在Java中使用 OpenCV 缩放图像
图像缩放是指图像的大小调整。它在机器学习应用程序中的图像处理和操作中很有用,因为它可以减少训练时间,因为像素数较少,模型的复杂性也较低。 OpenCV 库的 Imgproc 模块为调整图像大小提供了适当的插值方法。
Imgproc.resize OpenCV 的 Imgproc 模块的方法可用于调整图像大小。此函数将图像 src 大小调整为指定大小。它提供了调整图像大小的所有功能,即缩小或缩放图像以满足尺寸要求。
句法:
resize(
src: &Mat,
dst: &mut Mat,
dsize: Size,
fx: f64,
fy: f64,
interpolation: i32
)
参数: It is the method for image decimation. Default interpolation is INTER_LINEAR interpolationName Definition src It is the input image of the MAT type. dst It is the output image of MAT type dsize It is the size of the output image of Size type fx Scale factor along X-axis of double type fy Scale factor along Y-axis of double type interpolation
算法:
- 加载 OpenCV 模块。
- 从目录中读取图像(二维矩阵)。
- 将图像/2D 矩阵存储为 Mat 对象。
- 使用对象创建图像/2D 矩阵。
- 使用上述创建的对象进行缩放和写入。
样品:
假设用户想要调整 src 的大小,使其适合预先创建的目标文件,那么调用该函数的语法如下
resize(src, dst, dst.size(), 0, 0, interpolation);
假设用户想在每个方向将图像缩小2倍,那么调用该函数的语法如下
resize(src, dst, Size(), 0.5, 0.5, interpolation);
实现:将下面的图像视为应该缩放的输入图像,以产生新的输出图像。这里输入图像应该按比例缩小,这在示例的帮助下进行了说明。
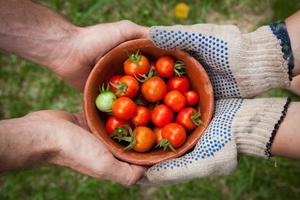
输入图像
Java
// Importing openCV modules
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.core.Size;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
public class GFG {
// Main driver code
public static void main(String args[])
{
// Load OpenCV library
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
// Reading the Image from the file/ directory
String image_location
= "C:\\Users\\user\\Downloads\\InputImage.jpg";
// Storing the image in a Matrix object
// of Mat type
Mat src = Imgcodecs.imread(image_location);
// New matrix to store the final image
// where the input image is supposed to be written
Mat dst = new Mat();
// Scaling the Image using Resize function
Imgproc.resize(src, dst, new Size(0, 0), 0.1, 0.1,
Imgproc.INTER_AREA);
// Writing the image from src to destination
Imgcodecs.imwrite("D:/resized_image.jpg", dst);
// Display message to show that
// image has been scaled
System.out.println("Image Processed");
}
}
输出:
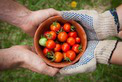
最终图像