使用 React 和 NeumorphismUI 设计一个脑力游戏
脑筋急转弯游戏旨在找出一个人可以尽快回答多少个问题。我们将设计一个带有 NeumorphismUI 的 React 应用程序,它将显示一些关于一些常见事实的有趣问题,并且用户必须尽快回答这些问题。
React 是一个用于构建用户界面的声明式、高效且灵活的 JavaScript 库。它是 MVC 中的“V”。 ReactJS 是一个开源的、基于组件的前端库,只负责应用程序的视图层。它由 Facebook 维护。
React 使用声明式范式,可以更轻松地推理您的应用程序,并旨在高效和灵活。它为应用程序中的每个状态设计简单的视图,当你的数据发生变化时,React 将有效地更新和呈现正确的组件。声明式视图使您的代码更可预测且更易于调试。
为什么要使用 NeumorphismUI?
NeumorphismUI 是一种用于设计 Web 元素、框架和屏幕的现代软 UI,也是一种相对较新的设计趋势,近来非常受欢迎。它的美学以极简和真实的 UI 为标志,这是对旧的 SkeuomorphismUI 设计趋势的重新推进。因此,为了使我们的游戏更加用户友好和有吸引力,我们将在我们的项目中使用它。
此游戏显示一组必须尽快回答的问题,并相应地显示分数。回答错误或输入错误的答案将导致负分。
我们将首先创建 React 应用程序,并使用一些 CSS 样式在其中添加一些逻辑,以赋予它一种柔和的感觉。
我们正在建造什么?
下面只是我们在这里构建的内容的一瞥。
现在我们将编写一些 JSX 代码来构建我们的 Web 应用程序。
JSX 布局: JSX 布局定义了将在页面上显示的元素结构。这包括
- 游戏主容器:此部分包含我们的游戏标题和游戏容器。
- 游戏容器:此部分包含游戏组件,其中包含整个游戏逻辑。
- 游戏组件:该组件包含游戏分数、问题、错误和成功信息、输入区域、重置和进入按钮。
- 游戏错误和成功消息:如果用户输入错误答案或尝试在输入区域为空的情况下提交答案,则会显示错误消息,而如果用户输入正确答案,则会显示成功消息。
- 输入区:此部分包含必须输入答案的输入区。
- 重置和输入按钮:如果用户想跳到另一个问题,可以单击重置按钮,也可以单击输入按钮提交答案。
执行:
第 1 步:使用以下命令创建一个 React 应用程序:
npx create-react-app Brain-Teasing-Game
第 2 步:创建项目文件夹后,即 Brain-Teasing-Game,使用以下命令移动到该文件夹:
cd Brain-Teasing-Game
项目结构:它将如下所示。
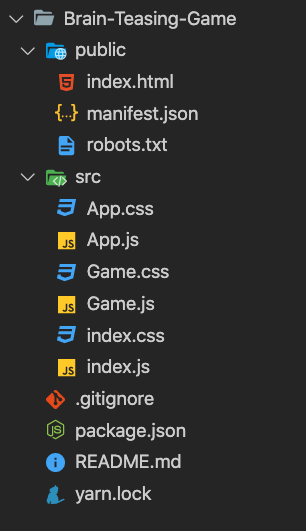
项目结构
文件名-App.js:
Javascript
import React from 'react';
import app from './App.css';
import Game from './Game.js';
function App() {
// Creating the container and importing
// Game component
return (
Welcome to the Brain Twister game
);
}
export default App;
Javascript
import React, { useEffect, useState } from 'react';
import game from './Game.css';
const Game = () => {
// Initialising states with default values
const [word, setWord] = useState('');
const [value, setValue] = useState('');
const [err, setErr] = useState('');
const [success, setSuccess] = useState('');
const [score, setScore] = useState(0);
const [question, setQuestion] = useState('');
// Starts generating words as soon as
// the component is placed in the DOM Tree
useEffect(() => {
wordGenerate();
}, []);
// Defining questions with their
// corresponding answers
const QUESTION = [];
const ANSWERS = [];
// Generating Random questions and storing
// their answers in the state variable
const wordGenerate = () => {};
// checking if the typed answer is correct or not
const check = () => {};
// defining function to skip
// to another question
const reset = () => {};
// Writing the JSX Code
return (
SCORE : {score}
{question ? (
Question: {question}
) : null}
{err ? {err}
: null}
{success ? {success}
: null}
setValue(e.target.value)}
placeholder="Enter Answer Here!"
