聚合查询
MongoDB 是一个 NoSQL 文档模型数据库,主要用于大数据分析,如选举投票结果、过去几年一个行业的发展情况等,为了做巨大的计算,在 MongoDB 中,我们使用聚合。
聚合对来自多个文档的数据进行分组,并提供汇总结果、大型结果集的平均值、大型结果集的最小值/最大值等。在本文中,让我们看看如何使用Mongoose执行聚合。
模块安装:使用以下命令安装所需的模块。
npm install mongoose
数据库:以下是 MongoDB 数据库中的集合中存在的示例数据。
Database Name: UserDB
Collection Name: UserValidation
下图显示其中存在文档。特别是单独使用ssn和工资列。
db.UserValidation.find({},{_id:0,salary:1,ssn:1,firstName:1});
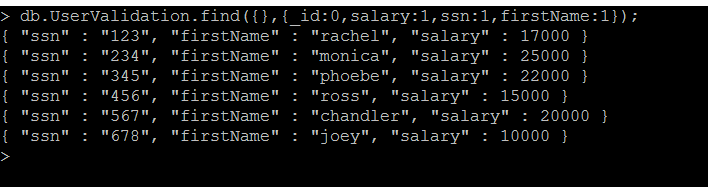
“UserValidation”集合中存在数据
项目结构:它看起来像这样。
示例 1:工资值的总和。
语法:当我们对字段求和时,我们需要一个分组操作($group),然后是求和($sum),如下所示。
db.UserValidation.aggregate([
{
$group: {
_id: null,
salarycount: {
$sum: "$salary"
}
}
}
]);
文件名:server.js
Javascript
// Requiring module
const mongoose = require("mongoose");
// Assumption is in our local, we have "UserDB"
// named mongoDB database available
mongoose.connect(
"mongodb://localhost:27017/UserDB",
function (err, db) {
console.log("Connected correctly to server");
// "UserValidation" named collection
// available and each document contains
// "salary" named column
var col = db.collection('UserValidation');
// By applying aggregate functionality,
// finding the summation of salary and
// the result is in "summedUpDocument"
col.aggregate([{
$group:
{ _id: null, total: { $sum: '$salary' } }
}]).toArray(function (err, summedUpDocument) {
// Summed up salary value can be printed
// as like below
console.log(summedUpDocument[0].total)
db.close();
})
});
Javascript
// Requiring module
const mongoose = require("mongoose");
// Assumption is in our local, we have
// "UserDB" named mongoDB database
// available
mongoose.connect(
"mongodb://localhost:27017/UserDB",
function (err, db) {
console.log("Connected correctly to server");
// "UserValidation" named collection
// available and each document contains
// "salary" named column
var col = db.collection('UserValidation');
// By applying aggregate functionality,
// finding the summation of salary
// Here applied match condition where
// matching address equal to
// Chennai and result will be in
// summedUpDocumentForChennai
col.aggregate([
{ $match: { address: { $eq: "Chennai" } } },
{
$group:
{ _id: null, total: { $sum: '$salary' } }
}
]).toArray(function (err, summedUpDocumentForChennai) {
// Summed up salary value can be printed
// as like below
console.log(summedUpDocumentForChennai[0].total)
db.close();
})
});
Javascript
// Requiring module
const mongoose = require("mongoose");
// Assumption is in our local, we have
// "UserDB" named mongoDB database
// available
mongoose.connect(
"mongodb://localhost:27017/UserDB",
function (err, db) {
console.log("Connected correctly to server");
// "UserValidation" named collection
// available and each document contains
// "salary" named column
var col = db.collection('UserValidation');
// By applying aggregate functionality,
// finding the summation of salary
// Here applied match condition where
// matching address equal to Chennai
// We are calculating total, average,
// minimum and maximum amount
col.aggregate([
{ $match: { address: { $eq: "Chennai" } } },
{
$group:
{
_id: null, totalSalary: { $sum: "$salary" },
averageSalary: { $avg: "$salary" },
minimumSalary: { $min: "$salary" },
maximumSalary: { $max: "$salary" }
}
}
]).toArray(function (err, projectFunctionality) {
console.log("Total Salary ..",
projectFunctionality[0].totalSalary)
