Flutter仪表是一个用dart语言编写的信息感知小部件,用于进行现代、交互式和动画仪表检查,并用于利用Flutter制作出色的便携式应用程序用户界面。在flutter有另一种风格的仪表。
以下是在Flutter应用程序中实现Flutter Gauge 的步骤:
第 1 步:将以下依赖项添加到pubspec.yaml 文件中。
dependencies:
flutter_gauge: ^1.0.8
第二步:导入以下包
import 'package:flutter_gauge/flutter_gauge.dart';
第 3 步:在应用程序的根目录中运行flutter包。
第 4 步:接下来,通过将以下内容添加到您的grade.properties 文件来启用AndriodX :
org.gradle.jvmargs=-Xmx1536M
android.enableR8=true
android.useAndroidX=true
android.enableJetifier=true
第五步:现在需要在各自的dart文件中实现如下代码
Dart
import 'package:flutter/material.dart';
import 'package:flutter_gauge/flutter_gauge.dart';
class FlutterGaugePage extends StatefulWidget {
@override
_FlutterGaugePageState createState() => _FlutterGaugePageState();
}
class _FlutterGaugePageState extends State {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
title: Text("GeeksforGeeks"),
backgroundColor: Color(0xFF4CAF50),
automaticallyImplyLeading: false,
centerTitle: true,
),
body: SingleChildScrollView(
child: Column(
children: [
Row(
children: [
Expanded(
child: Center(
child: FlutterGauge(
handSize: 25,index: 40.0,
end: 100,number:
Number.endAndCenterAndStart,
circleColor: Color(0xFF47505F),
secondsMarker:
SecondsMarker.secondsAndMinute,
counterStyle: TextStyle(
color: Colors.black,fontSize: 20,)
),
)
),
Expanded(
child: FlutterGauge(
secondsMarker: SecondsMarker.none,
hand: Hand.short, number: Number.none,
index: 66.0,circleColor: Color(0xFF9DC1DC),
counterStyle: TextStyle(color: Colors.black,
fontSize: 25
),
counterAlign: CounterAlign.center,
isDecimal: false
)),
],
),
Row(
children: [
Expanded(
child: FlutterGauge(
handSize: 25,index: 70.0,end: 100,
number: Number.endAndCenterAndStart,
secondsMarker: SecondsMarker.secondsAndMinute
,hand: Hand.short,
circleColor: Color(0xFF59EA50),
counterStyle: TextStyle(color:
Colors.black,fontSize: 20,)
),
),
Expanded(
child: FlutterGauge(
handSize: 25,index: 100.0,end: 500,
number: Number.endAndStart,
secondsMarker: SecondsMarker.minutes,
isCircle: false,
counterStyle: TextStyle(color:
Colors.black,fontSize: 20,)
),
),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Expanded(
child: FlutterGauge(
index: 50,width:280,counterStyle : TextStyle
(color: Colors.black,fontSize: 22,),
secondsMarker: SecondsMarker.secondsAndMinute,
number: Number.all, numberInAndOut:
NumberInAndOut.outside,
),
),
],
),
);
}
}
Dart
FlutterGauge(
handSize: 25,index: 40.0,
end: 100,number:
Number.endAndCenterAndStart,
circleColor: Color(0xFF47505F),
secondsMarker:
SecondsMarker.secondsAndMinute,
counterStyle: TextStyle(
color: Colors.black,fontSize: 20,
)
),
Dart
FlutterGauge(
secondsMarker: SecondsMarker.none,
hand: Hand.short,
number: Number.none, index: 66.0,
circleColor: Color(0xFF9DC1DC),
counterStyle: TextStyle(
color: Colors.black, fontSize:25),
counterAlign: CounterAlign.center,
isDecimal: false
)),
Dart
FlutterGauge(
handSize: 25,index: 70.0,end: 100,