className={game.Game__input_answer}
/>
);
};
export default Game;
Javascript
const QUESTION = [
'Greatest Computer Science portal for geeks',
'Full form of DSA',
'Most Used Web Framework',
'Founder of GeeksForGeeks',
'Who Created Facebook',
'CEO of Tesla and SpaceX',
'When 5G going to launch in India',
];
const ANSWERS = [
'GeeksForGeeks',
'Data Structures and algorithms',
'React',
'Sandeep Jain',
'Mark Zuckerberg',
'Elon Musk',
'2021',
];
Javascript
const wordGenerate = () => {
const randomNumber = Math.floor(Math.random() * ANSWERS.length);
setWord(ANSWERS[randomNumber]);
setQuestion(QUESTION[randomNumber]);
};
Javascript
const check = () => {
if (value) {
if (value.toLowerCase() === word.toLowerCase()) {
setValue('');
setErr(null);
setSuccess('Correct Answer');
setScore((prevScore) => prevScore + 1);
wordGenerate();
} else {
setSuccess(null);
setErr('Wrong Answer');
setScore((prevScore) => prevScore - 1);
setValue('');
wordGenerate();
}
} else {
setSuccess(null);
setErr('Please enter the value!');
}
};
Javascript
const reset = () => {
setValue(null);
setSuccess(null);
setErr(null);
wordGenerate();
};
Javascript
import React, { useEffect, useState } from 'react';
import game from './Game.css';
const Game = () => {
// Initialising states with default values
const [word, setWord] = useState('');
const [value, setValue] = useState('');
const [err, setErr] = useState('');
const [success, setSuccess] = useState('');
const [score, setScore] = useState(0);
const [question, setQuestion] = useState('');
// Starts generating words as soon as
// the component is placed in the DOM Tree
useEffect(() => {
wordGenerate();
}, []);
// Defining questions with their
// corresponding answers
const QUESTION = [
'Greatest Computer Science portal for geeks',
'Full form of DSA',
'Most Used Web Framework',
'Founder of GeeksForGeeks',
'Who Created Facebook',
'CEO of Tesla and SpaceX',
'When 5G going to launch in India',
];
const ANSWERS = [
'GeeksForGeeks',
'Data Structures and algorithms',
'React',
'Sandeep Jain',
'Mark Zuckerberg',
'Elon Musk',
'2021',
];
// Generating Random questions and storing
// their answers in the state variable
const wordGenerate = () => {
const randomNumber = Math.floor(Math.random() * ANSWERS.length);
setWord(ANSWERS[randomNumber]);
setQuestion(QUESTION[randomNumber]);
};
// checking if the typed answer is correct or not
const check = () => {
if (value) {
if (value.toLowerCase() === word.toLowerCase()) {
setValue('');
setErr(null);
setSuccess('Correct Answer');
setScore((prevScore) => prevScore + 1);
wordGenerate();
} else {
setSuccess(null);
setErr('Wrong Answer');
setScore((prevScore) => prevScore - 1);
setValue('');
wordGenerate();
}
} else {
setSuccess(null);
setErr('Please enter the value!');
}
};
// defining function to skip
// to another question
const reset = () => {
setValue(null);
setSuccess(null);
setErr(null);
wordGenerate();
};
// Writing the JSX Code
return (
SCORE : {score}
{question ? (
Question: {question}
) : null}
{err ? {err}
: null}
{success ? {success}
: null}
setValue(e.target.value)}
placeholder="Enter Answer Here!"
className={game.Game__input_answer}
/>
);
};
export default Game;
CSS
.Game__main_container {
width: 40%;
height: 50%;
margin: 100px auto;
border-radius: 3rem;
box-shadow: 0.8rem 0.8rem 1.4rem #c8d0e7eb, -0.2rem -0.2rem 1.8rem #fff;
padding: 4rem;
}
.Game__heading{
box-shadow: 0.3rem 0.3rem 0.6rem #c8d0e7eb, -0.2rem -0.2rem 0.5rem #fff,
inset 0.2rem 0.2rem 0.5rem #c8d0e7, inset -0.2rem -0.2rem 0.5rem #fff;
border-radius: 10px;
padding: 10px;
text-align: center;
color: #9baacf;
}
Filename: Game.css
