React Native 中的 Axios
Axios 是一个广泛使用的 HTTP 客户端,用于进行 REST API 调用。你可以在 React Native 中使用它来从任何 REST API 获取数据。
Axios 的特点:
- 它可以发出 XMLHttpRequests 和 HTTP 请求。
- 它可以理解来自 API 的所有请求和响应。
- Axios 是基于承诺的。
- 它可以转换 JSON 格式的响应。
如何在 React Native 中安装 Axios:
你可以使用 npm 或 yarn 在你的 React Native 项目中安装 Axios。在您的计算机上打开终端并转到您的项目目录。
- 使用 npm:
npm install axios
- 使用纱线:
yarn add axios
你可以在 React Native 中使用 Axios 发出 GET 和 POST 请求:
- GET 请求用于从 API 获取数据。
- POST 请求用于修改 API 服务器上的数据。
GET: axios.get() 方法用于在 Axios 中使用 React Native 执行 GET 请求。它需要一个基本 URL 来获取数据。您可以使用 params 指定要与基本 URL 一起传递的参数。
如果它成功执行,您将收到响应。此响应将包含有关请求的数据和其他信息。如果发生任何错误,则 catch 语句将得到该错误。
如果您希望每次都执行某些内容,在这种情况下,您可以将其写在语句下。
句法:
axios.get('/GeeksforGeeks', {
params: {
articleID: articleID
}
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
})
.then(function () {
// always executed
});
POST: axios.post() 方法用于在带有 React Native 的 Axios 中执行 POST 请求。它采用基本 URL 和参数来执行此操作。您可以指定要通过对象与基本 URL 一起传递的参数。
如果它成功执行,您将收到响应。此响应将包含有关请求的数据和其他信息。
如果发生任何错误,则 catch 语句将得到该错误。
axios.post('/GeeksforGeeks', {
articleID: 'articleID',
title: 'Axios in React Native'
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
创建反应应用程序:
第 1 步:打开终端并运行以下命令。它将在您的系统中全局安装 Expo CLI。
npm install -g expo-cli
第 2 步:现在,通过运行以下命令创建一个新的 React Native 项目。
expo init "Your_Project_Name"
第 3 步:您将被要求选择一个模板。选择空白模板。
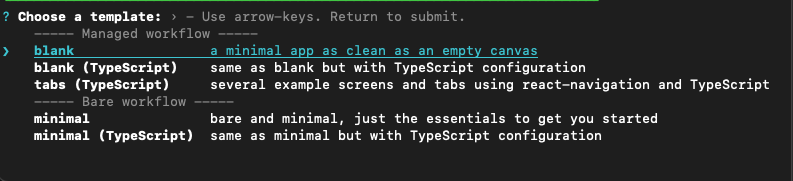
空白模板
第 4 步:现在转到项目文件夹并运行以下命令来启动服务器。
cd "Your_Project_Name"
npm start
第 5 步:使用 npm 或 yarn 命令安装 Axios。
npm install axios
要么
yarn add axios
项目结构:它将如下所示。
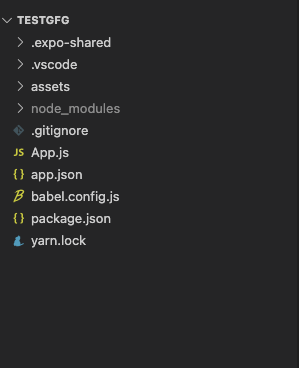
项目结构
我们将在 React Native 中使用 Axios 发出 GET 请求。我们将在这个例子中使用adviceslip API。此 API 将 id 作为参数并提供与该 id 相关的建议。
我们将声明一个随机生成 1 个 id 的函数,并在 Axios GET 请求的参数中传递这个 id。
我们的主App.js文件中将有 2 个组件,Text 和 Button。当您按下按钮时,Axios 将向通知 API 发出 GET 请求并获取一个随机通知。稍后,我们将使用 Text 组件在屏幕上显示此建议。
如果你想要一个完整的 React Native 项目,请使用这个 GitHub 存储库。
示例:打开App.js文件并在该文件中写入以下代码。
Javascript
import { useState } from "react";
import { StyleSheet, View, Text, Button } from "react-native";
import axios from "axios";
export default function App() {
const [advice, setAdvice] = useState("");
const getRandomId = (min, max) => {
min = Math.ceil(min);
max = Math.floor(max);
return (Math.floor(Math.random() *
(max - min + 1)) + min).toString();
};
const getAdvice = () => {
axios
.get("http://api.adviceslip.com/advice/" +
getRandomId(1, 200))
.then((response) => {
setAdvice(response.data.slip.advice);
});
};
return (
{advice}
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#fff",
alignItems: "center",
justifyContent: "center",
},
advice: {
fontSize: 20,
fontWeight: "bold",
marginHorizontal: 20,
},
});
输出:
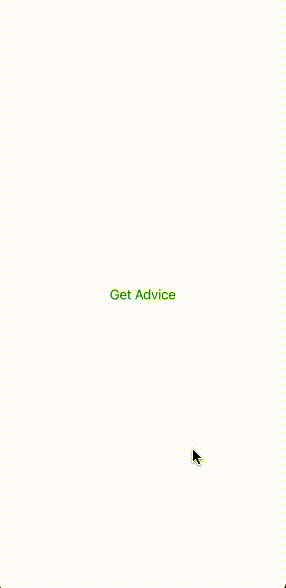
使用 Axios 获取请求