julia 中打开文件,我们有“ open ”方法。 open 方法接受两个参数,即文件名(要打开的文件)和操作模式(读、写或追加)。
对于读取模式,我们使用(“r”)、写入模式(“w”)和附加模式(“a”)。
Julia
# here we open file in read mode
abc = open("example.txt","r")
# here we open file in write mode
efg = open("example.txt","w")
# here we open file in append mode
hij = open("example.txt","a")
Julia
open("example.txt") do file
# modify mile content
end
Julia
# METHOD 1
# reading the content of file as a string
mydata = read(abc, String)
# METHOD 2
mydata = open("example.txt") do file
read(file, String)
end
Julia
# open file
mni = open("example.txt","r");
# reading file content line by line
line_by_line = readlines(mni)
Julia
# open file in write mode
abc = open("example.txt", "w")
Julia
# writing to a file using write() method
write(abc, " Hello World , Julia welcomes you")
# We need to close the file in order
# to write the content from the disk to file
close(abc)
Julia
# open file in append mode
abc = open("example.txt", "a")
# writing to a file using write() method
write(abc, " Hello World , Julia welcomes you")
# We need to close the file in order to write the content from the disk to file
close(abc)
Julia
open("example.txt") do abc
# change the case of content
# from uppercase to lowercase
lowercase(read(abc, String))
end
Julia
close(abc) # close method will close the file
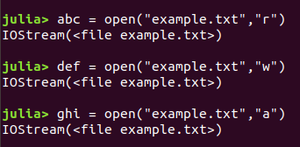
文件以不同模式打开
就像Python一样,Julia 也有一个“ do ” 帮助我们避免操作模式并防止我们每次修改后关闭文件的问题的方法。
朱莉娅
open("example.txt") do file
# modify mile content
end
读取文件内容
读取整个文件
在这里,我们将使用“ read ”方法来读取文件的内容,它接受两个参数,即要读取的文件和读取文件的方法(字符串、整数等)。
朱莉娅
# METHOD 1
# reading the content of file as a string
mydata = read(abc, String)
# METHOD 2
mydata = open("example.txt") do file
read(file, String)
end

文件读取
逐行读取文件
这里我们将使用“ readlines ”方法将文件作为数组准备好,文件的每一行作为数组元素。
朱莉娅
# open file
mni = open("example.txt","r");
# reading file content line by line
line_by_line = readlines(mni)
写入文件
在这里,我们将文件的模式从读取更改为写入,以便在“打开”方法中写入文件。
使用写入模式
朱莉娅
# open file in write mode
abc = open("example.txt", "w")
现在,我们将使用 Julia 中的 write 方法将内容写入“ example.txt ”文件,write 方法将从文件中删除先前的内容,或者如果文件不存在则创建该文件。
朱莉娅
# writing to a file using write() method
write(abc, " Hello World , Julia welcomes you")
# We need to close the file in order
# to write the content from the disk to file
close(abc)
使用追加模式
朱莉娅
# open file in append mode
abc = open("example.txt", "a")
# writing to a file using write() method
write(abc, " Hello World , Julia welcomes you")
# We need to close the file in order to write the content from the disk to file
close(abc)
修改文件内容
在这里,我们将学习修改文件的内容,即我们将使用小写方法将文件内容的大小写从大写更改为小写。
朱莉娅
open("example.txt") do abc
# change the case of content
# from uppercase to lowercase
lowercase(read(abc, String))
end
关闭文件
这里我们将使用 Julia 中的 close 方法在修改其内容后关闭文件。
朱莉娅
close(abc) # close method will close the file