console.log("Average Salary ..",
projectFunctionality[0].averageSalary)
console.log("Minimum Salary ..",
projectFunctionality[0].minimumSalary)
console.log("Maximum Salary ..",
projectFunctionality[0].maximumSalary)
db.close();
})
});
运行程序的步骤:使用以下命令运行服务器。
node server.js
输出:
示例 2:计算基于位置的员工工资总和。
语法:为了提供额外的过滤条件,我们可以使用 $match运算符。
db.UserValidation.aggregate([
{
$match: {
address: {
$eq: "Chennai"
}
}
},
{
$group: {
_id: null,
salarycount: {
$sum: "$salary"
}
}
}]);
在这里,我们将地址列匹配为仅具有Chennai 。因此,仅对与钦奈地址值匹配的文件进行汇总。
文件名:server.js
Javascript
// Requiring module
const mongoose = require("mongoose");
// Assumption is in our local, we have
// "UserDB" named mongoDB database
// available
mongoose.connect(
"mongodb://localhost:27017/UserDB",
function (err, db) {
console.log("Connected correctly to server");
// "UserValidation" named collection
// available and each document contains
// "salary" named column
var col = db.collection('UserValidation');
// By applying aggregate functionality,
// finding the summation of salary
// Here applied match condition where
// matching address equal to
// Chennai and result will be in
// summedUpDocumentForChennai
col.aggregate([
{ $match: { address: { $eq: "Chennai" } } },
{
$group:
{ _id: null, total: { $sum: '$salary' } }
}
]).toArray(function (err, summedUpDocumentForChennai) {
// Summed up salary value can be printed
// as like below
console.log(summedUpDocumentForChennai[0].total)
db.close();
})
});
运行程序的步骤:使用以下命令运行服务器。
node server.js
输出:
示例 3:获取平均值/最小值/最大值
语法:我们需要使用 avg 功能来获取平均/最小功能,以获取最小/最大功能,使用以下语法。
db.UserValidation.aggregate([{
$match: { address: { $eq: "Chennai" } }
},
{
$group: {
_id: null,
totalSalary: { $sum: "$salary" },
averageSalary: { $avg: "$salary" },
minimumSalary: { $min: "$salary" },
maximumSalary: { $max: "$salary" }
}
}
])
文件名:server.js
Javascript
// Requiring module
const mongoose = require("mongoose");
// Assumption is in our local, we have
// "UserDB" named mongoDB database
// available
mongoose.connect(
"mongodb://localhost:27017/UserDB",
function (err, db) {
console.log("Connected correctly to server");
// "UserValidation" named collection
// available and each document contains
// "salary" named column
var col = db.collection('UserValidation');
// By applying aggregate functionality,
// finding the summation of salary
// Here applied match condition where
// matching address equal to Chennai
// We are calculating total, average,
// minimum and maximum amount
col.aggregate([
{ $match: { address: { $eq: "Chennai" } } },
{
$group:
{
_id: null, totalSalary: { $sum: "$salary" },
averageSalary: { $avg: "$salary" },
minimumSalary: { $min: "$salary" },
maximumSalary: { $max: "$salary" }
}
}
]).toArray(function (err, projectFunctionality) {
console.log("Total Salary ..",
projectFunctionality[0].totalSalary)
console.log("Average Salary ..",
projectFunctionality[0].averageSalary)
console.log("Minimum Salary ..",
projectFunctionality[0].minimumSalary)
console.log("Maximum Salary ..",
projectFunctionality[0].maximumSalary)
db.close();
})
});
运行程序的步骤:使用以下命令运行服务器。
node server.js
输出:
注意:默认情况下,_id 字段将可用于输出文档。我们可以使用 1 在输出文档中包含一个字段,使用 0 来抑制。
结论:聚合查询非常强大,其功能类似于 SQL 的group by子句(即 $group)和功能(即 $match)。 MongoDB 是一个非常强大的 NoSQL 文档类型数据库,聚合查询在其中发挥着至关重要的作用。
参考资料: https://docs.mongodb.com/manual/aggregation/