Flutter的 Circular Reveal Animation 的灵感来自ViewAnimationUtils.createCircularReveal(…)。它完全符合名称的含义,这意味着它通常用于显示内容,通常是圆形图像,其中圆圈变大并使图像可见。
在本文中,我们将通过一个简单的应用程序实现一个简单的圆形显示动画。要构建相同的,请按照以下步骤操作:
- 将依赖项添加到pubspec.yaml 文件。
- 创建一个图像目录并添加要在其中显示的图像。
- 激活pubspec.yaml 文件中的资产
- 将依赖项导入到 main 中。dart文件
- 通过扩展StatelessWidget创建应用程序的根
- 通过扩展StatefulWidget 来设计主页
- 在主页的正文中调用CircularRevealAnimation()。
- 添加一个floatingActionButton并为其分配一个动作以显示图像。
让我们详细看看这些步骤。
添加依赖
将circular_reveal_animation依赖添加到pubspec.yaml 文件的dependencies 部分,如下所示:

依赖
添加图像
使用圆形显示动画显示的图像可以添加到资产目录内的图像目录中,如下所示:
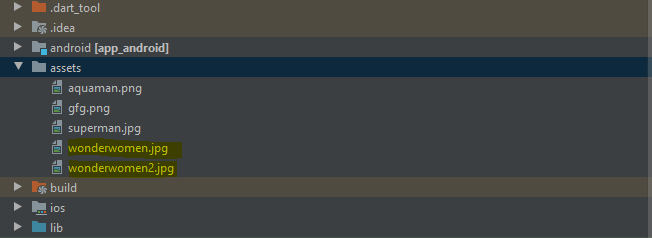
添加图像
激活资产
在pubspec.yanl 文件中,可以通过在资产部分下添加图像路径来激活资产以在应用程序中使用:

激活资产
导入依赖
在 main.c 中使用下面的代码行。 dart文件以使用circle_reveal_animation依赖项:
import 'package:circular_reveal_animation/circular_reveal_animation.dart';
创建应用程序根
扩展一个StatelessWidget来为应用程序创建一个根目录,如下所示:
Dart
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'GeeksForGeeks',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
Dart
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State
with SingleTickerProviderStateMixin {
AnimationController animationController;
Animation animation;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("GeeksForGeeks"),
backgroundColor: Colors.green,
),
body:
),
Dart
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
SizedBox(height: 16),
CircularRevealAnimation(
child: Image.asset('assets/wonderwomen.jpg'),
animation: animation,
centerAlignment: Alignment.centerRight,
centerOffset: Offset(130, 100),
minRadius: 12,
maxRadius: 200,
),
],
),
),
),
Dart
floatingActionButton: FloatingActionButton(
backgroundColor: Colors.green,
onPressed: () {
if (animationController.status == AnimationStatus.forward ||
animationController.status == AnimationStatus.completed) {
animationController.reverse();
} else {
animationController.forward();
}
}),
);
Dart
import 'package:circular_reveal_animation/circular_reveal_animation.dart';
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
// root
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'GeeksForGeeks',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
// Homepage
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State
with SingleTickerProviderStateMixin {
AnimationController animationController;
Animation animation;
// initialize state
@override
void initState() {
super.initState();
animationController = AnimationController(
vsync: this,
duration: Duration(milliseconds: 1000),
);
animation = CurvedAnimation(
parent: animationController,
curve: Curves.easeIn,
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("GeeksForGeeks"),
backgroundColor: Colors.green,
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
SizedBox(height: 16),
CircularRevealAnimation(
child: Image.asset('assets/wonderwomen.jpg'),
animation: animation,
centerAlignment: Alignment.centerRight,
centerOffset: Offset(130, 100),
minRadius: 12,
maxRadius: 200,
),
],
),
),
),
// button with assigned action
floatingActionButton: FloatingActionButton(
backgroundColor: Colors.green,
onPressed: () {
if (animationController.status == AnimationStatus.forward ||
animationController.status == AnimationStatus.completed) {
animationController.reverse();
} else {
animationController.forward();
}
}),
);
}
}
创建主页
将StatefulWidget扩展到appbar和body上,为应用创建一个平面主页,如下图:
Dart
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State
with SingleTickerProviderStateMixin {
AnimationController animationController;
Animation animation;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("GeeksForGeeks"),
backgroundColor: Colors.green,
),
body:
),
调用依赖
在主页的正文中调用CircularRevealAnimation()函数并将图像添加到相同的内容中,如下所示:
Dart
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
SizedBox(height: 16),
CircularRevealAnimation(
child: Image.asset('assets/wonderwomen.jpg'),
animation: animation,
centerAlignment: Alignment.centerRight,
centerOffset: Offset(130, 100),
minRadius: 12,
maxRadius: 200,
),
],
),
),
),
添加按钮
添加一个FloatingActionButton并为其分配一个动作,该动作从对按下的依赖中调用CircularRevealAnimation() ,如下所示:
Dart
floatingActionButton: FloatingActionButton(
backgroundColor: Colors.green,
onPressed: () {
if (animationController.status == AnimationStatus.forward ||
animationController.status == AnimationStatus.completed) {
animationController.reverse();
} else {
animationController.forward();
}
}),
);
完整的源代码:
Dart
import 'package:circular_reveal_animation/circular_reveal_animation.dart';
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
// root
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'GeeksForGeeks',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
// Homepage
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State
with SingleTickerProviderStateMixin {
AnimationController animationController;
Animation animation;
// initialize state
@override
void initState() {
super.initState();
animationController = AnimationController(
vsync: this,
duration: Duration(milliseconds: 1000),
);
animation = CurvedAnimation(
parent: animationController,
curve: Curves.easeIn,
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("GeeksForGeeks"),
backgroundColor: Colors.green,
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
SizedBox(height: 16),
CircularRevealAnimation(
child: Image.asset('assets/wonderwomen.jpg'),
animation: animation,
centerAlignment: Alignment.centerRight,
centerOffset: Offset(130, 100),
minRadius: 12,
maxRadius: 200,
),
],
),
),
),
// button with assigned action
floatingActionButton: FloatingActionButton(
backgroundColor: Colors.green,
onPressed: () {
if (animationController.status == AnimationStatus.forward ||
animationController.status == AnimationStatus.completed) {
animationController.reverse();
} else {
animationController.forward();
}
}),
);
}
}
输出: