角度材质按钮切换
Angular Material是由 Google 开发的 UI 组件库,以便 Angular 开发人员可以以结构化和响应式的方式开发现代应用程序。通过使用这个库,我们可以极大地提高最终用户的用户体验,从而为我们的应用程序赢得人气。该库包含现代即用型元素,可以直接使用最少或无需额外代码。
Angular 材质中的Button Toggle组件允许用户在打开和关闭状态之间切换以执行特定操作。
语法:
Toggle Button
句法:
Toggle Button 1
Toggle Button 2
...
安装语法:
基本的先决条件是我们必须在系统上安装 Angular CLI 才能添加和配置 Angular 材质库。在 Angular CLI 上执行以下命令以安装 Angular 材质库:
ng add @angular/material
在执行上述命令之前,请确保应在终端中打开路径。
有关详细的安装过程,请参阅将 Angular 材质组件添加到 Angular 应用程序一文。
添加按钮切换组件:
要使用 Button Toggle 组件,我们需要将其导入app.module.ts文件:
import {MatButtonToggleModule} from '@angular/material/button-toggle';
要在我们的代码中使用切换按钮组件,我们必须将MatButtonToggleModule导入到 imports 数组中。
项目结构:安装成功后,项目结构如下图所示:
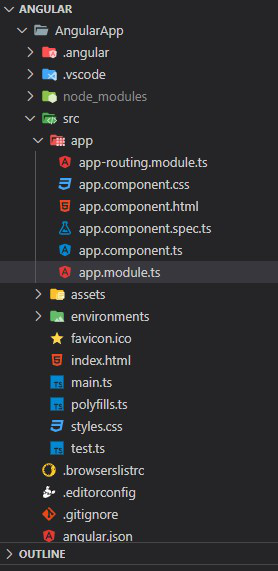
项目结构
示例 1 :下面的示例说明了 Angular Material Button Toggle 的实现。
app.component.html
GeeksforGeeks
Angular Material Button Toggle
Toggle Button 1
Toggle Button 2
You have selected : {{ toggleBtn.value }}
app.component.ts
import { Component } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
title = "AngularApp";
}
app.module.ts
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { FormsModule } from "@angular/forms";
import { AppComponent } from "./app.component";
import { MatButtonToggleModule }
from "@angular/material/button-toggle";
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations";
@NgModule({
imports: [
BrowserModule,
FormsModule,
MatButtonToggleModule,
BrowserAnimationsModule,
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
app.component.html
GeeksforGeeks
Angular Material Button Toggle
Single selection
Angular Material UI
React Material UI
You have selected : {{ toggleGroup1.value }}
Multiple selection
Angular
React
Vue
You have selected : {{ toggleGroup2.value }}
app.component.ts
import { Component } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
title = "AngularApp";
}
app.module.ts
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { FormsModule } from "@angular/forms";
import { AppComponent } from "./app.component";
import { MatButtonToggleModule }
from "@angular/material/button-toggle";
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations";
@NgModule({
imports: [
BrowserModule,
FormsModule,
MatButtonToggleModule,
BrowserAnimationsModule,
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
app.component.ts
import { Component } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
title = "AngularApp";
}
app.module.ts
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { FormsModule } from "@angular/forms";
import { AppComponent } from "./app.component";
import { MatButtonToggleModule }
from "@angular/material/button-toggle";
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations";
@NgModule({
imports: [
BrowserModule,
FormsModule,
MatButtonToggleModule,
BrowserAnimationsModule,
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
输出:
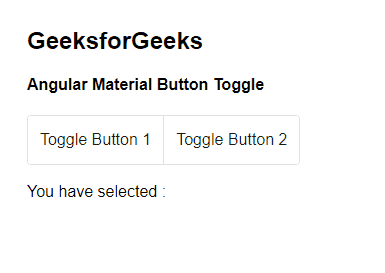
角度材质按钮切换
按钮切换选择模式:
Angular Toggle Button 有 2 种选择模式:
- 单选:
它只允许在切换组中选择一项。要创建单个选择切换按钮,
- 多项选择:
它允许在切换组中选择多个项目。为了创建一个多选切换按钮,
示例 2 :下面的示例通过在 Angular Material 中指定不同的选择模式来说明 Button Toggle 的实现。
app.component.html
GeeksforGeeks
Angular Material Button Toggle
Single selection
Angular Material UI
React Material UI
You have selected : {{ toggleGroup1.value }}
Multiple selection
Angular
React
Vue
You have selected : {{ toggleGroup2.value }}
app.component.ts
import { Component } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
title = "AngularApp";
}
app.module.ts
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { FormsModule } from "@angular/forms";
import { AppComponent } from "./app.component";
import { MatButtonToggleModule }
from "@angular/material/button-toggle";
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations";
@NgModule({
imports: [
BrowserModule,
FormsModule,
MatButtonToggleModule,
BrowserAnimationsModule,
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
输出:
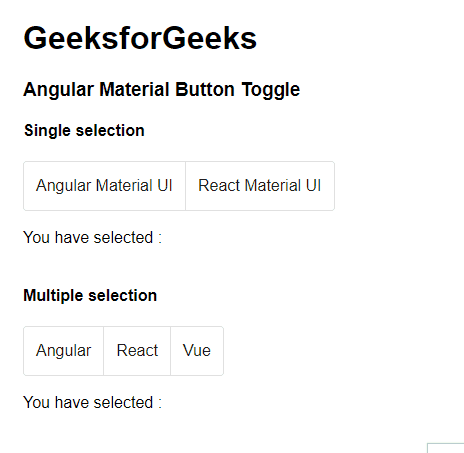
按钮切换选择模式
参考:https://material.angular.io/components/button-toggle/overview