.Game__score { text-align: right; color: #6d5dfc; font-weight: 700; display: inline-block; width:100%; } .Game__error, .Game__success { color: red; border-radius: 10px; padding: 20px; margin: 10px 0; background-color: rgba(255, 0, 0, 0.207); } .Game__success { color: #fff; background-color: rgba(0, 128, 0, 0.399); } .Game__question { color: #1a1a1a; font-size: 20px; } .Game__input_answer { width: 20.4rem; height: 3rem; display: block; border: none; border-radius: 1rem; font-size: 1.4rem; padding-left: 1.4rem; box-shadow: inset 0.2rem 0.2rem 0.5rem #c8d0e7, inset -0.2rem -0.2rem 0.5rem #fff; background: none; color: #9baacf; margin: 50px auto; outline: none; } .Game__input_answer input::placeholder { color: #bec8e482; } input:focus { box-shadow: 0.3rem 0.3rem 0.6rem #c8d0e7eb, -0.2rem -0.2rem 0.5rem #fff; } .Game__start, .Game__submit { width: 45%; height: 4rem; font-size: 20px; margin: 10px; border-radius: 1rem; box-shadow: 0.3rem 0.3rem 0.6rem #c8d0e7, -0.2rem -0.2rem 0.5rem #fff; justify-self: center; display: inline; outline: none; border: none; cursor: pointer; } .Game__start { background: #6d5dfc; box-shadow: inset 0.2rem 0.2rem 1rem #8abdff, inset -0.2rem -0.2rem 1rem #5b0eeb, 0.3rem 0.3rem 0.6rem #c8d0e7, -0.2rem -0.2rem 0.5rem #fff; color: #e4ebf5; }
文件名-Game.js:
Javascript
import React, { useEffect, useState } from 'react';
import game from './Game.css';
const Game = () => {
// Initialising states with default values
const [word, setWord] = useState('');
const [value, setValue] = useState('');
const [err, setErr] = useState('');
const [success, setSuccess] = useState('');
const [score, setScore] = useState(0);
const [question, setQuestion] = useState('');
// Starts generating words as soon as
// the component is placed in the DOM Tree
useEffect(() => {
wordGenerate();
}, []);
// Defining questions with their
// corresponding answers
const QUESTION = [];
const ANSWERS = [];
// Generating Random questions and storing
// their answers in the state variable
const wordGenerate = () => {};
// checking if the typed answer is correct or not
const check = () => {};
// defining function to skip
// to another question
const reset = () => {};
// Writing the JSX Code
return (
SCORE : {score}
{question ? (
Question: {question}
) : null}
{err ? {err}
: null}
{success ? {success}
: null}
setValue(e.target.value)}
placeholder="Enter Answer Here!"
className={game.Game__input_answer}
/>
);
};
export default Game;
现在,我们的应用程序的基本结构已经创建,现在是时候通过在游戏组件中定义初始化函数来添加一些逻辑了。
游戏的主要逻辑:游戏的主要逻辑在已经初始化的函数中定义。有几个函数协同工作来运行游戏。
第 1 步:写下问题及其相应的答案
脑筋急转弯游戏的问题和答案将存储在初始化的数组中。
Javascript
const QUESTION = [ 'Greatest Computer Science portal for geeks', 'Full form of DSA', 'Most Used Web Framework', 'Founder of GeeksForGeeks', 'Who Created Facebook', 'CEO of Tesla and SpaceX', 'When 5G going to launch in India', ]; const ANSWERS = [ 'GeeksForGeeks', 'Data Structures and algorithms', 'React', 'Sandeep Jain', 'Mark Zuckerberg', 'Elon Musk', '2021', ];
第 2 步:生成随机问题
当 Game 组件被放置在 DOM 树中或当用户单击进入或重置按钮时,将调用函数wordGenerate()。
我们将生成一个 0 到 ANSWERS 数组长度范围内的随机数,将其存储为变量,然后使用它来挑选随机问题及其答案并将它们存储在状态变量中。
Javascript
const wordGenerate = () => { const randomNumber = Math.floor(Math.random() * ANSWERS.length); setWord(ANSWERS[randomNumber]); setQuestion(QUESTION[randomNumber]); };
第三步:检查输入的答案是否正确
我们这里有两种情况:
- 用户未在输入区域输入任何值:
- 如果键入的值为 null 则将显示错误消息
- 用户输入了一些值:
- 将输入的值转换为小写,将其与存储的答案进行比较,然后相应地显示错误或成功消息
Javascript
const check = () => { if (value) { if (value.toLowerCase() === word.toLowerCase()) { setValue(''); setErr(null); setSuccess('Correct Answer'); setScore((prevScore) => prevScore + 1); wordGenerate(); } else { setSuccess(null); setErr('Wrong Answer'); setScore((prevScore) => prevScore - 1); setValue(''); wordGenerate(); } } else { setSuccess(null); setErr('Please enter the value!'); } };
- 用户未在输入区域输入任何值:
第 4 步:向重置按钮处理程序添加逻辑
单击重置按钮时,删除所有错误消息并生成一个新问题。
Javascript
const reset = () => { setValue(null); setSuccess(null); setErr(null); wordGenerate(); };
Filename-Game.js:在我们的脑筋急转弯游戏中添加完所有的逻辑后, Game.js最终的样子如下:
Javascript
import React, { useEffect, useState } from 'react';
import game from './Game.css';
const Game = () => {
// Initialising states with default values
