使用Python修改 PDF 文件
下面的文章描述了如何使用 python 的 pylovepdf 模块修改 PDF。便携式文档格式 (PDF) 是 Adobe 于 1993 年开发的一种文件格式,用于以独立于应用软件、硬件和操作系统的方式呈现文档,包括文本格式和图像。
pylovepdf 模块可以使用 pip 命令下载:
pip install pylovepdf
iLovePDF API 即'pylovepdf' 模块是围绕REST 组织的。他们的 API 是可预测的、面向资源的 URL,并使用 HTTP 响应代码来指示 API 错误。它们使用内置的 HTTP 功能,如 HTTP 身份验证和 HTTP 动词,现成的 HTTP 客户端可以理解这些功能。它们支持跨源资源共享,允许您从客户端 Web 应用程序安全地与其 API 交互。使用这个 API,我们可以压缩 PDF 文件,也可以添加水印,将它们转换为图像,甚至拆分它们,反之亦然以及许多其他东西。
首先,我们需要一个公钥来使用此模块,以便登录 https://developer.ilovepdf.com/,登录后,公钥将在“我的项目”部分中可见。下面是公钥的截图
现在,由于我们有了公钥,我们可以使用此 API 使用以下步骤修改任何 PDF 文件:
- 1.使用公钥创建一个ILovePdf对象
- 2.上传PDF文件
- 3.处理PDF文件
- 4. 下载PDF文件
使用示例正确描述了该模块的实现。单击此处获取本文提供的示例中使用的 PDF:
示例 1:压缩 PDF 文件
Python3
# importing the ilovepdf api
from pylovepdf.ilovepdf import ILovePdf
# public key
public_key = 'paste_your_public_key_here'
# creating a ILovePdf object
ilovepdf = ILovePdf(public_key, verify_ssl=True)
# assigning a new compress task
task = ilovepdf.new_task('compress')
# adding the pdf file to the task
task.add_file('my_pdf.pdf')
# setting the output folder directory
# if no folder exist it will create one
task.set_output_folder('output_folder')
# execute the task
task.execute()
# download the task
task.download()
# delete the task
task.delete_current_task()
Python
# public key
from pylovepdf.ilovepdf import ILovePdf
public_key = 'paste your code here'
# importing the ilovepdf api
# creating a ILovePdf object
ilovepdf = ILovePdf(public_key, verify_ssl=True)
# assigning a new split task task
task = ilovepdf.new_task('split')
# adding the pdf file to the task
task.add_file('my_pdf.pdf')
# setting the output folder directory
# if no folder exist it will create one
task.set_output_folder('output_folder')
# execute the task
task.execute()
# download the task
task.download()
# delete the task
task.delete_current_task()
处理前:
输出 :
处理后:
示例 2:拆分 PDF
Python
# public key
from pylovepdf.ilovepdf import ILovePdf
public_key = 'paste your code here'
# importing the ilovepdf api
# creating a ILovePdf object
ilovepdf = ILovePdf(public_key, verify_ssl=True)
# assigning a new split task task
task = ilovepdf.new_task('split')
# adding the pdf file to the task
task.add_file('my_pdf.pdf')
# setting the output folder directory
# if no folder exist it will create one
task.set_output_folder('output_folder')
# execute the task
task.execute()
# download the task
task.download()
# delete the task
task.delete_current_task()
输出 :
处理后:
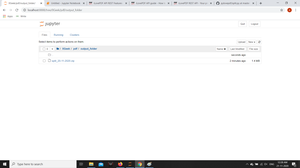
压缩