Flutter振——TextOverFlow
Text 可能会从容器、卡片等小部件中溢出。Text Widget 有一个属性overflow来处理 android、IOS、WEB、桌面应用程序中的溢出文本。溢出属性有多个参数,如剪辑、省略号、淡入淡出、可见等。每个参数都有不同的函数来处理文本。因此,在本文中,我们将介绍所有 TextOverFlow 参数。
TextOverFlow 参数
省略号:使用省略号 (...) 表示文本溢出。
Code: Text(
'Wanted Text',
overflow: TextOverflow.ellipsis,
),
淡入淡出:溢出的文本显示为透明。
Code: Text(
'Wanted Text',
overflow: TextOverflow.ellipsis,
),
Visible :在容器外渲染文本。
Code: Text(
'Wanted Text',
overflow: TextOverflow.visible,
),
Clip :剪辑溢出的文本以修复其容器。
Code: Text(
'Wanted Text',
overflow: TextOverflow.ellipsis,
),
以下实施:
创建一个项目并导入材料包。
Dart
import 'package:flutter/material.dart';
Dart
void main(){
runApp(TextHome());
}
Dart
class TextHome extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp();
}
Dart
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('textOverFlow Example')
),
body: Column(
children :[
Container(
padding: EdgeInsets.fromLTRB(20, 20, 20, 0),
child :Text(
"This is example of textoverflow ecllipsis, In the end there are the dots",
style: TextStyle(fontSize: 22),
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.center,
)),
])
)
);
}
Dart
import 'package:flutter/material.dart';
void main() {
runApp(const TextHome());
}
class TextHome extends StatelessWidget {
const TextHome({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
title: const Text("TextOverflow"),
),
body: Column(
children: [
Text("Ellipsis Example"),
Container(
padding: const EdgeInsets.all(10),
child: const Text(
"This is the example of TextOverflow ellipsis, In the end there are the dots",
overflow: TextOverflow.ellipsis,
),
),
Divider(),
Text("fade Example"),
Container(
width: 500,
height: 50,
padding: const EdgeInsets.all(10),
child: const Text(
"This is the example of TextOverflow fade, In the end text is faded",
overflow: TextOverflow.fade,
),
),
Divider(),
Text("clip Example"),
Container(
width: 500,
height: 55,
padding: const EdgeInsets.all(10),
child: const Text(
"This is the example of TextOverflow clip, This will clip the text",
overflow: TextOverflow.clip,
),
),
Divider(),
Text("visible Example"),
Container(
width: 500,
height: 50,
padding: const EdgeInsets.all(10),
child: const Text(
"This is the example of TextOverflow visible, Text is visible",
overflow: TextOverflow.visible,
),
),
],
),
),
);