const [word, setWord] = useState('');
const [value, setValue] = useState('');
const [err, setErr] = useState('');
const [success, setSuccess] = useState('');
const [score, setScore] = useState(0);
const [question, setQuestion] = useState('');
// Starts generating words as soon as
// the component is placed in the DOM Tree
useEffect(() => {
wordGenerate();
}, []);
// Defining questions with their
// corresponding answers
const QUESTION = [
'Greatest Computer Science portal for geeks',
'Full form of DSA',
'Most Used Web Framework',
'Founder of GeeksForGeeks',
'Who Created Facebook',
'CEO of Tesla and SpaceX',
'When 5G going to launch in India',
];
const ANSWERS = [
'GeeksForGeeks',
'Data Structures and algorithms',
'React',
'Sandeep Jain',
'Mark Zuckerberg',
'Elon Musk',
'2021',
];
// Generating Random questions and storing
// their answers in the state variable
const wordGenerate = () => {
const randomNumber = Math.floor(Math.random() * ANSWERS.length);
setWord(ANSWERS[randomNumber]);
setQuestion(QUESTION[randomNumber]);
};
// checking if the typed answer is correct or not
const check = () => {
if (value) {
if (value.toLowerCase() === word.toLowerCase()) {
setValue('');
setErr(null);
setSuccess('Correct Answer');
setScore((prevScore) => prevScore + 1);
wordGenerate();
} else {
setSuccess(null);
setErr('Wrong Answer');
setScore((prevScore) => prevScore - 1);
setValue('');
wordGenerate();
}
} else {
setSuccess(null);
setErr('Please enter the value!');
}
};
// defining function to skip
// to another question
const reset = () => {
setValue(null);
setSuccess(null);
setErr(null);
wordGenerate();
};
// Writing the JSX Code
return (
SCORE : {score}
{question ? (
Question: {question}
) : null}
{err ? {err}
: null}
{success ? {success}
: null}
setValue(e.target.value)}
placeholder="Enter Answer Here!"
className={game.Game__input_answer}
/>
);
};
export default Game;
现在将所有逻辑添加到游戏中,我们将为其添加一些 CSS 样式,以赋予它更柔和的 UI 感觉。
CSS 样式: CSS 用于设置不同部分的样式并使它们更具视觉吸引力。
- 为每个元素提供足够的填充和边距。
- 每个元素的文本大小使得用户在玩游戏时易于阅读。
- index.css 文件中还提供了背景颜色#e4ebf5e0
- 代码:
文件名:App.css
CSS
.Game__main_container {
width: 40%;
height: 50%;
margin: 100px auto;
border-radius: 3rem;
box-shadow: 0.8rem 0.8rem 1.4rem #c8d0e7eb, -0.2rem -0.2rem 1.8rem #fff;
padding: 4rem;
}
.Game__heading{
box-shadow: 0.3rem 0.3rem 0.6rem #c8d0e7eb, -0.2rem -0.2rem 0.5rem #fff,
inset 0.2rem 0.2rem 0.5rem #c8d0e7, inset -0.2rem -0.2rem 0.5rem #fff;
border-radius: 10px;
padding: 10px;
text-align: center;
color: #9baacf;
}
Filename: Game.css
.Game__score { text-align: right; color: #6d5dfc; font-weight: 700; display: inline-block; width:100%; } .Game__error, .Game__success { color: red; border-radius: 10px; padding: 20px; margin: 10px 0; background-color: rgba(255, 0, 0, 0.207); } .Game__success { color: #fff; background-color: rgba(0, 128, 0, 0.399); } .Game__question { color: #1a1a1a; font-size: 20px; } .Game__input_answer { width: 20.4rem; height: 3rem; display: block; border: none; border-radius: 1rem; font-size: 1.4rem; padding-left: 1.4rem; box-shadow: inset 0.2rem 0.2rem 0.5rem #c8d0e7, inset -0.2rem -0.2rem 0.5rem #fff; background: none; color: #9baacf; margin: 50px auto; outline: none; } .Game__input_answer input::placeholder { color: #bec8e482; } input:focus { box-shadow: 0.3rem 0.3rem 0.6rem #c8d0e7eb, -0.2rem -0.2rem 0.5rem #fff; } .Game__start, .Game__submit { width: 45%; height: 4rem; font-size: 20px; margin: 10px; border-radius: 1rem; box-shadow: 0.3rem 0.3rem 0.6rem #c8d0e7, -0.2rem -0.2rem 0.5rem #fff; justify-self: center; display: inline; outline: none; border: none; cursor: pointer; } .Game__start { background: #6d5dfc; box-shadow: inset 0.2rem 0.2rem 1rem #8abdff, inset -0.2rem -0.2rem 1rem #5b0eeb, 0.3rem 0.3rem 0.6rem #c8d0e7, -0.2rem -0.2rem 0.5rem #fff; color: #e4ebf5; }
运行应用程序的步骤:从项目的根目录使用以下命令运行应用程序:
npm start
输出:游戏现在可以在任何浏览器中玩了。
源代码: https://github.com/RahulBansal123/Brain-Teasing-Game