number: Number.endAndCenterAndStart,
secondsMarker: SecondsMarker.secondsAndMinute,
hand: Hand.short,
circleColor: Color(0xFF59EA50),
counterStyle: TextStyle(color: Colors.black,
fontSize: 20,
)
),
Dart
FlutterGauge(
handSize: 25, index: 100.0, end: 500,
number: Number.endAndStart,
secondsMarker: SecondsMarker.minutes,
isCircle: false,
counterStyle: textStyle(
color: Colors.black, fontSize: 20,
)
)
Dart
FlutterGauge(
index: 50,width:280,
counterStyle : TextStyle(color: Colors.black,
fontSize: 22,),
secondsMarker: SecondsMarker.secondsAndMinute,
number: Number.all,
numberInAndOut: NumberInAndOut.outside,
),
输出:
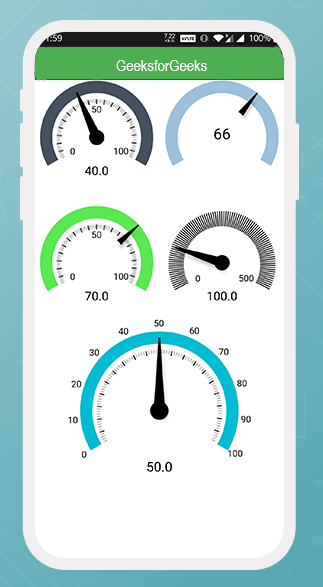
Flutter Gauge 演示
解释:
以下是对上述dart代码中实现的flutter计类型的解释。
Dart
FlutterGauge(
handSize: 25,index: 40.0,
end: 100,number:
Number.endAndCenterAndStart,
circleColor: Color(0xFF47505F),
secondsMarker:
SecondsMarker.secondsAndMinute,
counterStyle: TextStyle(
color: Colors.black,fontSize: 20,
)
),
输出:
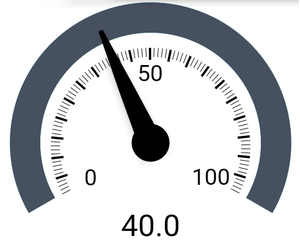
Flutter Gauge Type 1
在上面的flutter计中,我们将索引设置为 40。我们还将handSize设置为 25,并将数字设置为endAndCenterAndStart,从 0 开始,以 100 结束。这个flutter计中使用的secondsMarker是秒和分钟函数.我们还设置了圆圈颜色,但默认颜色为蓝色。
Dart
FlutterGauge(
secondsMarker: SecondsMarker.none,
hand: Hand.short,
number: Number.none, index: 66.0,
circleColor: Color(0xFF9DC1DC),
counterStyle: TextStyle(
color: Colors.black, fontSize:25),
counterAlign: CounterAlign.center,
isDecimal: false
)),
输出:
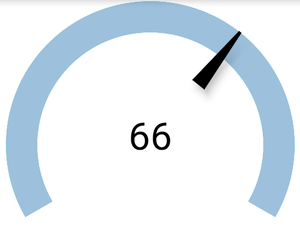
Flutter Gauge Type 2
在上面的flutter计中,我们将指数设置为 66。手设置为空头。数字和秒的标记设置为无。我们还设置了圆圈颜色,但默认颜色为蓝色。我们已将十进制值设置为 false 并将计数器对齐在中心。
Dart
FlutterGauge(
handSize: 25,index: 70.0,end: 100,
number: Number.endAndCenterAndStart,
secondsMarker: SecondsMarker.secondsAndMinute,
hand: Hand.short,
circleColor: Color(0xFF59EA50),
counterStyle: TextStyle(color: Colors.black,
fontSize: 20,
)
),
输出:
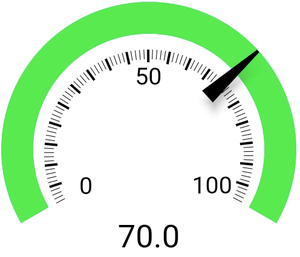
Flutter Gauge Type 3
在上面的flutter计中,我们将索引设置为 70。我们还将handSize设置为 25,并将数字设置为endAndCenterAndStart,从 0 开始,以 100 结束。这个flutter计中使用的secondsMarker是秒和分钟函数并且手被设置为短。我们还设置了圆圈颜色,但默认颜色为蓝色。
Dart
FlutterGauge(
handSize: 25, index: 100.0, end: 500,
number: Number.endAndStart,
secondsMarker: SecondsMarker.minutes,
isCircle: false,
counterStyle: textStyle(
color: Colors.black, fontSize: 20,
)
)
输出:
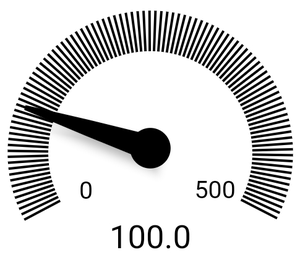
Flutter Gauge Type 4
在此以上flutter计,我们已经设置了索引为100。我们还设置handSize 25以及从0开始作为endandStart的数量和与500 secondsMarker结束本flutter规使用的微小的函数和手被设置为短。我们还将圆圈设置为假。
Dart
FlutterGauge(
index: 50,width:280,
counterStyle : TextStyle(color: Colors.black,
fontSize: 22,),
secondsMarker: SecondsMarker.secondsAndMinute,
number: Number.all,
numberInAndOut: NumberInAndOut.outside,
),
输出:
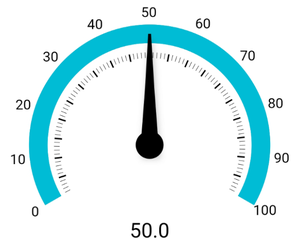
Flutter Gauge Type 5
在上面的flutter计中,我们将索引设置为 50。我们还将宽度设置为 280,将数字设置为全部。这个flutter计中使用的secondsMarker是一个分钟函数,数字在外面显示InAndOut 。我们还设置了圆圈颜色,但默认颜色为蓝色。