多级链表
多级链表
多级链表是一种二维数据结构,由多个链表组成,多级链表中的每个节点都有一个 next 和 child 指针。所有元素都使用指针链接。
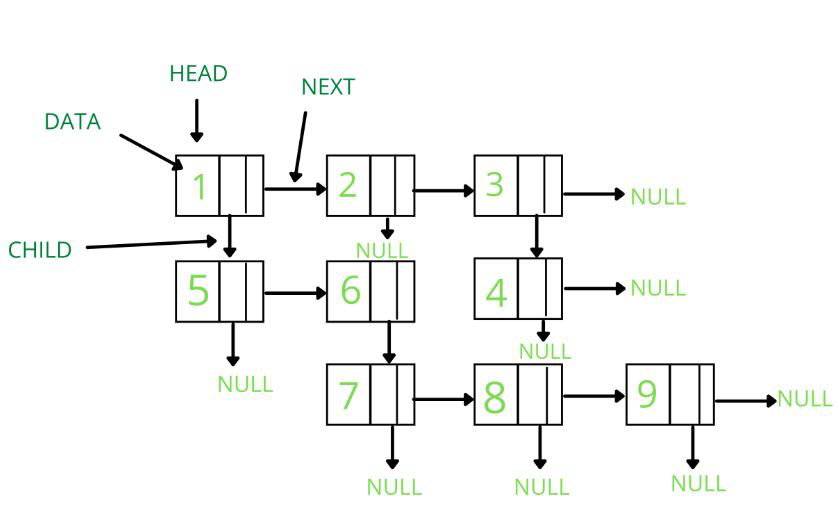
多级链表
表示:
多级链表由指向链表第一个节点的指针表示。与链表类似,第一个节点称为头。如果多级链表为空,则 head 的值为 NULL。列表中的每个节点至少包含三个部分:
1.数据。
2.指向下一个节点的指针。
3.指向子节点的指针。
多级链表的每个节点表示为:
class Node
{
public:
int data;
Node *next;
Node *child;
};
下面是多级链表的实现
C++
// C++ program to implement
// a multilevel linked list
#include
using namespace std;
// Representation of node
class Node {
public:
int data;
Node* next;
Node* child;
};
// A function to create a linked list
// with n(size) nodes returns head pointer
Node* createList(int* arr, int n)
{
Node* head = NULL;
Node* tmp;
// Traversing the passed array
for (int i = 0; i < n; i++) {
// Creating a node if the list
// is empty
if (head == NULL) {
tmp = head = new Node();
}
else {
tmp->next = new Node();
tmp = tmp->next;
}
// Created a node with data and
// setting child and next pointer
// as NULL.
tmp->data = arr[i];
tmp->next = tmp->child = NULL;
}
return head;
}
// To print the linked list
void printMultiLevelList(Node* head)
{
// While head is not null
while (head) {
if (head->child) {
printMultiLevelList(head->child);
}
cout << head->data << " ";
head = head->next;
}
}
// Driver code
int main()
{
// Initializing the data in arrays(row wise)
int arr1[3] = { 1, 2, 3 };
int arr2[2] = { 5, 6 };
int arr3[1] = { 4 };
int arr4[3] = { 7, 8, 9 };
// creating Four linked lists
// Passing array and size of array
// as parameters
Node* head1 = createList(arr1, 3);
Node* head2 = createList(arr2, 2);
Node* head3 = createList(arr3, 1);
Node* head4 = createList(arr4, 3);
// Initializing children and next pointers
// as shown in given diagram
head1->child = head2;
head1->next->next->child = head3;
head2->next->child = head4;
// Creating a null pointer.
