在Java中使用 OpenCV 旋转图像
图像旋转是一种常见的图像处理程序,用于以任何所需的角度旋转图像。这有助于图像反转、翻转和获得图像的预期视图。图像旋转在匹配、对齐和其他基于图像的算法中有应用。 OpenCV 是一个著名的用于图像处理的库。
方法:
在切换之前,为Java设置一个 OpenCV 环境以处理图像,然后对它们执行操作。现在,借助warpAffine() 方法在 OpenCV 中执行图像旋转。使用这种技术可以以任何角度旋转图像,为此需要定义要围绕哪个点或轴旋转。
如上所述,依次遵循以下步骤:
- 确定要进行旋转的点和旋转角度。
- 获取源图像的旋转矩阵。
- 使用获得的旋转矩阵对源图像使用仿射变换。
除了这种方法之外,还有两种方法可以以 90 度的倍数(即 ±90、±180、±270)的角度旋转图像。仿射变换的计算强度是其数十倍。仿射插值并使用大量浮点运算。对于 90 度的倍数的角度,以下方法比warpAffine()更有效。
可能发生的图像旋转用例
- 旋转法
- 转置和翻转方法
所需方法:
- 经仿射()
- getRotationMatrix2D()
- 旋转()
- 翻动()
- 转置()
方法描述如下:
1. warpAffine(): Applies an affine transformation to an image.
Syntax:
Parameters:
- src – input image.
- dst – output image that has the size dsize and the same type as src.
- M – 2×3 transformation (rotation) matrix.
- dsize – size of the output image.
- flags – Optional field. WARP_INVERSE_MAP flag.
- When flag is not provided, image mapping is in counterclockwise direction.
- If the WARP_INVERSE_MAP flag is set, image mapping is in clockwise direction.
2. getRotationMatrix2D() : Calculates an affine matrix of 2D rotation.
Syntax:
Parameters:
- center – Center of the rotation in the source image.
- angle – Rotation angle in degrees. Positive values mean counter-clockwise rotation
- scale – Isotropic scale factor.
3. rotate() : Rotates a 2D array in multiples of 90 degrees.
Syntax:
Parameters:
- src – input array.
- dst – output array of the same type as src.
- rotateCode – An enum to specify how to rotate the array.
- ROTATE_90_CLOCKWISE : Rotate image by 90 degrees clockwise or 270 degrees counter clockwise
- ROTATE_90_COUNTERCLOCKWISE : Rotate image by 90 degrees counterclockwise or 270 degrees clockwise
- ROTATE_180 : Rotate by 180 degrees.
4. flip(): Flips a 2D array around vertical, horizontal, or both axes.
Syntax:
Core.flip(Mat src, Mat dst, int flipCode)
Parameters:
- src – input array.
- dst – output array of the same size and type as src.
- flipCode – a flag to specify how to flip the array.
- flipCode=0 means flipping around the x-axis.
- flipCode>0 (Positive value such as, 1) means flipping around y-axis.
- flipcode<0 (Negative value such as, -1) means flipping around both axes.
5. Transpose() : Transposes a matrix.
Syntax:
Core.transpose(Mat src, Mat dst)
Parameters:
- src – input array.
- dst – output array of the same type as src.
插图:
Input: Considering a sample input image:
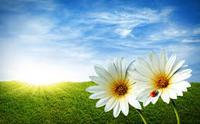
Input Image
Pseudo Code:
double angle=45
Point rotPoint=new Point(src.cols()/2.0, src.rows()/2.0)
Mat rotMat = Imgproc.getRotationMatrix2D( rotPoint, angle, 1);
Imgproc.warpAffine(src, dst, rotMat, src.size());
Steps to be followed-
- midpoint of a source image,
- rotPoint, is considered for rotation,
- rotation angle is 45°.
- Rotation matrix is obtained by getRotationMatrix2D() method.
- Affine transformation is performed using warpAffine() method.
- Input image will be rotated in 45° counterclockwise direction as shown below.
Output: Image is as shown:
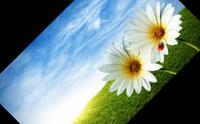
45° Counter clockwise Rotated Image
示例输入图像:用于实现目的
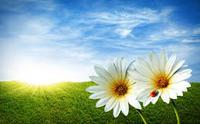
输入图像
案例 1:旋转方法 -考虑实现部分的相同输入图像。
使用rotate 方法旋转图像涉及正确使用rotatecode。
- 如果角度是 90° 或 -270°
- 使用旋转代码 → ROTATE_90_CLOCKWISE
- 如果角度是 180° 或 -180°
- 使用旋转代码 → ROTATE_180
- 如果角度是 270° 或 -90°
- 使用旋转代码 → ROTATE_90_COUNTERCLOCKWISE
例子
Java
Imgproc.warpAffine(Mat src, Mat dst, Mat M, Size dsize, int flags)
Java
Imgproc.getRotationMatrix2D(Point center, double angle, double scale)
输出:
案例 2:转置和翻转方法
- 如果角度为 90° 或 -270°,则转置源图像矩阵,然后
- 用正值翻转它作为翻转码
- 如果角度为 270° 或 -90°,则转置源图像矩阵,然后
- 用 0 作为翻转码翻转它
- 如果角度为 180°,则以 -1 作为翻转码翻转源图像
执行:
Java
Core.rotate(Mat src, Mat dst, int rotateCode)
输出: