Android UI 布局
Android布局用于定义用户界面,其中包含将出现在 android 应用程序或活动屏幕上的 UI 控件或小部件。通常,每个应用程序都是 View 和 ViewGroup 的组合。众所周知,一个android应用程序包含大量的活动,我们可以说每个活动都是应用程序的一个页面。因此,每个活动都包含多个用户界面组件,这些组件是 View 和 ViewGroup 的实例。布局中的所有元素都是使用View和ViewGroup对象的层次结构构建的。
看法
View定义为用户界面,用于创建 TextView、ImageView、EditText、RadioButton 等交互 UI 组件,并负责事件处理和绘图。它们通常被称为小部件。
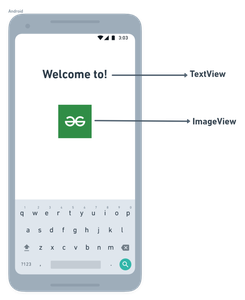
看法
ViewGroup充当布局和布局参数的基类,这些参数包含其他视图或视图组并定义布局属性。它们通常称为布局。
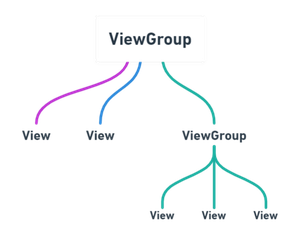
视图组
Android 框架将允许我们以两种方式使用 UI 元素或小部件:
- 在 XML 文件中使用 UI 元素
- 在 Kotlin 文件中动态创建元素
Android 布局的类型
- Android 线性布局: LinearLayout 是 ViewGroup 的子类,用于根据方向属性在水平或垂直的特定方向上一一提供子 View 元素。
- Android 相对布局: RelativeLayout 是 ViewGroup 的子类,用于指定子 View 元素之间的相对位置,例如(A 在 B 的右侧)或相对于父级(固定在父级的顶部)。
- Android Constraint Layout: ConstraintLayout 是 ViewGroup 的子类,用于指定每个子 View 相对于其他存在的 View 的布局约束的位置。 ConstraintLayout 类似于RelativeLayout,但功能更强大。
- Android Frame Layout: FrameLayout是一个ViewGroup子类,用于指定它所包含的View元素在彼此的顶部的位置,以在FrameLayout内只显示单个View。
- Android Table Layout: TableLayout 是ViewGroup 的子类,用于在行和列中显示子View 元素。
- Android Web View: WebView 是一个浏览器,用于在我们的活动布局中显示网页。
- Android ListView: ListView 是一个ViewGroup,用于在单列中显示可滚动的项目列表。
- Android Grid View: GridView 是一个 ViewGroup,用于在行和列的网格视图中显示可滚动的项目列表。
在 XML 文件中使用 UI 元素
在这里,我们可以创建一个类似于网页的布局。 XML 布局文件包含至少一个根元素,可以在其中添加其他布局元素或小部件以构建视图层次结构。以下是示例:
XML
Kotlin
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import android.widget.LinearLayout
import android.widget.Toast
import android.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// create the button
val showButton = Button(this)
showButton.setText("Submit")
// create the editText
val editText = EditText(this)
val linearLayout = findViewById(R.id.l_layout)
linearLayout.addView(editText)
linearLayout.addView(showButton)
// Setting On Click Listener
showButton.setOnClickListener
{
// Getting the user input
val text = editText.text
// Showing the user input
Toast.makeText(this, text, Toast.LENGTH_SHORT).show()
}
}
}
从 Activity 加载 XML 布局文件及其元素
创建布局后,我们需要从活动的onCreate()回调方法加载 XML 布局资源,并使用findViewById从 XML 访问 UI 元素。
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// finding the button
val showButton = findViewById
在这里,我们可以观察上面的代码,发现我们正在使用R.layout.activity_main形式的setContentView方法调用我们的布局。一般来说,在我们的activity启动过程中,android框架会调用onCreate()回调方法来获取一个activity需要的布局。
在 Kotlin 文件中动态创建元素
我们可以在运行时通过在 Kotlin 文件中以编程方式使用自定义 View 和 ViewGroup 对象来创建或实例化 UI 元素或小部件。下面是使用 LinearLayout 创建布局以编程方式在活动中保存 EditText 和 Button 的示例。
科特林
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import android.widget.LinearLayout
import android.widget.Toast
import android.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// create the button
val showButton = Button(this)
showButton.setText("Submit")
// create the editText
val editText = EditText(this)
val linearLayout = findViewById(R.id.l_layout)
linearLayout.addView(editText)
linearLayout.addView(showButton)
// Setting On Click Listener
showButton.setOnClickListener
{
// Getting the user input
val text = editText.text
// Showing the user input
Toast.makeText(this, text, Toast.LENGTH_SHORT).show()
}
}
}
布局的不同属性
XML attributes | Description |
---|---|
android:id | Used to specify the id of the view. |
android:layout_width | Used to declare the width of View and ViewGroup elements in the layout. |
android:layout_height | Used to declare the height of View and ViewGroup elements in the layout. |
android:layout_marginLeft | Used to declare the extra space used on the left side of View and ViewGroup elements. |
android:layout_marginRight | Used to declare the extra space used on the right side of View and ViewGroup elements. |
android:layout_marginTop | Used to declare the extra space used in the top side of View and ViewGroup elements. |
android:layout_marginBottom | Used to declare the extra space used in the bottom side of View and ViewGroup elements. |
android:layout_gravity | Used to define how child Views are positioned in the layout. |