}
}
现在在void main()函数中调用runApp()方法并调用类TextHome() 。
Dart
void main(){
runApp(TextHome());
}
现在创建一个名为TextHome()的新类,这将是一个无状态类,因为我们的应用程序在运行时不会改变它的状态。并返回MaterialApp()。
Dart
class TextHome extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp();
}
在脚手架的属性主体中创建一个列小部件。创建一个具有子属性Text( )的容器小部件。在溢出属性中给出省略号。
Dart
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('textOverFlow Example')
),
body: Column(
children :[
Container(
padding: EdgeInsets.fromLTRB(20, 20, 20, 0),
child :Text(
"This is example of textoverflow ecllipsis, In the end there are the dots",
style: TextStyle(fontSize: 22),
overflow: TextOverflow.ellipsis,
textAlign: TextAlign.center,
)),
])
)
);
}
您只需更改 TextOverflow 参数即可为所有其他人执行此操作。对于所有溢出参数,Final TextHome类将是这样的。
Dart
import 'package:flutter/material.dart';
void main() {
runApp(const TextHome());
}
class TextHome extends StatelessWidget {
const TextHome({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
title: const Text("TextOverflow"),
),
body: Column(
children: [
Text("Ellipsis Example"),
Container(
padding: const EdgeInsets.all(10),
child: const Text(
"This is the example of TextOverflow ellipsis, In the end there are the dots",
overflow: TextOverflow.ellipsis,
),
),
Divider(),
Text("fade Example"),
Container(
width: 500,
height: 50,
padding: const EdgeInsets.all(10),
child: const Text(
"This is the example of TextOverflow fade, In the end text is faded",
overflow: TextOverflow.fade,
),
),
Divider(),
Text("clip Example"),
Container(
width: 500,
height: 55,
padding: const EdgeInsets.all(10),
child: const Text(
"This is the example of TextOverflow clip, This will clip the text",
overflow: TextOverflow.clip,
),
),
Divider(),
Text("visible Example"),
Container(
width: 500,
height: 50,
padding: const EdgeInsets.all(10),
child: const Text(
"This is the example of TextOverflow visible, Text is visible",
overflow: TextOverflow.visible,
),
),
],
),
),
);
}
}
解释:
- Main 是在加载的程序上运行的主要方法。
- 加载后, TextHome类为 Run。
- TextHome 类是一个无状态的 Widget,因为我们只需要显示溢出的文本。
- 由于Flutter基于小部件,我们必须创建一个。
- MaterialApp 允许我们设置标题和主题。
- MaterialApp 作为家庭脚手架。
- Scaffold 允许我们设置 AapBar 和页面的正文。
- 作为一个 AapBar 是一个标题。
- 作为一个主体,它需要一列。
- Column 采用容器小部件。
- 容器接受我们将应用溢出的子文本。
- 文本首先取我们要显示的值,然后应用溢出。
输出:
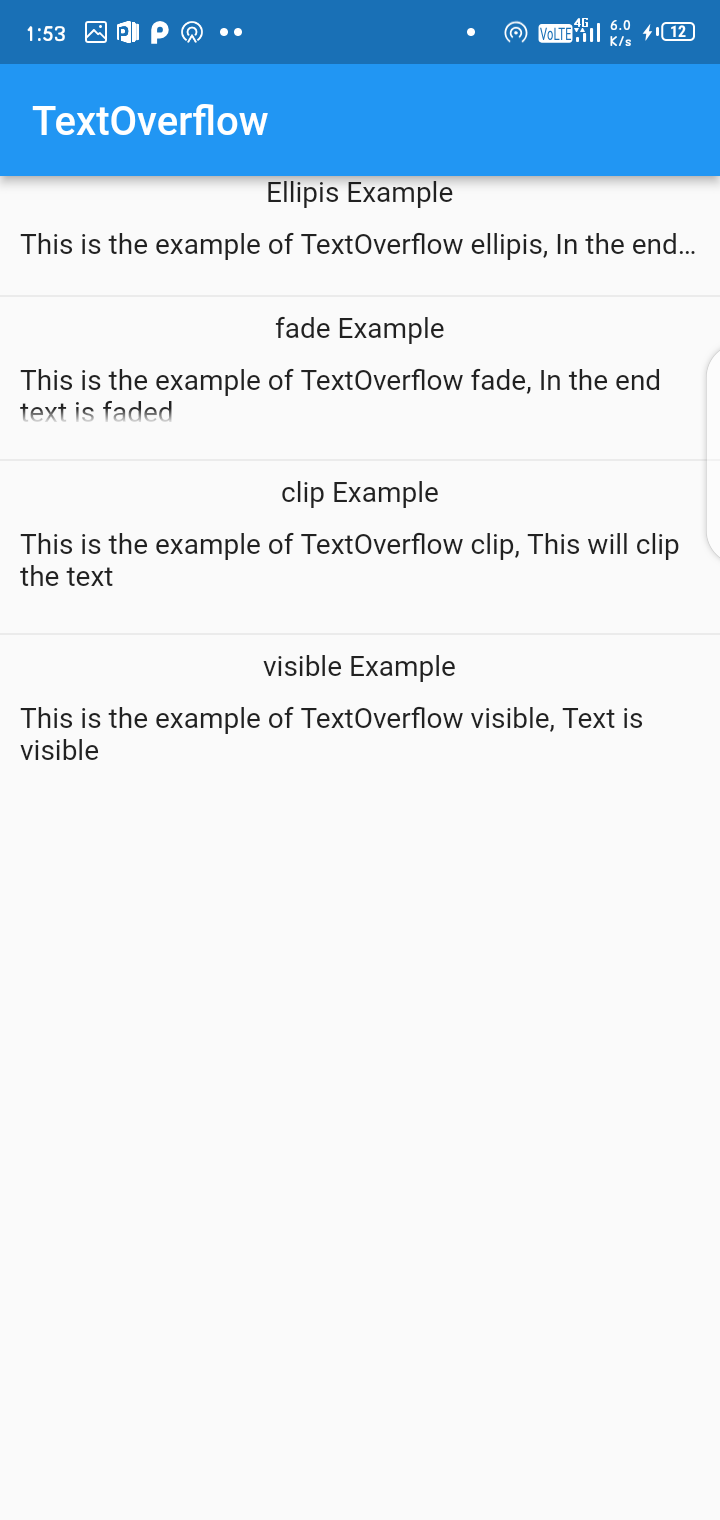