给定N ‘,非平行线的数量。任务是找到这些线可以划分平面的最大区域数。
例子:
Input : N = 3
Output : 7
Input : N = 2
Output : 4
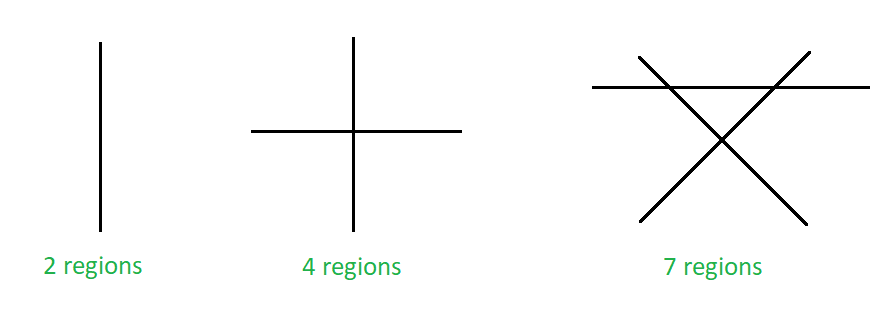
由于非平行线形成的平面上的最大区域数
方法:上图显示了一条线可以划分平面的最大区域数。一条线可以把一个平面分成两个区域,两条不平行的线可以把一个平面分成4个区域,三条不平行的线可以分成7个区域,以此类推。当将第n 行添加到 (n-1) 行的集群中时,形成的额外区域的最大数量等于 n。
现在解决递归如下:
L(2) – L(1) = 2 … (i)
L(3) – L(2) = 3 … (ii)
L(4) – L(3) = 4 … (iii)
. . .
. . .
L(n) – L(n-1) = n ; … (n)
Adding all the above equation we get,
L(n) – L(1) = 2 + 3 + 4 + 5 + 6 + 7 + …… + n ;
L(n) = L(1) + 2 + 3 + 4 + 5 + 6 + 7 + …… + n ;
L(n) = 2 + 2 + 3 + 4 + 5 + 6 + 7 + …… + n ;
L(n) = 1 + 2 + 3 + 4 + 5 + 6 + 7 + …… + n + 1 ;
L(n) = n ( n + 1 ) / 2 + 1 ;
N条非平行线可划分平面的区域数等于N*(N+1)/2+1 。
下面是上述方法的实现:
C++
// C++ program to implement the above problem
#include
using namespace std;
// Function to find the maximum
// number of regions on a plane
void maxRegions(int n)
{
int num;
num = n * (n + 1) / 2 + 1;
// print the maximum number of regions
cout << num;
}
// Driver code
int main()
{
int n = 10;
maxRegions(n);
return 0;
}
Java
// Java program to implement the above problem
class GFG
{
// Function to find the maximum
// number of regions on a plane
static void maxRegions(int n)
{
int num;
num = n * (n + 1) / 2 + 1;
// print the maximum number of regions
System.out.println(num);;
}
// Driver code
public static void main(String[] args)
{
int n = 10;
maxRegions(n);
}
}
// This code is contributed by 29AjayKumar
Python3
# Python3 program to implement
# the above problem
# Function to find the maximum
# number of regions on a plane
def maxRegions(n):
num = n * (n + 1) // 2 + 1
# print the maximum number
# of regions
print(num)
# Driver code
n = 10
maxRegions(n)
# This code is contributed
# by Mohit Kumar
C#
// C# program to implement the above problem
using System;
class GFG
{
// Function to find the maximum
// number of regions on a plane
static void maxRegions(int n)
{
int num;
num = n * (n + 1) / 2 + 1;
// print the maximum number of regions
Console.WriteLine(num);
}
// Driver code
public static void Main(String[] args)
{
int n = 10;
maxRegions(n);
}
}
// This code is contributed by 29AjayKumar
Javascript
56
时间复杂度: O(1)