Python的in_place 模块
有时,在处理Python文件时,我们可以使用实用程序来更改文件,而无需使用系统上下文或 sys stdout。这种情况需要就地读/写文件,即不使用进程资源。这可以使用Python的 ein_place 模块来实现。这个模块——
- 不使用 sys.stdout,而是返回新的文件处理程序实例。
- 支持所有文件处理程序方法。
- 在异常情况下,保留原始文件,有助于原子性。
- 创建临时文件不会影响其他打开的文件。
安装
只需运行此命令即可安装所需的模块
pip install in_place
现在要实际完成工作,请使用此模块的 in_place() 方法。
Syntax:
in_place.InPlace(filename, mode=t, backup=None, backup_ext=None, delay_open=False, move_first=False)
Parameter:
filename : Location of file to open and edit inplace.
mode : Mode with which to open file. Options are byte (b) and text(t). In case its open in byte more, read and write happens in bytes. In text mode, R & W happens in string. Defaults to text mode.
backup : Path to save original file after the end of process in case to keep backup of original file.
backup_ext : Created backup of with name – filename + backup_ext, of original file before editing.
delay_open : In case set to True, it will only open file after calling open(), whereas by default its open as soon as class is initiated.
move_first : If set to True, the input file is sent to temporary location and output is edited in place first. By default this process takes place after calling of close() or when process ends.
使用此函数首先打开正在使用的文件并对其执行所需的任务。下面给出的是相同的最简单的实现。
示例 1:
输入:

原创内容
Python3
import in_place
# using inplace() to perform same file edit.
with in_place.InPlace('gfg_inplace') as f:
for line in f:
# reversing cases while writing in file, inplace
f.write(''.join(char.upper() if char.islower() else char.lower()
for char in line))
Python3
import in_place
# using inplace() to perform same file edit.
# backs up original file in given path.
with in_place.InPlace('gfg_inplace', backup='origin_gfg_inplace') as f:
for line in f:
# reversing cases while writing in file, inplace
f.write(''.join(char.upper() if char.islower() else char.lower()
for char in line))
Python3
import in_place
# using inplace() to perform same file edit.
# adds extension to file and creates backup
with in_place.InPlace('gfg_inplace', backup_ext='-original') as f:
for line in f:
# reversing cases while writing in file, inplace
f.write(''.join(char.upper() if char.islower() else char.lower()
for char in line))
输出 :
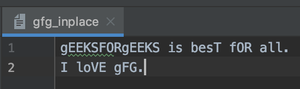
代码执行后文件的输出。- 改变情况。
也可以先将现有文件的数据备份到其他文件,然后对该文件进行所需的更改。为此,您要通过其创建备份的文件的名称作为备份参数的值给出。
示例 2:
输入:
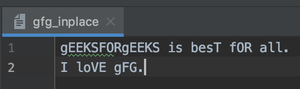
代码执行后文件的输出。- 改变情况。
蟒蛇3
import in_place
# using inplace() to perform same file edit.
# backs up original file in given path.
with in_place.InPlace('gfg_inplace', backup='origin_gfg_inplace') as f:
for line in f:
# reversing cases while writing in file, inplace
f.write(''.join(char.upper() if char.islower() else char.lower()
for char in line))
输出 :
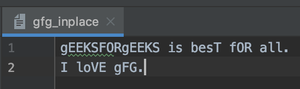
代码执行后,就地更改。
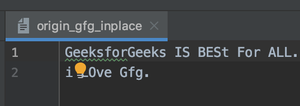
在新文件中创建原始内容的备份。
上述方法的替代方法是使用关键字backup_ext。到这个 只需传递额外的单词来区分现有文件。
示例 3:
输入:
蟒蛇3
import in_place
# using inplace() to perform same file edit.
# adds extension to file and creates backup
with in_place.InPlace('gfg_inplace', backup_ext='-original') as f:
for line in f:
# reversing cases while writing in file, inplace
f.write(''.join(char.upper() if char.islower() else char.lower()
for char in line))
输出 :
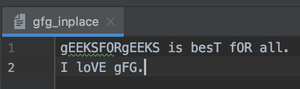
代码执行后,就地更改。
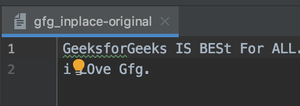
创建原始文件的备份,添加给定的扩展名。