如何修复:“numpy.ndarray”对象没有属性“append”
NumPy 是Python编程语言的库,增加了对大型多维数组和矩阵的支持,以及对这些数组进行操作的大量高级数学函数集合。如果您从事分析,您可能在Python中遇到过这个库。一开始,从Python传统列表切换到 NumPy 数组时,有些事情可能会让程序员感到困惑。我们可能遇到的一个这样的错误是“ AttributeError: 'numpy.ndarray' object has no attribute 'append' ”。在本文中,让我们看看为什么会看到此错误以及如何修复它。
将项目添加到Python列表时,我们使用列表的 append 方法。语法非常简单,当我们尝试在 NumPy 数组上复制相同的内容时,我们会得到上述错误。让我们通过一个例子来看看它。
示例:描述错误的代码
Python
# Append method on python lists
import numpy
print("-"*15, "Python List", "-"*15)
# Create a python list
pylist = [1, 2, 3, 4]
# View the data type of the list object
print("Data type of python list:", type(pylist))
# Add (append) an item to the python list
pylist.append(5)
# View the items in the list
print("After appending item 5 to the pylist:", pylist)
print("-"*15, "Numpy Array", "-"*15)
# Append method on numpy arrays
# Import the numpy library
# Create a numpy array
nplist = numpy.array([1, 2, 3, 4])
# View the data type of the numpy array
print("Data type of numpy array:", type(nplist))
# Add (append) an item to the numpy array
nplist.append(5)
Python
# Append method on numpy arrays
# Import the numpy library
import numpy
# Create a numpy array
nplist = numpy.array([1, 2, 3, 4])
# View the data type of the numpy array
print("Data type of numpy array:", type(nplist))
# View the items in the numpy array
print("Initial items in nplist:", nplist)
# Add (append) an item to the numpy array
nplist = numpy.append(nplist, 5)
# View the items in the numpy array
print("After appending item 5 to the nplist:", nplist)
输出:
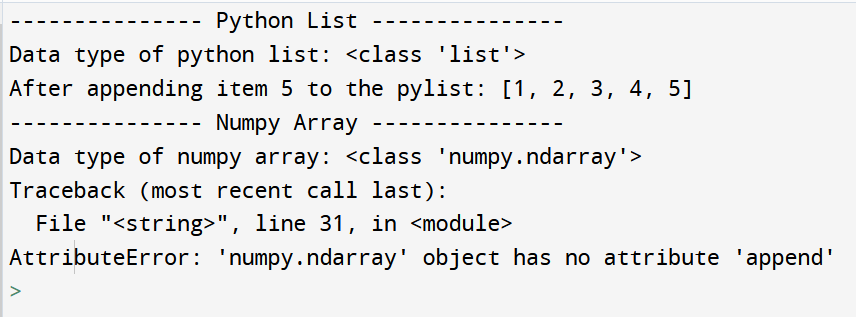
Numpy 追加错误
在上面的输出中,我们可以看到Python list 的数据类型为list 。当我们执行追加操作时,项目即 5 被追加到列表pylist的末尾。当我们对 NumPy 数组尝试相同的方法时,它失败并抛出错误“ AttributeError: 'numpy.ndarray' object has no attribute 'append' ”。输出非常解释性,NumPy 数组有一种numpy.ndarray类型,它没有任何append()方法。
现在,我们知道 NumPy 数组不支持追加,那么我们如何使用它呢?它实际上是 NumPy 的一个方法而不是它的数组,让我们通过下面给出的示例来理解它,我们实际上是对一个 numpy 列表执行附加操作。
Syntax:
numpy.append(arr, values, axis=None)
Parameters:
- arr: numpy array: The array to which the values are appended to as a copy of it.
- values: numpy array or value: These values are appended to a copy of arr. It must be of the correct shape (the same shape as arr, excluding axis). If axis is not specified, values can be any shape and will be flattened before use.
- axis: int, optional: The axis along which values are appended. If axis is not given, both arr and values are flattened before use.
示例:固定代码
Python
# Append method on numpy arrays
# Import the numpy library
import numpy
# Create a numpy array
nplist = numpy.array([1, 2, 3, 4])
# View the data type of the numpy array
print("Data type of numpy array:", type(nplist))
# View the items in the numpy array
print("Initial items in nplist:", nplist)
# Add (append) an item to the numpy array
nplist = numpy.append(nplist, 5)
# View the items in the numpy array
print("After appending item 5 to the nplist:", nplist)
输出:
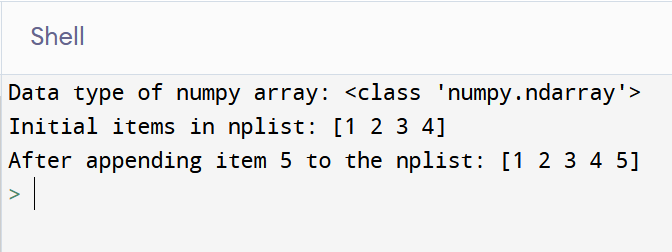
Numpy追加输出
在输出中,我们可以看到最初,NumPy 数组有 4 个项目(1、2、3、4)。将 5 附加到列表后,它会反映在 NumPy 数组中。之所以如此,是因为这里 append函数用于 NumPy 而不是 NumPy 数组对象( numpy.ndarray )。