Node* head = NULL;
head = head1;
// Function Call to print
printMultiLevelList(head);
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
public static class Node {
int data;
Node next;
Node child;
};
// A function to create a linked list
// with n(size) nodes returns head pointer
public static Node createList(int arr[], int n)
{
Node head = null;
Node tmp = null;
// Traversing the passed array
for (int i = 0; i < n; i++)
{
// Creating a node if the list
// is empty
if (head == null) {
tmp = head = new Node();
}
else {
tmp.next = new Node();
tmp = tmp.next;
}
// Created a node with data and
// setting child and next pointer
// as NULL.
tmp.data = arr[i];
tmp.next = tmp.child = null;
}
return head;
}
// To print the linked list
public static void printMultiLevelList(Node head)
{
// While head is not null
while (head != null) {
if (head.child != null) {
printMultiLevelList(head.child);
}
System.out.print(head.data + " ");
head = head.next;
}
}
// Driver code
public static void main(String[] args)
{
int arr1[] = { 1, 2, 3 };
int arr2[] = { 5, 6 };
int arr3[] = { 4 };
int arr4[] = { 7, 8, 9 };
// creating Four linked lists
// Passing array and size of array
// as parameters
Node head1 = createList(arr1, 3);
Node head2 = createList(arr2, 2);
Node head3 = createList(arr3, 1);
Node head4 = createList(arr4, 3);
// Initializing children and next pointers
// as shown in given diagram
head1.child = head2;
head1.next.next.child = head3;
head2.next.child = head4;
// Creating a null pointer.
Node head = null;
head = head1;
// Function Call to print
printMultiLevelList(head);
}
}
// This code is contributed by maddler.
Python3
'''package whatever #do not write package name here '''
class Node:
def __init__(self):
self.data = 0;
self.next = None;
self.child = None;
# A function to create a linked list
# with n(size) Nodes returns head pointer
def createList(arr, n):
head = None;
tmp = None;
# Traversing the passed array
for i in range(n):
# Creating a Node if the list
# is empty
if (head == None):
tmp = head = Node();
else:
tmp.next = Node();
tmp = tmp.next;
# Created a Node with data and
# setting child and next pointer
# as NULL.
tmp.data = arr[i];
tmp.next = tmp.child = None;
return head;
# To print linked list
def printMultiLevelList(head):
# While head is not None
while (head != None):
if (head.child != None):
printMultiLevelList(head.child);
print(head.data, end=" ");
head = head.next;
# Driver code
if __name__ == '__main__':
arr1 = [ 1, 2, 3 ];
arr2 = [ 5, 6] ;
arr3 = [ 4 ];
arr4 = [ 7, 8, 9] ;
# creating Four linked lists
# Passing array and size of array
# as parameters
head1 = createList(arr1, 3);
head2 = createList(arr2, 2);
head3 = createList(arr3, 1);
head4 = createList(arr4, 3);
# Initializing children and next pointers
# as shown in given diagram
head1.child = head2;
head1.next.next.child = head3;
head2.next.child = head4;
# Creating a None pointer.
head = None;
head = head1;
# Function Call to print
printMultiLevelList(head);
# This code is contributed by umadevi9616
C#
/*package whatever //do not write package name here */
using System;
public class GFG {
public class Node {
public int data;
public Node next;
public Node child;
};
// A function to create a linked list
// with n(size) nodes returns head pointer
public static Node createList(int []arr, int n)
{
Node head = null;
Node tmp = null;
// Traversing the passed array
for (int i = 0; i < n; i++)
{
// Creating a node if the list
// is empty
if (head == null) {
tmp = head = new Node();
}
else {
tmp.next = new Node();
tmp = tmp.next;
}
// Created a node with data and
// setting child and next pointer
// as NULL.
tmp.data = arr[i];
tmp.next = tmp.child = null;
}
return head;
}
// To print the linked list
public static void printMultiLevelList(Node head)
{
// While head is not null
while (head != null) {
if (head.child != null) {
printMultiLevelList(head.child);
}
Console.Write(head.data + " ");
head = head.next;
}
}
// Driver code
public static void Main(String[] args)
{
int []arr1 = { 1, 2, 3 };
int []arr2 = { 5, 6 };
int []arr3 = { 4 };
int []arr4 = { 7, 8, 9 };
// creating Four linked lists
// Passing array and size of array
// as parameters
Node head1 = createList(arr1, 3);
Node head2 = createList(arr2, 2);
Node head3 = createList(arr3, 1);
Node head4 = createList(arr4, 3);
// Initializing children and next pointers
// as shown in given diagram
head1.child = head2;
head1.next.next.child = head3;
head2.next.child = head4;
// Creating a null pointer.
Node head = null;
head = head1;
// Function Call to print
printMultiLevelList(head);
}
}
// This code is contributed by shikhasingrajput
Javascript
输出
5 7 8 9 6 1 2 4 3